How to Find the CPU and RAM Usage Using PowerShell
-
How to Find the CPU and RAM Usage Using PowerShell Using
Get-Counter
-
How to Find the CPU and RAM Usage Using PowerShell Using
Get-WmiObject
-
How to Find the CPU and RAM Usage Using PowerShell Using
Get-Process
- Conclusion
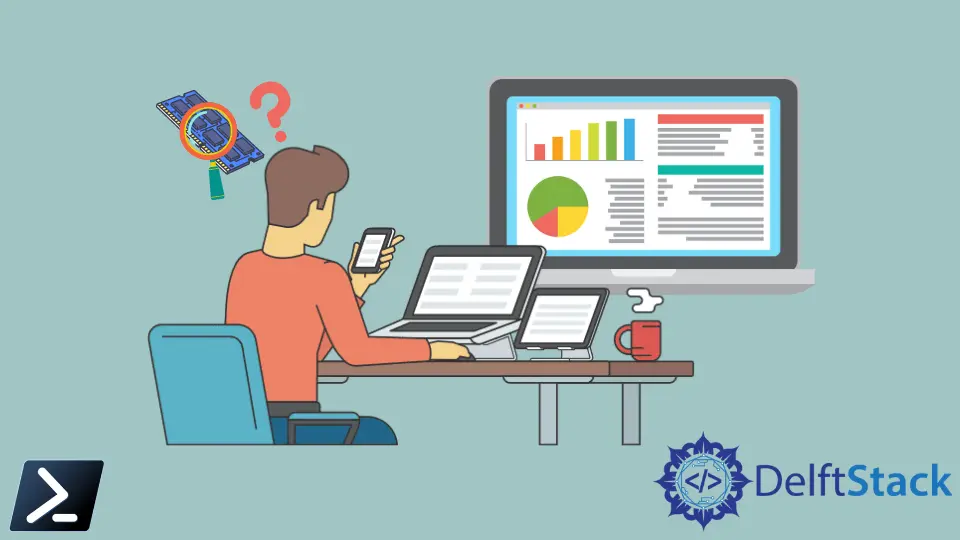
As technology advances, the need for efficient system monitoring becomes increasingly crucial. Windows administrators and users often find themselves seeking quick and effective ways to assess CPU and RAM usage for optimal system performance in their Windows machines.
In this article, we will explore the power of PowerShell, a versatile scripting language in the Windows environment, and discover how it can be used to find and monitor CPU and RAM usage seamlessly.
By utilizing PowerShell’s capabilities, users can gain real-time insights into their system’s memory usage, empowering them to make informed decisions and ensure a smooth computing experience.
How to Find the CPU and RAM Usage Using PowerShell Using Get-Counter
PowerShell, with its powerful cmdlets, offers a robust solution to retrieve real-time performance data. One such cmdlet, Get-Counter
, provides a versatile and efficient way to access this information.
The Get-Counter
cmdlet allows us to retrieve performance counter data from local or remote machines. To monitor CPU and RAM usage, we can use specific performance counter paths.
Here is the basic syntax:
Get-Counter -Counter "\CounterPath"
For CPU usage, the counter path is '\Processor(_Total)\% Processor Time'
, and for RAM usage, it is '\Memory\Available MBytes'
.
PowerShell Find the CPU and Memory Usage Using Get-Counter
Code Example
Let’s delve into a complete working code example that demonstrates how to use Get-Counter
to monitor both CPU and RAM usage:
# PowerShell get memory usage
Get-Counter '\Processor(_Total)\% Processor Time' -Continuous | ForEach-Object {
$cpuUsage = $_.CounterSamples.CookedValue
Write-Host "Current CPU Usage: $cpuUsage%"
}
Get-Counter '\Memory\Available MBytes' -Continuous | ForEach-Object {
$ramUsage = $_.CounterSamples.CookedValue
Write-Host "Available RAM: ${ramUsage}MB"
}
Let’s break down each section of the code to understand its functionality.
Monitoring CPU Usage:
Get-Counter '\Processor(_Total)\% Processor Time' -Continuous | ForEach-Object {
$cpuUsage = $_.CounterSamples.CookedValue
Write-Host "Current CPU Usage: $cpuUsage%"
}
In the first part, we use Get-Counter
to retrieve real-time data on the percentage of total processor time used. The specified counter path, '\Processor(_Total)\% Processor Time'
, targets the overall CPU usage across all cores.
The -Continuous
parameter ensures that the command runs continuously, providing live updates, and only stops until CTRL+C is pressed.
The output of Get-Counter
is then passed through a ForEach-Object
loop. For each received counter sample, the script extracts the processed value (CookedValue
), representing the current CPU usage percentage.
This value is stored in the variable $cpuUsage
, and a message displaying the current CPU usage is printed to the console using the PowerShell function Write-Host
.
Code Output:
Monitoring Memory Usage (RAM):
Get-Counter '\Memory\Available MBytes' -Continuous | ForEach-Object {
$ramUsage = $_.CounterSamples.CookedValue
Write-Host "Available RAM: ${ramUsage}MB"
}
The second part of the script focuses on monitoring the memory percentage. Similar to the CPU section, we use Get-Counter
with the counter path '\Memory\Available MBytes'
to continuously retrieve data on the available memory in megabytes.
The -Continuous
parameter ensures ongoing monitoring.
Again, the output is processed in a ForEach-Object
loop. For each counter sample received, the script extracts the processed value representing available bytes in RAM, which is stored in the variable $ramUsage
.
The final step involves displaying the available RAM information on the console using Write-Host
.
Code Output:
This real-time display of information is invaluable for system administrators and users alike, enabling them to promptly identify and address performance issues. Get-Counter
simplifies the process of monitoring CPU and RAM usage, making it an essential tool in the PowerShell arsenal.
How to Find the CPU and RAM Usage Using PowerShell Using Get-WmiObject
In addition to the Get-Counter
cmdlet, PowerShell offers another powerful tool, Get-WmiObject
, for gathering system information, including CPU and RAM usage.
The Get-WmiObject
cmdlet allows us to access Windows Management Instrumentation (WMI) classes, providing a wealth of information about the system. To monitor CPU and physical memory usage, specific WMI classes and properties are queried.
The basic syntax is as follows:
Get-WmiObject -Class WMI_Class | Select-Object Property1, Property2, ...
For CPU usage, we use the Win32_Processor
class, and for RAM or physical memory usage, the Win32_OperatingSystem
class.
PowerShell Find the CPU and RAM Usage Using Get-WmiObject
Code Example
Let’s explore a complete working code example that demonstrates how to use Get-WmiObject
to monitor both CPU and RAM usage:
# PowerShell get memory usage of all the processes
$cpuObject = Get-WmiObject -Class Win32_Processor
$cpuLoadPercentage = $cpuObject.LoadPercentage
Write-Host "CPU Load Percentage: $cpuLoadPercentage"
$ramObject = Get-WmiObject -Class Win32_OperatingSystem
$ramUsageGB = [math]::Round(($ramObject.TotalVisibleMemorySize - $ramObject.FreePhysicalMemory) / 1GB, 2)
Write-Host "Available RAM: ${ramUsageGB}GB"
Let’s break down each section of the PowerShell script above to understand its functionality.
Monitoring CPU Usage:
$cpuObject = Get-WmiObject -Class Win32_Processor
$cpuLoadPercentage = $cpuObject.LoadPercentage
Write-Host "CPU Load Percentage: $cpuLoadPercentage"
Here, the script first utilizes Get-WmiObject
to query information from the Win32_Processor
class, which contains details about the system’s processor. The obtained information is stored in the variable $cpuObject
.
Subsequently, the script extracts the LoadPercentage
property from $cpuObject
, representing the current CPU load percentage. This value is then stored in the variable $cpuLoadPercentage
.
Finally, a message displaying the CPU load percentage is printed to the console using Write-Host
.
Code Output:
Monitoring Memory Usage (RAM):
$ramObject = Get-WmiObject -Class Win32_OperatingSystem
$ramUsageGB = [math]::Round(($ramObject.TotalVisibleMemorySize - $ramObject.FreePhysicalMemory) / 1GB, 2)
Write-Host "Available RAM: ${ramUsageGB}GB"
In the RAM monitoring section, the script employs Get-WmiObject
to retrieve information from the Win32_OperatingSystem
class, which contains various properties related to the operating system, including memory-related details. The acquired information is stored in the variable $ramObject
.
Next, the script calculates the available RAM in gigabytes by subtracting the free physical memory (FreePhysicalMemory
) from the total physical memory or total visible memory size (TotalVisibleMemorySize
). The result is then rounded to two decimal places using [math]::Round
to ensure a cleaner output.
The available RAM value in gigabytes is stored in the variable $ramUsageGB
. Finally, a message displaying the available RAM is printed to the console using Write-Host
.
Code Output:
This information, retrieved using the Get-WmiObject
cmdlet, provides valuable insights into the system’s performance, allowing users to effectively monitor resource utilization and make informed decisions for system optimization. The script’s simplicity and clarity make it a versatile tool for administrators and users alike.
How to Find the CPU and RAM Usage Using PowerShell Using Get-Process
As part of our exploration into monitoring system performance with PowerShell, we will now focus on the Get-Process
cmdlet. Unlike Get-Counter
and Get-WmiObject
, Get-Process
provides information about running processes on the local machine, allowing us to extract CPU and memory percentage details.
The Get-Process
cmdlet retrieves information about the currently running processes on a local or remote computer. To monitor CPU and RAM usage, we will filter and extract relevant information from the output.
The basic syntax is as follows:
Get-Process -Name "ProcessName" | Select-Object Property1, Property2, ...
For CPU usage, we will focus on the CPU
property, and for RAM usage, the WorkingSet
property.
PowerShell Find the CPU and RAM Usage Using Get-Process
Code Example
Let’s explore a complete working code example that demonstrates how to use Get-Process
to monitor both CPU and RAM usage:
# PowerShell get memory usage
$cpuProcesses = Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
ForEach ($process in $cpuProcesses) {
$processName = $process.ProcessName
$cpuUsage = $process.CPU
Write-Host "Process: $processName, CPU Usage: $cpuUsage%"
}
$ramProcesses = Get-Process | Sort-Object WorkingSet -Descending | Select-Object -First 5
ForEach ($process in $ramProcesses) {
$processName = $process.ProcessName
$ramUsageMB = [math]::Round($process.WorkingSet / 1MB, 2)
Write-Host "Process: $processName, RAM Usage: ${ramUsageMB}MB"
}
Similar to the previous examples, this PowerShell script is also organized into two sections: one focusing on CPU usage and the other on RAM usage. Let’s break down each section of the code.
Monitoring CPU Usage:
$cpuProcesses = Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
ForEach ($process in $cpuProcesses) {
$processName = $process.ProcessName
$cpuUsage = $process.CPU
Write-Host "Process: $processName, CPU Usage: $cpuUsage%"
}
In the first code section, the script starts by using Get-Process
to retrieve information about all running processes on the system. The output is then sorted in descending order based on CPU usage using Sort-Object
.
The Select-Object -First 5
ensures that only the top 5 processes with the highest CPU usage are considered. The resulting processes are stored in the variable $cpuProcesses
.
A ForEach loop
is employed to iterate through each process object in $cpuProcesses
. For each process, the script extracts the process name (ProcessName
) and CPU usage (CPU
).
This information is then displayed on the console using Write-Host
, presenting the process name along with its corresponding CPU usage percentage.
Code Output:
Monitoring Memory Usage (RAM):
$ramProcesses = Get-Process | Sort-Object WorkingSet -Descending | Select-Object -First 5
ForEach ($process in $ramProcesses) {
$processName = $process.ProcessName
$ramUsageMB = [math]::Round($process.WorkingSet / 1MB, 2)
Write-Host "Process: $processName, RAM Usage: ${ramUsageMB}MB"
}
In the second code section, a similar approach is taken. Again, Get-Process
is used to gather information about all running processes.
The output is then sorted based on the RAM usage (WorkingSet
) in descending order, and only the top 5 processes running are selected. The resulting processes are stored in the variable $ramProcesses
.
A ForEach
loop iterates through each process in $ramProcesses
. For each process, the script extracts the process name and calculates the RAM usage in megabytes.
The calculation involves dividing the working set size by 1 megabyte and rounding the result to two decimal places using [math]::Round
. The information is then displayed on the console using Write-Host
, presenting the process name along with its corresponding RAM usage in megabytes.
Code Output:
This script provides a dynamic approach to monitoring CPU and memory usage, focusing on the top-consuming processes. By leveraging the capabilities of Get-Process
, administrators and users can gain insights into resource-intensive activities on their systems.
Conclusion
Monitoring CPU and RAM usage is essential for maintaining a healthy and responsive computing environment. PowerShell, with its rich set of cmdlets, provides powerful tools like Get-Counter
, Get-WmiObject
, and Get-Process
to streamline this process.
Whether you prefer real-time updates, detailed WMI class information, or insights into individual processes, PowerShell offers flexible solutions to cater to diverse monitoring needs. By incorporating these techniques into your system management toolkit, you can effortlessly stay informed about CPU and RAM usage, enabling timely interventions and proactive system optimization.