How to Handle Errors Using Try-Catch Block in PowerShell
-
the
$ErrorActionPreference
Variable in PowerShell -
the
ErrorAction
Common Parameter in PowerShell -
Use PowerShell
try-catch
Block
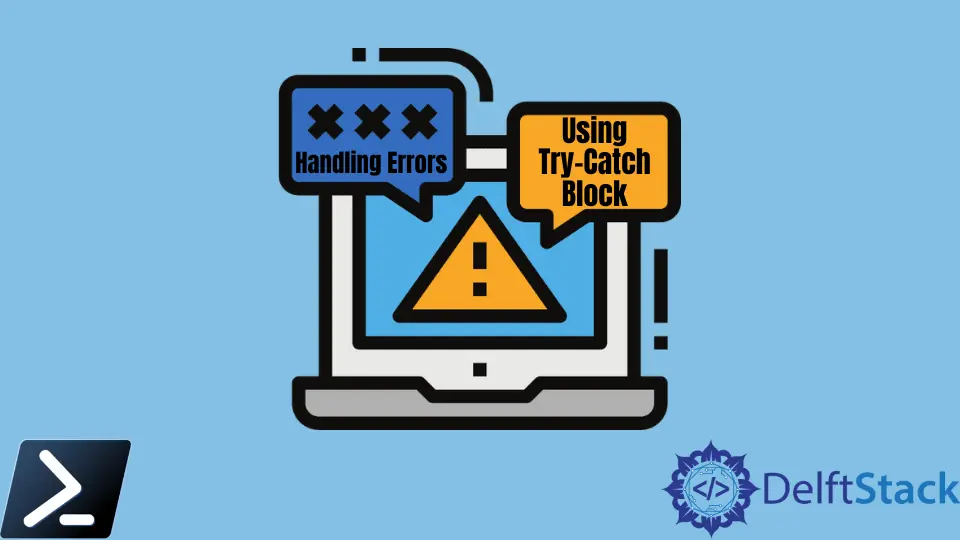
Code errors are frequently challenging to read, making establishing what and where the script went wrong nearly impossible. PowerShell has some options for improving error handling.
This article will discuss Error Action Preferences in PowerShell and how we can intercept them to perform error handling using the PowerShell Try-Catch
blocks (and Finally
blocks).
the $ErrorActionPreference
Variable in PowerShell
Preference variables are a notion in PowerShell, and these variables are used to alter PowerShell’s behavior in various ways. The $ErrorActionPreference
variable, is one of these variables.
The variable $ErrorActionPreference
controls how PowerShell handles non-terminating errors. The $ErrorActionPreference
value is set to "Continue"
by default.
When the $ErrorActionPreference
variable is set to "Stop"
, PowerShell treats all errors as terminating errors. Use the snippet below to change the $ErrorActionPreference
value.
$ErrorActionPreference = "Stop"
the ErrorAction
Common Parameter in PowerShell
The ErrorAction
parameter applies to any command that supports common parameters if the $ErrorActionPreference
value is applied to the PowerShell session. The values for the ErrorAction
argument are the same as for the $ErrorActionPreference
variable.
The value of the ErrorAction
parameter takes precedence over the value of the $ErrorActionPreference
parameter.
Example Code:
$ErrorActionPreference = "Continue"
$files = Get-Content .\files.txt
foreach ($file in $files) {
Write-Output "Reading file $file"
# Use the -ErrorAction common parameter
Get-Content $file -ErrorAction STOP
}
After running the modified code, we will see that even though the $ErrorActionPreference
is set to "Continue"
, the script is still terminated once it encounters an error. The script ended because the PowerShell ErrorAction
parameter value in Get-Content
was set to "Stop"
.
Use PowerShell try-catch
Block
Now, it’s time we learn about the PowerShell Try-Catch-Finally
blocks. PowerShell’s 'Try-Capture'
(and optional 'Finally'
) blocks let you wrap a block around a piece of code and catch any problems.
Example Code:
try {
<statement list>
}
catch [[<error type>][',' <error type>]*] {
<statement list>
}
finally {
<statement list>
}
The code we want PowerShell to Try
and monitor for faults is in the Try
block. If an error occurs when running the code in the Try
block, the error is saved in the $Error
variable and forwarded to the Catch
block.
When the Try
block returns an error, the Catch
block provides the steps to take. A Try
statement can contain several Catch
blocks.
That code is found at the end of the Try
statement in the Finally
block. Whether or not the script counted an error, this block executes.
A simple Try
statement has two blocks: a Try
and a Catch
. Finally
is an optional block.
The Capture
option should be empty to catch a non-specific exception. The script in the $ErrorActionPreference
Variable part of the example code below is adjusted to use the Try Catch
blocks.
The foreach
statement is now included within the Try
block below. A 'Catch'
block contains the code to display the string "An Error Occurred"
if an error occurs.
Lastly, the Finally
block code clears the $Error
variable.
Example Code:
$files = Get-Content .\files.txt
try {
foreach ($file in $files) {
Write-Output "Reading file $file"
Get-Content $file -ErrorAction STOP
}
}
catch {
Write-Host "An Error Occured" -ForegroundColor RED
}
finally {
$Error.Clear()
}
Output:
An Error Occured
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn