The filter Keyword in PowerShell
- Understanding the Filter Keyword
- Using the Filter Keyword with Get-ChildItem
- Filtering Processes with Get-Process
- Filtering Services with Get-Service
- Conclusion
- FAQ
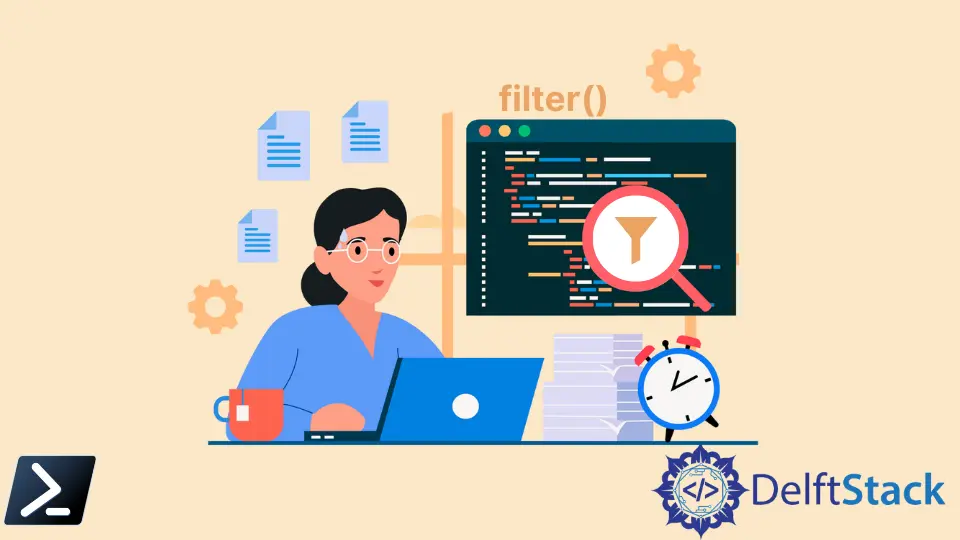
PowerShell is a powerful tool for system administration and automation, and one of its most useful features is the filter keyword.
This tutorial is designed to teach you how to effectively use the filter keyword in PowerShell to streamline your tasks and improve your workflow. Whether you’re managing files, querying databases, or automating repetitive tasks, understanding how to filter data can save you time and enhance your productivity.
In this article, we’ll explore the various ways to utilize the filter keyword, providing practical examples and clear explanations to help you master this essential PowerShell feature. Let’s dive in!
Understanding the Filter Keyword
The filter keyword in PowerShell allows users to specify criteria that limit the data returned by commands. This is particularly useful when working with large datasets or when you only need specific information. By applying filters, you can focus on the data that matters most to you, making your scripts more efficient and easier to read.
In PowerShell, the filter keyword is often used in conjunction with cmdlets like Get-ChildItem
, Get-Process
, and Get-Service
. For example, if you want to list only specific files in a directory, you can use the filter keyword to refine your search. This approach not only optimizes your scripts but also enhances performance by reducing the amount of data processed.
Using the Filter Keyword with Get-ChildItem
One of the most common uses of the filter keyword is with the Get-ChildItem
cmdlet, which retrieves the files and folders in a specified location. By leveraging the filter keyword, you can specify file types or names, making it easier to find exactly what you need.
Here’s a simple example of using the filter keyword to list all .txt
files in a directory:
Get-ChildItem -Path "C:\MyFolder" -Filter "*.txt"
Output:
File1.txt
File2.txt
Notes.txt
In this example, the -Path
parameter specifies the directory to search, while the -Filter
parameter restricts the results to only those files with a .txt
extension. This command is efficient because it only retrieves the files that match the specified filter, minimizing the amount of data processed.
Using the filter keyword in this way can greatly enhance your productivity. Instead of sifting through a long list of files, you can quickly access the specific ones you need. Additionally, this method reduces the load on system resources, making your scripts run faster and more efficiently.
Filtering Processes with Get-Process
Another powerful application of the filter keyword is with the Get-Process
cmdlet. This cmdlet allows you to retrieve information about the processes running on your system. By using the filter keyword, you can narrow down the list to only the processes that are relevant to your needs.
Here’s an example of how to filter processes by name:
Get-Process -Name "chrome" -Filter "chrome*"
Output:
Handles NPM(K) PM(K) WS(K) VM(M) CPU(s) Id ProcessName
------- ------ ----- ----- ----- ------ -- -----------
123 10 15000 20000 50 0.5 1234 chrome
124 11 16000 21000 55 0.6 1235 chrome
In this case, the -Name
parameter is used to specify the process you want to filter, while the -Filter
parameter helps to match any processes that start with “chrome”. This is particularly useful when dealing with applications that may have multiple instances running simultaneously.
By filtering processes, you can easily monitor specific applications, troubleshoot issues, or gather performance metrics without being overwhelmed by unrelated data. It simplifies your workflow and allows you to focus on what’s important.
Filtering Services with Get-Service
The Get-Service
cmdlet is another area where the filter keyword shines. It allows you to retrieve the status of services on your system. By applying filters, you can quickly identify which services are running, stopped, or disabled.
Here’s how you can filter services by their status:
Get-Service -Status Running -Filter "w*"
Output:
Status Name DisplayName
------ ---- -----------
Running wuauserv Windows Update
Running w3svc World Wide Web Publishing Service
In this example, the -Status
parameter is used to focus on services that are currently running, while the -Filter
parameter narrows the search to services whose names start with “w”. This allows you to quickly assess the status of relevant services without having to sift through the entire list.
Using the filter keyword with Get-Service
can be particularly beneficial for system administrators who need to manage multiple services efficiently. It enables you to keep your system running smoothly by quickly identifying any issues with essential services.
Conclusion
The filter keyword in PowerShell is an invaluable tool for anyone looking to enhance their scripting capabilities. By learning how to effectively use filters with various cmdlets like Get-ChildItem
, Get-Process
, and Get-Service
, you can streamline your tasks, improve performance, and focus on the data that matters most. With practice, you’ll find that filtering not only simplifies your scripts but also makes your work more efficient. So, dive into PowerShell, and start harnessing the power of the filter keyword today!
FAQ
-
what is the filter keyword in PowerShell?
The filter keyword is used to specify criteria that limit the data returned by commands in PowerShell, making it easier to find specific information. -
how do I use the filter keyword with Get-ChildItem?
You can use the filter keyword with Get-ChildItem by specifying the -Filter parameter followed by the criteria, such as file extensions. -
can I filter processes in PowerShell?
Yes, you can filter processes using the Get-Process cmdlet by applying the -Name and -Filter parameters to narrow down the results. -
what is the benefit of using the filter keyword?
Using the filter keyword improves performance and efficiency by reducing the amount of data processed and helping you focus on relevant information. -
how can I filter services in PowerShell?
You can filter services using the Get-Service cmdlet by applying the -Status and -Filter parameters to view only the services that meet your criteria.