How to Manage ACL Permissions Using PowerShell
- NTFS Permission Types in PowerShell
-
Use the
Get-Acl
Command to Get ACL for Folders and Files in PowerShell -
Use the
Set-Acl
Command to Set ACL for Files and Folders in PowerShell -
Use the
-RemoveAccessRule
Parameter to Remove User Permissions in PowerShell - Disable or Enable Permission Inheritance in PowerShell
-
Use the
SetOwner
Method to Change File and Folder Ownership in PowerShell
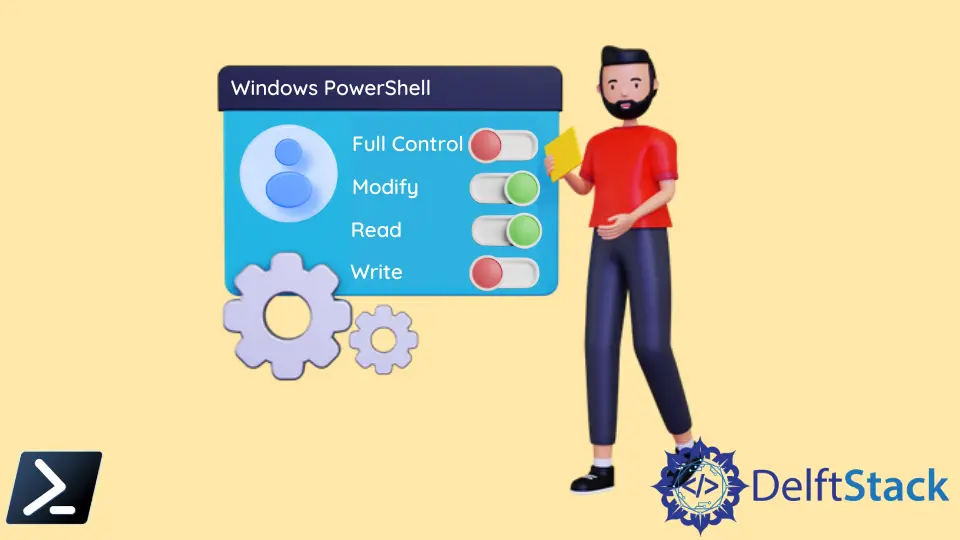
System administrators configure NTFS access control lists ACLs
by adding access control entries ACEs
on NTFS files servers to implement a least-privilege model.
This article will learn the different permission types and how to query, modify, and remove ACL on files and folders using PowerShell.
NTFS Permission Types in PowerShell
There are both advanced and basic NTFS permissions. For example, you can set each permission to Deny
or Allow
.
Full Control
: Users with this permission can modify, add, move and delete files and directories, as well as their associated properties. In addition, the users with this permission can change permissions settings for all subdirectories and files.Modify
: Users with this permission can view and modify files and file properties, including adding and deleting files to a directory or file properties to a file.Read & Execute
: Users with this permission can run executable files, including scripts.Read
: Users can view files, file properties, and directories with this permission.Write
: Users with this permission can write to a file and add files to directories.
Here is the list of advanced permissions:
Traverse Folder
orExecute File
: Users with this advanced permission can navigate through folders to reach other folders or files, even if they have no permissions for these files or folders. Users with this advanced permission can also run executable files. TheTraverse Folder
permission takes effect when the user or group doesn’t have theBypass Traverse Checking
right in the Group Policy snap-in.List Folder
orRead Data
: Users with this advanced permission can view a list of files and subfolders within the folder and the content of the files.Read Attributes
: Users with this advanced permission can view the attributes of a folder or file, such as whether it is hidden or read-only.Write Attributes
: Users with this advanced permission can change the attributes of a file or folder.Read Extended Attributes
: Users with this advanced permission can view the extended attributes of a folder or file, such as permissions and creation and modification times.Write Extended Attributes
: Users with this advanced permission can change the extended attributes of a folder or file.Create Files
orWrite Data
: TheCreate Files
permission will allow users to create files within the folder with this advanced permission.This permission applies to folders only.
TheWrite Data
permission will enable users with this advanced permission to change the file and overwrite existing content. This permission only applies to files.Create Folders
orAppend Data
: TheCreate Folders
permission allows users to create folders within a folder with this advanced permission.This permission applies to folders only.
TheAppend Data
permission will enable users with this advanced permission to make changes to the end of the file, but they cannot change, overwrite, or delete existing data. This permission only applies to files.Delete
: Users with this advanced permission can delete the folder or file. If users do not have theDelete
permission on a folder or file, they can still delete an object if they have theDelete Subfolders And Files
permission on the parent folder.Read Permissions
: Users with this advanced permission can read the permissions of a folder or file, such asFull Control
,Read
, andWrite
.Change Permissions
: Users with this advanced permission can change the permissions of a file or folder.Take Ownership
: Users with this advanced permission can take ownership of the file or folder. The file or folder owner can always change its permissions, regardless of any existing permissions that protect the file or folder.Synchronize
: Users with this advanced permission can use the object for synchronization. This permission will enable a thread to wait until the object is in the signaled state. This permission is not presented in ACL Editor.
We can find all information on these user permissions by running the following PowerShell script below:
[System.Enum]::GetNames([System.Security.AccessControl.FileSystemRights])
The NTFS permissions can be either explicit or inherited. Explicit permissions are configured individually, while inherited permissions are inherited from the parent folder.
The hierarchy for permissions is as follows:
- Explicit Deny
- Explicit Allow
- Inherited Deny
- Inherited Allow
Use the Get-Acl
Command to Get ACL for Folders and Files in PowerShell
The first PowerShell command used to manage file and folder permissions is Get-Acl
; it lists all object permissions.
Get-Acl \\fs1\shared\hr | fl
A user must own both the target and source folders to copy permissions.
Get-Acl \\fs1\shared\hr | Set-Acl \\fs1\shared\hr
Use the Set-Acl
Command to Set ACL for Files and Folders in PowerShell
The PowerShell Set-Acl
command is used to change the security descriptor of a specified item, such as a file, folder, or registry key; in other words, it is used to modify file or folder permissions.
$acl = Get-Acl \\fs1\shared\hr
$AccessRule = New-Object System.Security.AccessControl.FileSystemAccessRule("ENTERPRISE\User01", "FullControl", "Allow")
$acl.SetAccessRule($AccessRule)
$acl | Set-Acl \\fs1\shared\hr
Use the -RemoveAccessRule
Parameter to Remove User Permissions in PowerShell
$acl = Get-Acl \\fs1\shared\hr
$AccessRule = New-Object System.Security.AccessControl.FileSystemAccessRule("ENTERPRISE\User01", "FullControl", "Allow")
$acl.RemoveAccessRule($AccessRule)
$acl | Set-Acl \\fs1\shared\hr
Disable or Enable Permission Inheritance in PowerShell
To manage an inheritance, we can use the SetAccessRuleProtection
method. The method has two parameters:
- The first parameter is responsible for blocking inheritance from the parent folder. It returns Boolean states:
$true
and$false
. - The second parameter is used whether the current inherited permissions are removed or retained. It also returns Boolean states:
$true
and$false
.
$acl = Get-Acl \\fs1\shared\hr
$acl.SetAccessRuleProtection($true, $false)
$acl | Set-Acl \\fs1\shared\hr
Let’s revert this change and enable inheritance for the folder again:
$acl = Get-Acl \\fs1\shared\hr
$acl.SetAccessRuleProtection($false, $true)
$acl | Set-Acl \\fs1\shared\hr
Use the SetOwner
Method to Change File and Folder Ownership in PowerShell
If we want to set an owner for a folder, you need to run the SetOwner
method.
$acl = Get-Acl \\fs1\shared\hr
$object = New-Object System.Security.Principal.Ntaccount("ENTERPRISE\User01")
$acl.SetOwner($object)
$acl | Set-Acl \\fs1\shared\hr
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn