How to Increase and Decrease Variable in PowerShell
- Understanding Increment and Decrement Operations in PowerShell
- Pre-Increment/Decrement and Post-Increment/Decrement in PowerShell
-
Pre-Increment/Decrement and Post-Increment/Decrement With
for
Loops in PowerShell -
Pre-Increment/Decrement and Post-Increment/Decrement With
do-while
Loops in PowerShell - Use Assignment Operators to Increment/Decrement in PowerShell
- Use Arithmetic Operators to Increment/Decrement in PowerShell
- Conclusion
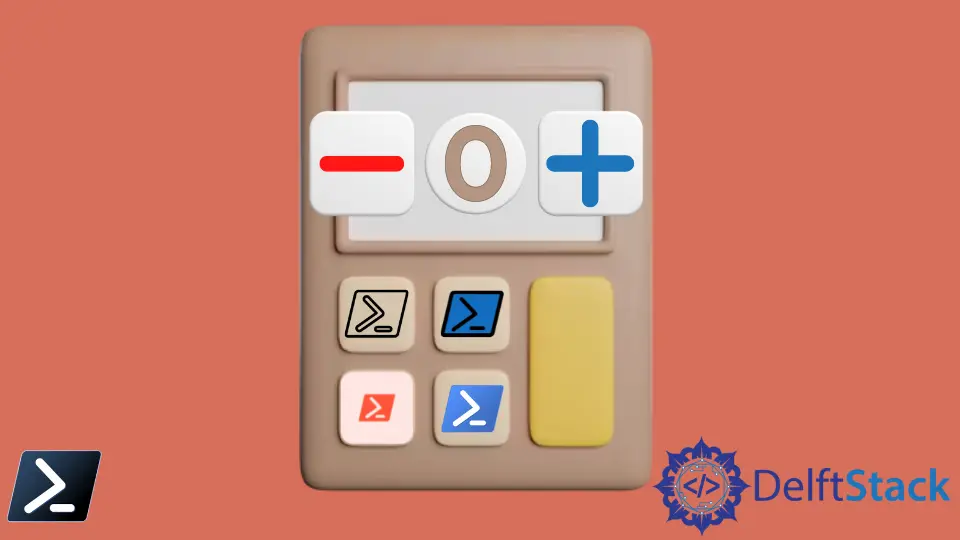
In PowerShell scripting, the capability to increment and decrement variables is fundamental for various scenarios, from simple arithmetic operations to more complex control flow mechanisms. This comprehensive guide explores multiple methods for incrementing and decrementing variables in PowerShell, providing detailed examples and explanations for each technique.
Understanding Increment and Decrement Operations in PowerShell
Incrementing involves increasing the value of a variable by a specific amount, typically by one. Conversely, decrementing decreases the variable’s value, often by one as well.
These operations are essential for iterative tasks, loop control, and numeric manipulations within PowerShell scripts.
Common Syntax:
- Increment:
$variable++
or$variable += 1
- Decrement:
$variable--
or$variable -= 1
Like any programming language, the increment operator signified by a double plus sign (++
) will increase the variable’s value by 1
. In contrast, the decrement operator represented by a double minus sign (--
) will decrease by 1
.
Incrementing and decrementing operators are easier to write when compared to the traditional way of writing it. Technically, $num++
is more seamless when compared to $num = $num + 1
.
Increment Example
$counter = 5
$counter++
Write-Host "Incremented counter: $counter"
Output:
Incremented counter: 6
Explanation:
$counter = 5
: Initializes the variable$counter
.$counter++
: Utilizes the increment operator to increase the value of$counter
by one.Write-Host "Incremented counter: $counter"
: Displays the incremented value of$counter
.
Decrement Example
$counter = 10
$counter--
Write-Host "Decremented counter: $counter"
Output:
Decremented counter: 9
Explanation:
$counter = 10
: Initializes the variable$counter
.$counter--
: Utilizes the decrement operator to decrease the value of$counter
by one.Write-Host "Decremented counter: $counter"
: Displays the decremented value of$counter
.
Incrementing and decrementing are often used in loops as exit conditions. However, we must adequately understand when to increment/decrement in a loop.
Pre-Increment/Decrement and Post-Increment/Decrement in PowerShell
The pre-increment and pre-decrement operators affect the value of a variable before its utilization in an expression. On the other hand, the post-increment and post-decrement operators impact the value of a variable after its utilization in an expression.
We can write the increment/decrement operators before the variable in PowerShell.
Syntax:
++$number
--$number
By definition, the pre-increment/decrement operators increment/decrement their operand by one, and the value of the expression returns the resulting incremented (or decremented) value.
Loop Iterations
$i = 0
while ($i -lt 5) {
Write-Host "Index: $i"
++$i # Pre-increment for clarity
}
Output:
Index: 0
Index: 1
Index: 2
Index: 3
Index: 4
Using pre-increment in loops can enhance readability by indicating the incrementation occurs before using the variable’s updated value within the loop’s block.
Counter Operations
$counter = 10
$counter-- # Post-decrement for post-usage decrement
Write-Host "Current Counter Value: $counter"
Output:
Current Counter Value: 9
In scenarios where the variable’s updated value isn’t immediately utilized, post-decrement can be used for clarity in intent.
Differences Between Pre- And Post-Increment/Decrement
The crucial difference lies in when the increment or decrement operation occurs concerning the variable’s utilization within an expression. Pre-increment and pre-decrement adjust the variable’s value before its utilization, affecting the entire expression’s evaluation.
Conversely, post-increment and post-decrement alter the variable after its value is utilized in an expression, impacting only subsequent evaluations.
Pre-Increment/Decrement and Post-Increment/Decrement With for
Loops in PowerShell
In PowerShell, for
loops are crucial for iterating through collections or executing a block of code a specific number of times. Understanding how pre-increment and post-increment/decrement operators function within these loops is essential for controlling loop behavior and variable manipulation.
Syntax of a for
Loop in PowerShell
A for
loop in PowerShell consists of three components:
for ($initialization; $condition; $increment / decrement) {
# Code block to execute
}
$initialization
: The initial setup, where variables are initialized.$condition
: The condition to evaluate for each iteration. When false, the loop terminates.$increment/decrement
: The operation that modifies the loop variable.
Pre-Increment in for
Loops (++$variable
)
The pre-increment operator (++$variable
) adjusts the variable’s value before its utilization in the loop’s block. Here’s an example:
for ($i = 0; $i -lt 5; ++$i) {
Write-Host "Pre-increment: $i"
}
Output:
Pre-increment: 0
Pre-increment: 1
Pre-increment: 2
Pre-increment: 3
Pre-increment: 4
In this scenario, $i
is incremented by 1
before its utilization within the loop’s block. This loop prints values from 0
to 4
.
Post-Increment in for
Loops ($variable++
)
The post-increment operator ($variable++
) modifies the variable’s value after its utilization in the loop’s block. Here’s an example:
for ($j = 0; $j -lt 5; $j++) {
Write-Host "Post-increment: $j"
}
Output:
Post-increment: 0
Post-increment: 1
Post-increment: 2
Post-increment: 3
Post-increment: 4
Here, $j
is incremented by 1
after its utilization within the loop’s block. This loop also prints values from 0
to 4
.
Pre-Increment/Decrement and Post-Increment/Decrement With do-while
Loops in PowerShell
In PowerShell, do-while
loops are crucial for executing a block of code at least once and repeating it based on a condition. Understanding how pre-increment and post-increment/decrement operators function within these loops is essential for controlling loop behavior and variable manipulation.
Syntax of a do-while
Loop in PowerShell
A do-while
loop executes a block of code at least once and then continues execution based on a specified condition:
do {
# Code block to execute
} while ($condition)
- The code block within the
do { }
section executes at least once before evaluating the$condition
. - If
$condition
evaluates to true, the loop continues; otherwise, it terminates.
Post-Increment in do-while
Loops (++$variable
)
The do-while
loop will execute the loop first before checking the exit condition. For example, try running the script below that numbers 1
through 5
using the do-while
loop.
Example Script:
$num = 1
Do {
$num
} While ($num++ -le 5)
Output:
1
2
3
4
5
6
Since we are post-incrementing, the loop will first check the condition, and since $num
is still equal to 5
, the loop will allow the script to run another pass. After the condition has been checked, $num
will increment to 6
, thus the returned output.
Pre-Increment in do-while
Loops (++$variable
)
We want to pre-increment the $num
value in the following example. In this way, when the condition is evaluated, the $num
value will be less than or equal to 5
.
All we need to do is move where ++
appears to pre-increment to stop the loop when the $num
value is equal to 5
.
Example Script:
$num = 1
Do {
$num
} While (++$num -le 5)
Output:
1
2
3
4
5
If we use the While
loop instead, post-incrementing/decrementing will work fine since the condition is checked first before running the loop.
The point is that incrementing and decrementing are as important as where we place these operators in programming logic, so we should use them carefully when scripting.
Use Assignment Operators to Increment/Decrement in PowerShell
In PowerShell, assignment operators provide convenient ways to modify variable values by performing arithmetic operations like incrementing and decrementing. Understanding these operators is essential for efficient variable manipulation within scripts and functions.
Increment Assignment Operator (+=
)
The +=
operator allows for efficient incrementing of a variable by a specific value. Here’s an example:
$counter = 7
$counter += 1
Write-Host "Incremented counter: $counter"
Output:
Incremented counter: 8
Explanation:
$counter = 7
: Initializes the variable$counter
.$counter += 1
: Increases the value of$counter
by one using the+=
assignment operator.Write-Host "Incremented counter: $counter"
: Displays the incremented value of$counter
.
Decrement Assignment Operator (-=
)
Conversely, the -=
operator facilitates efficient decrementing of a variable by a specified value:
$counter = 12
$counter -= 1
Write-Host "Decremented counter: $counter"
Output:
Decremented counter: 11
Explanation:
$counter = 12
: Initializes the variable$counter
.$counter -= 1
: Decreases the value of$counter
by one using the-=
assignment operator.Write-Host "Decremented counter: $counter"
: Displays the decremented value of$counter
.
Use Arithmetic Operators to Increment/Decrement in PowerShell
In PowerShell, arithmetic operators play a crucial role in altering numerical values by incrementing or decrementing them. Understanding these operators is essential for manipulating variables, counters, and array indices, enhancing code flexibility and functionality.
Addition Operator (+
) for Incrementing
The addition operator (+
) increases the value of a variable by a specified amount:
$counter = 9
$counter = $counter + 1
Write-Host "Incremented counter: $counter"
Output:
Incremented counter: 10
Explanation:
$counter = 9
: Initializes the variable$counter
.$counter = $counter + 1
: Adds one to the current value of$counter
and assigns it back to$counter
.Write-Host "Incremented counter: $counter"
: Displays the incremented value of$counter
.
Subtraction Operator (-
) for Decrementing
The subtraction operator (-
) decreases the value of a variable by a specified amount:
$counter = 15
$counter = $counter - 1
Write-Host "Decremented counter: $counter"
Output:
Decremented counter: 14
Explanation:
$counter = 15
: Initializes the variable$counter
.$counter = $counter - 1
: Subtracts one from the current value of$counter
and assigns it back to$counter
.Write-Host "Decremented counter: $counter"
: Displays the decremented value of$counter
.
Conclusion
In PowerShell scripting, the ability to increment and decrement variables is fundamental across various scenarios, from basic arithmetic operations to intricate control flow mechanisms. This comprehensive guide meticulously explores multiple methods for incrementing and decrementing variables in PowerShell, offering detailed examples and explanations for each technique.
From the fundamental syntax of increment and decrement operations to in-depth insights into pre-increment/decrement, post-increment/decrement, and their effective utilization within loops like for
and do-while
, this guide covers a spectrum of approaches. Moreover, it delves into using assignment and arithmetic operators, emphasizing their significance in manipulating variable values efficiently.
Understanding when and how to apply these operations is crucial. Whether in iterative tasks, loop control, numeric manipulations, or script optimization, the comprehension of these techniques ensures precise and efficient PowerShell scripting.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn