How to Initialize an Array of Custom Objects in PowerShell
- Using Arrays in Windows PowerShell
- Accessing an Array in Windows PowerShell
- Changing Items in an Array Using Windows PowerShell
- Iterating an Array in Windows PowerShell
- Creating Objects in an Array Using Windows PowerShell
- Accessing Objects in an Array Using Windows PowerShell
- Updating Objects in an Array Using Windows PowerShell
- Accessing Properties in an Array Using Windows PowerShell
- Creating an ArrayList in Windows PowerShell
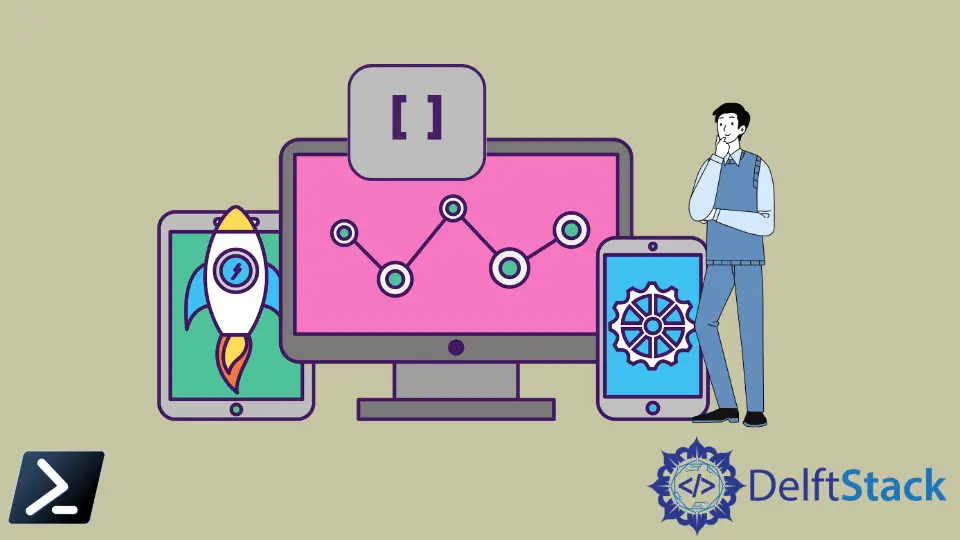
Arrays are a fundamental feature of Windows PowerShell. These make it possible to ingest, manipulate and output accurate data structures (and not just raw strings).
This capability makes PowerShell different and more practical than other scripting languages.
This article will explain what arrays are, how to create arrays in Windows PowerShell, and then use essential functions to manipulate them.
Using Arrays in Windows PowerShell
Arrays in Windows PowerShell can contain one or more items. An array can be a string, an integer, an object, or another array.
Each item has an index that starts at 0 and iterates per item.
The basic syntax of creating an array is @()
.
$data = @()
We can put values inside the parentheses after the @
:
$data = @('Zero', 'One', 'Two', 'Three')
To display items in the array, we can call the named variable:
$data
Output:
Zero
One
Two
Three
Accessing an Array in Windows PowerShell
Now that we have an array, we will want to access items. There are several ways to do this.
The first method is to use the index of the items in the array. As we have mentioned, indexes start at 0
.
So we will need to tell Windows PowerShell to look at the item whose assigned index is zero to retrieve the first item in our array.
$data[0]
This syntax will return the string zero
because that was the first string we placed in our array. We can also extend this snippet to return multiple items from the same array by putting more indexes in the same command.
$data[0, 2, 3]
Output:
Zero
Two
Three
Changing Items in an Array Using Windows PowerShell
We can use the same method to update the items in an array. For instance, to update the item whose assigned index is 2, we can run:
$data[2] = 'second'
This method gives us direct access to the items inside an array.
Iterating an Array in Windows PowerShell
One of the most powerful features of Windows PowerShell is performing the same action on all the items in an array. The most basic method is using a Pipeline character (|
).
For example, to add a description to each item in our array, we can use this command:
$data | ForEach-Object { "Item: [$PSItem]" }
This command tells the PowerShell scripting environment to take the items in the $data
array one at a time.
Creating Objects in an Array Using Windows PowerShell
We can create an array full of custom objects using the same method with strings, using the array (@()
) function. For example, to make a test list of employees, we can use:
$data = @(
[pscustomobject]@{FirstName = 'Kevin'; LastName = 'Marquette' }
[pscustomobject]@{FirstName = 'John'; LastName = 'Doe' }
)
Most PowerShell native commands will return an array full of objects of this type when you assign them a variable to work with.
Accessing Objects in an Array Using Windows PowerShell
The process we ran through before to access individual pieces of data can equally be used with arrays that contain objects.
$data[0]
Output:
FirstName LastName
----- ----
John Hampton
Alternatively, we can get the properties of individual objects by specifying which property we would like to call within the same command.
$data[0].FirstName
Output:
John
Updating Objects in an Array Using Windows PowerShell
The exact syntax allows us to update individual properties within objects held in arrays.
$data[0].FirstName = 'Jay'
Accessing Properties in an Array Using Windows PowerShell
In most programming and scripting languages, we would have to use an iterative method to access all the properties in various objects. We can do that in Windows PowerShell as well.
$data | ForEach-Object { $_.LastName }
This method will return a list of all of the LastName
properties in our array, but it is computationally expensive and challenging to write every time you want to see this data. So instead, PowerShell has a shortcut.
$data.LastName
And you will see the same list. PowerShell is taking each object in turn, just as before, but it hides this complexity from us.
Creating an ArrayList in Windows PowerShell
Adding items to arrays can be challenging. However, ArrayList handles this more elegantly, a different type of collection.
However, we will have to use the .NET framework to use this type of collection. To create an ArrayList, and add items to it, run the following:
$myarray = [System.Collections.ArrayList]::new()
[void]$myArray.Add('Value')
Here, we can call the default .NET constructor to create a new ArrayList and then use the Add
operator to add items to it.
The [void]
data type is there because sometimes these commands throw out strange outputs that can mess with the code.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn