How to Get Credential Using PowerShell
-
Using
Get-Credential
to Get Credential in PowerShell -
Using
Get-Credential
Cmdlet Without a Prompt to Get Credential in PowerShell
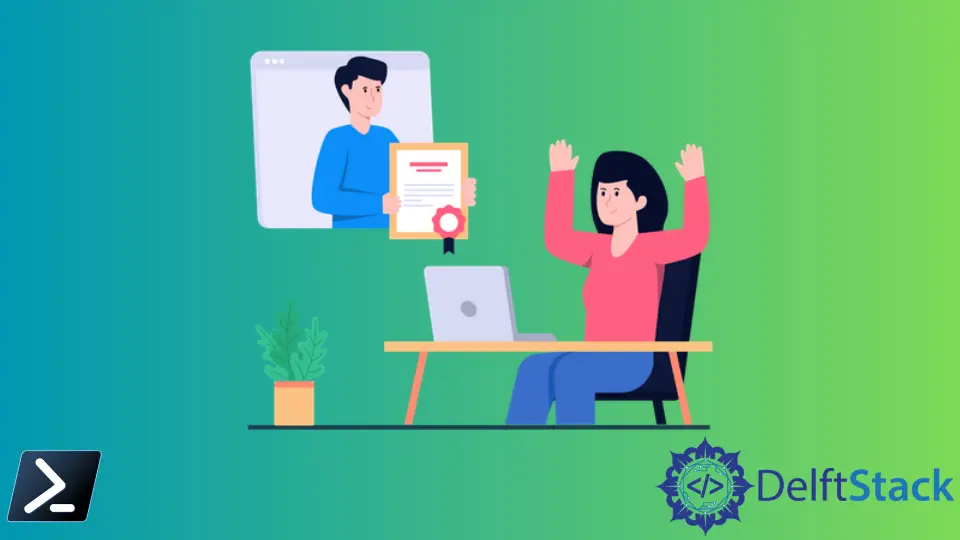
PSCredential objects are a creative way to securely store and pass credentials to various services. Many native and third-party cmdlets require PSCredential objects on many different commands. In addition, credentials are a ubiquitous object in Windows PowerShell.
This article will discuss how to use the Windows PowerShell Get-Credential
cmdlet and get a credential without any prompts.
Using Get-Credential
to Get Credential in PowerShell
Usually, to create a PSCredential object, we would use the Get-Credential
cmdlet. The said cmdlet is the most common way Windows PowerShell receives input to create the PSCredential object. This object mainly consists of the username and password.
Every time it’s executed, it will either prompt in the command line for the username and password, or a pop-up dialog box will appear asking for the user’s credentials.
Using Get-Credential
Cmdlet Without a Prompt to Get Credential in PowerShell
For example, we are working on an automated script that runs in a scheduled task or part of some more powerful automation framework. In this case, there’s no one operating the console to type in a username and password. So, it’s better to build a PSCredential object from scratch.
To create a PSCredential object with no interaction involves a process of encryption. The PSCredential object requires a plain-text username and an encrypted password.
To encrypt a password, we need to convert a standard string to a secure string. We do that by using the ConvertTo-SecureString
cmdlet. We can pass a plain-text password to this cmdlet, and because it is plain text, we have to use the -PlainText
and -Force
parameters. Both parameters indicate that we are passing a plain-text string with no requirement for confirmation.
$pass = ConvertTo-SecureString 'MySecretPassword' -AsPlainText -Force
Once a secure string is created, it’s time to make the PSCredential object. To do this, we can use the New-Object
cmdlet to define an object of System.Management.Automation.PSCredential
. The PSCredential class has a constructor that will accept the plain-text username and a secure string password that we can use as arguments.
$credential = New-Object System.Management.Automation.PSCredential ('user01', $pass)
We now have a PSCredential object with our plain-text username and secure string password saved in a variable. We can now pass the $credential
variable to several commands requiring a -Credential
parameter, which will work great. To verify if it was created with the expected username and password, we can reference the UserName
property, displaying the username you used earlier.
Example Code:
$credential.Username
Output:
user01
The password is encrypted through a secure string, but if you read the password on the same computer and logged in user, we can use the GetNetworkCredential()
method to see the password in plain text. So append GetNetworkCredential()
at the end of the $credential
variable but notice that you will not know the password printed on the console once executed.
Example Code:
$credential.GetNetworkCredential()
Output:
UserName Domain
-------- ------
user01
To see the password, you’ll need to use the Password
property on the object that GetNetworkCredential()
returns. The returned password should be the same password you provided to the PSCredential constructor.
Example Code:
$credential.GetNetworkCredential().Password
Output:
MySecretPassword
Now that we have verified that the $credential
variable holds both our username and passwords, we can use the variable as a parameter in the Get-Credential
cmdlet.
Get-Credential -Credential $credential
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn