How to Get the MD5 Checksum in PowerShell
- Using the MD5 Hashing Algorithm in PowerShell
-
Using the
[System.Security.Cryptography.MD5]
Class in PowerShell -
Using the
MD5CryptoServiceProvider
Class in PowerShell - Conclusion
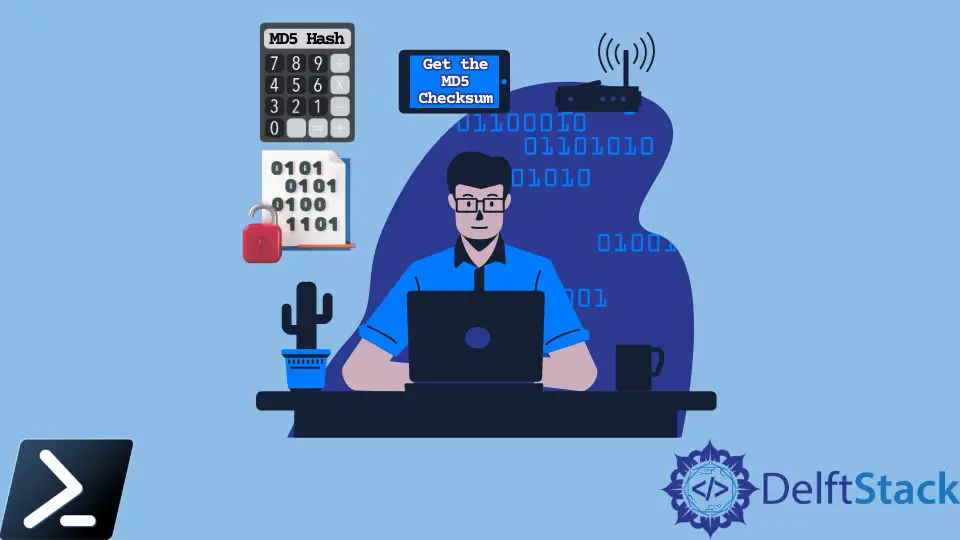
When it comes to ensuring data integrity and security in PowerShell, the MD5 hashing algorithm remains a valuable tool despite its diminishing relevance in security contexts. Although not recommended for cryptographic purposes due to vulnerabilities, MD5 remains useful for verifying file transfers and detecting tampering.
Leveraging PowerShell’s capabilities, we explore various methods to compute MD5 checksums, providing insights into both fundamental and advanced approaches.
Using the MD5 Hashing Algorithm in PowerShell
Even if MD5 is not recommended for security, it’s still an excellent solution to check if a file transfer has been tampered with or successful.
First, get the MD5 fingerprint of the file before and after the transfer.
If it results in the same value, the file transfer has not been tampered with. If not, it’s corrupted.
To do this, we will use the Get-FileHash
cmdlet. The Get-FileHash
cmdlet displays the hash value of a file.
It uses the SHA256
algorithm by default, but we can add an extra parameter to use MD5
.
Get-FileHash [-Path] <file> [[-Algorithm] <algo>] [Options]
-Algorithm
: Specifies the hash algorithm to use. In this case, we useMD5
.-Path
: Specifies the path to the file for which you want to compute the hash value.
We can also use it with a stream instead of a file path.
Get-FileHash [-InputStream] <stream> [[-Algorithm] <algo>] [Options]
-
[-InputStream] <stream>
: Specifies the input stream for hash calculation, defaulting to the file specified by-Path
. -
[[-Algorithm] <algo>]
: Specifies the hash algorithm (e.g.,MD5
,SHA1
) to use. -
[Options]
: Additional settings like-Path
,-LiteralPath
,-Force
, etc., for customization and error handling.
Here is an example:
Get-FileHash -Path "path\example.txt" -Algorithm MD5
In this code, we utilize the Get-FileHash
cmdlet to compute the MD5 checksum of the file located at "C:\Path\To\File.txt"
. The cmdlet automatically calculates the MD5 hash value for the specified file.
Output:
The -Path
argument is not mandatory, so we don’t need to use it. Instead, we give the file path and add the algorithm parameter to use MD5
instead of SHA256
.
Using PowerShell in a script, we can create a variable with the result and get the hash value with the hash property like $variable.Hash
to ensure it’s the same value as the original file.
Hashing Strings in PowerShell
Unfortunately, there is no direct function or native commands to generate a hash from a string in PowerShell.
However, it’s possible to use Get-FileHash
with a stream parameter, so it’s a solution to compute the hash of a string.
Let’s start directly with the snippet below:
$stringAsStream = [System.IO.MemoryStream]::new()
$writer = [System.IO.StreamWriter]::new($stringAsStream)
$writer.write("MD5Online")
$writer.Flush()
$stringAsStream.Position = 0
Get-FileHash -InputStream $stringAsStream -Algorithm MD5
In this code snippet, we create a MemoryStream
object $stringAsStream
and initialize a StreamWriter $writer
to write the string MD5Online
into the stream. We then flush the writer to ensure data is written to the stream.
After setting the position of the stream back to the beginning, we utilize the Get-FileHash
cmdlet with the -InputStream
parameter set to $stringAsStream
and the -Algorithm
parameter set to MD5
. This command calculates the MD5
checksum of the provided string.
Output:
Compared to the first section of this article, the only change is that we used -InputStream
instead of -Path
.
As you can see on the output, we get the MD5
hash of our string as a result.
Using the [System.Security.Cryptography.MD5]
Class in PowerShell
The [System.Security.Cryptography.MD5]
class provides functionality to compute the MD5 hash value of data. This class is part of the .NET Framework’s Cryptography namespace and offers a robust and efficient solution for generating MD5 checksums in PowerShell scripts.
The [System.Security.Cryptography.MD5]
class provides a method called ComputeHash()
to calculate the MD5 hash value. The syntax is as follows:
$md5 = [System.Security.Cryptography.MD5]::Create()
$hash = $md5.ComputeHash($data)
$md5
: Represents an instance of the MD5 class created using theCreate()
method.$hash
: Stores the computed MD5 hash value.$data
: Specifies the data for which the MD5 checksum needs to be calculated. This can be a byte array representing file contents or a string.
Here is an example:
$data = [System.Text.Encoding]::UTF8.GetBytes("MD5Example")
$md5 = [System.Security.Cryptography.MD5]::Create()
$hash = $md5.ComputeHash($data)
$hashString = [System.BitConverter]::ToString($hash) -replace '-', ''
Write-Output $hashString
In this code snippet, we convert the string MD5Example
into a byte array using [System.Text.Encoding]::UTF8.GetBytes()
. We then create an instance of the MD5 class using [System.Security.Cryptography.MD5]::Create()
and call the ComputeHash()
method to generate the MD5 hash value for the provided data.
Finally, we convert the hash value to a string format using [System.BitConverter]::ToString()
and remove any dashes in the output using the -replace
operator.
Output:
Using the MD5CryptoServiceProvider
Class in PowerShell
The MD5CryptoServiceProvider
class is part of the .NET Framework’s Cryptography namespace. This class provides functionality to compute the MD5 hash value of data using the MD5 cryptographic algorithm.
The MD5CryptoServiceProvider
class provides a method called ComputeHash()
to calculate the MD5 hash value. The syntax is as follows:
$md5 = New-Object -TypeName System.Security.Cryptography.MD5CryptoServiceProvider
$hash = $md5.ComputeHash($data)
$md5
: Represents an instance of theMD5CryptoServiceProvider
class created usingNew-Object
.$hash
: Stores the computed MD5 hash value.$data
: Specifies the data for which the MD5 checksum needs to be calculated. This can be a byte array representing file contents or a string.
Here is an example:
$data = [System.Text.Encoding]::UTF8.GetBytes("MD5Example")
$md5 = New-Object -TypeName System.Security.Cryptography.MD5CryptoServiceProvider
$hash = $md5.ComputeHash($data)
$hashString = [System.BitConverter]::ToString($hash) -replace '-', ''
Write-Output $hashString
In this code snippet, we convert the string MD5Example
into a byte array using [System.Text.Encoding]::UTF8.GetBytes()
. We then create an instance of the MD5CryptoServiceProvider
class using New-Object
and call the ComputeHash()
method to generate the MD5 hash value for the provided data.
Finally, we convert the hash value to a string format using [System.BitConverter]::ToString()
and remove any dashes in the output using the -replace
operator.
Output:
Conclusion
PowerShell offers multiple avenues for computing MD5 checksums, each suited to different scenarios and levels of complexity. Whether using built-in cmdlets like Get-FileHash
, harnessing the power of .NET classes such as System.Security.Cryptography.MD5
, or employing cryptographic providers like MD5CryptoServiceProvider
, PowerShell users have versatile tools at their disposal for ensuring data integrity and security.
By understanding and utilizing these methods effectively, PowerShell scripts can confidently handle tasks requiring MD5 checksums with precision and reliability.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn