How to Invoke WebRequest in PowerShell 2.0
-
PowerShell Static Member Operator
::
-
Create a Web Request With the
WebRequest
Class to Invoke WebRequest in PowerShell 2.0
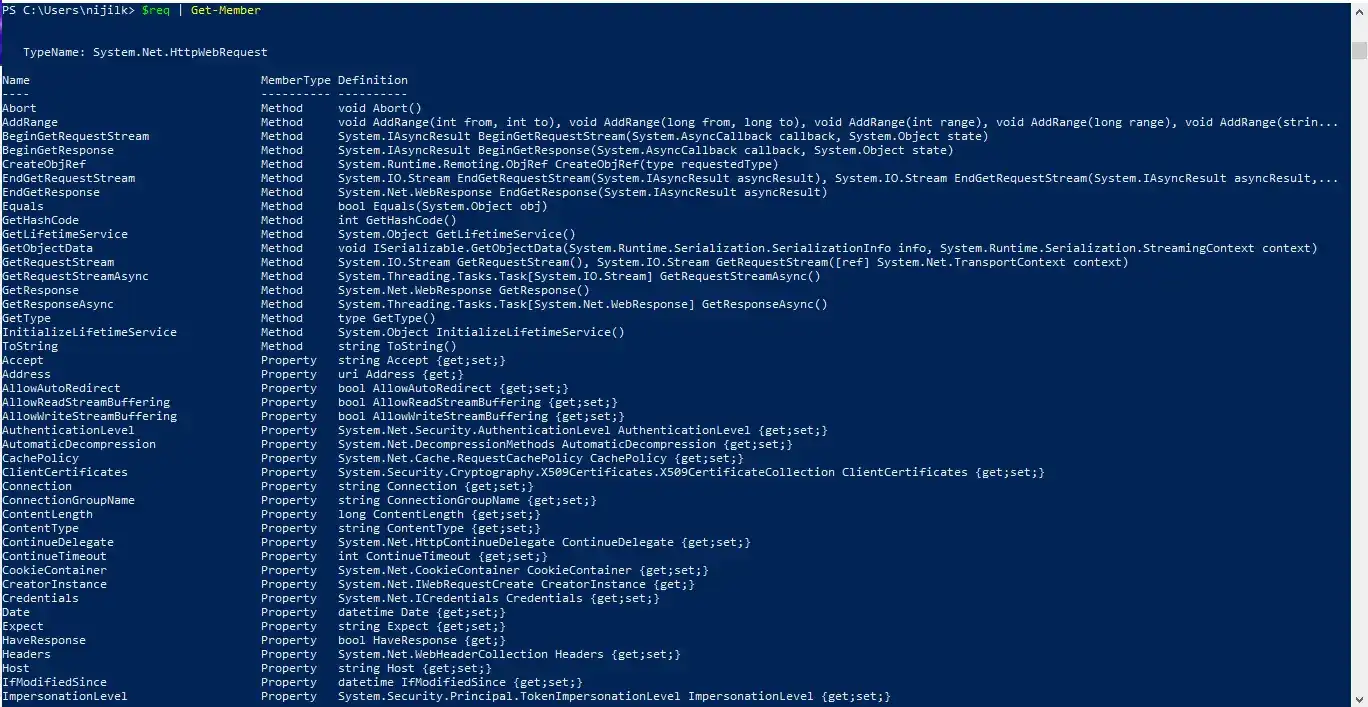
From PowerShell version 3.0, the Invoke-WebRequest
cmdlet has been introduced to send HTTP or HTTPS web requests and get the response back.
Before that, in PowerShell version 2.0, the .Net
base class library was used to send web requests. Both the WebRequest
and HttpWebRequest
classes can be used for this purpose.
The strength of PowerShell is that it enables you to access in-built .Net
classes and methods without a hassle.
PowerShell Static Member Operator ::
PowerShell’s most significant advantage is that it has given the capability to access .Net
classes within PowerShell scripts. The static member operator calls the static methods and properties inside a .Net
library class.
Let’s say we want to access the Now
property of the DateTime
class. It would ideally return the current system date and time.
We can call this property using PowerShell, as follows.
[datetime]::Now
Output:
As expected, the current date and time have been printed.
Create a Web Request With the WebRequest
Class to Invoke WebRequest in PowerShell 2.0
The WebRequest
class is a .NET
based abstract implemented to invoke a request against a given URI (Uniform Resource Identifier). It resides in the System.Net
package of the .NET
framework.
The following is the syntax to invoke a webrequest from PowerShell.
[System.Net.WebRequest]::Create(uri)
Here, uri
is the URI of the website.
Let’s make a simple GET
request to the https://google.com
.
$req = [System.Net.WebRequest]::Create('https://google.com')
This would create a PowerShell $req
object that can be manipulated further. We can list the available properties and methods for the $req
object.
$req | Get-Member
Output:
We can use the GetResponse()
method to access the response object out of this request.
$resp = $req.GetResponse()
Write the $resp
object to the PowerShell console window.
The same procedure can be followed with some additional information when you need to process a POST
request. In that case, you need to use the request
method as POST
.
In addition, the request content type and the body must be constructed and passed as follows.
$request = [System.Net.WebRequest]::Create('https://abc.com');
$request.Method = "POST";
$request.ContentType = "application/x-www-form-urlencoded";
$bytes = [System.Text.Encoding]::ASCII.GetBytes("name=tony&age=55");
$request.ContentLength = $bytes.Length;
$requestStream = $request.GetRequestStream();
$requestStream.Write( $bytes, 0, $bytes.Length );
$requestStream.Close();
$request.GetResponse();
You can use the above implementation to proceed with a POST
request using the WebRequest
class.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.