Different Parameters in PowerShell
- Parameters in PowerShell
- Named Parameters
- Parameter Defaults
- Switch Parameters
- Mandatory Parameters
- Pipeline Parameters
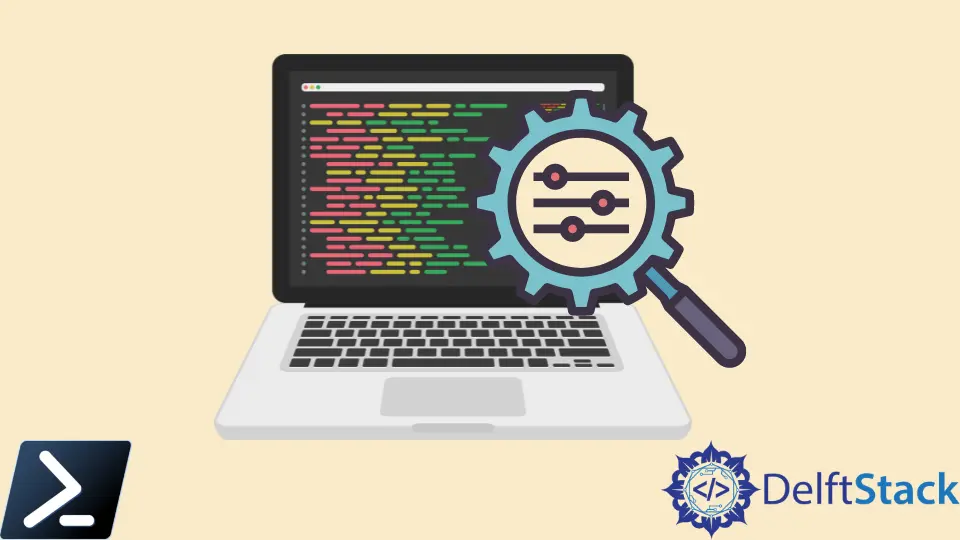
In this article, we will learn what parameter function is, different kinds of variables that we can define in a parameter, different types of parameters, and their sample uses.
Parameters in PowerShell
Administrators like us can create parameters for scripts and functions using the parameter param()
function. The parameter script block contains one or more parameters signified by variables.
Example Code:
param ($myVariable)
However, to ensure the parameter declared accepts only the type of input we need, best practices indicate assigning a data type to the parameter by enclosing the data type with square brackets []
before the variable.
Example Code:
param ([String]$myVariable)
Named Parameters
One way to use parameters in a script is via named parameters. When calling a function or script via named parameters, we use the variable name as the full name of the parameter.
Example Code:
param ([String]$name)
We can then use the named parameters to argue when executing a .ps1
file.
Example Code:
powershell.exe .\sample.ps1 -name "John"
Parameter Defaults
We can assign a value to a parameter by giving the parameter a default value beforehand inside the script. Then, running the script without passing values to the console will take the default value of the variable pre-defined inside the script.
Example Code:
param ([String]$name = "John")
Switch Parameters
Another parameter type that we can use is the switch parameter defined by the [switch]
data type. The switch parameter is used for Boolean values to indicate true
or false
.
Example Code:
param ([switch]$isEnabled)
Mandatory Parameters
Usually, we have one or more required parameters that must be used when executing a script. Therefore, our script may fail if we don’t have values assigned for these parameters.
We can make a parameter mandatory by inserting a Mandatory
data type inside the parameter data type block [Parameter()]
.
Example Code:
param (
[Parameter(Mandatory)]
[String] $servername
)
If left without value, PowerShell will not allow the script to run and prompt you for a value when executing. It is worth noting that parameters without the [Parameter(Mandatory)]
block are considered optional parameters.
Pipeline Parameters
Most PowerShell cmdlets let you use the pipe (|
) symbol to pass data. We can take advantage of this PowerShell feature when working with parameters.
Using this method will open up many opportunities for running chained PowerShell files.
File - pipeline.ps1
:
param([parameter(ValueFromPipeline)]$pipedVar)
Write-Output $pipedVar
Example Code:
"This string is from the pipeline." | .\pipeline.ps1
Output:
This string is from the pipeline.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn