How to Create Log Files in PowerShell
- Writing Output to Log Files Using a Custom PowerShell Script
-
Writing Output to Log Files in Using
Start-Transcript
in PowerShell
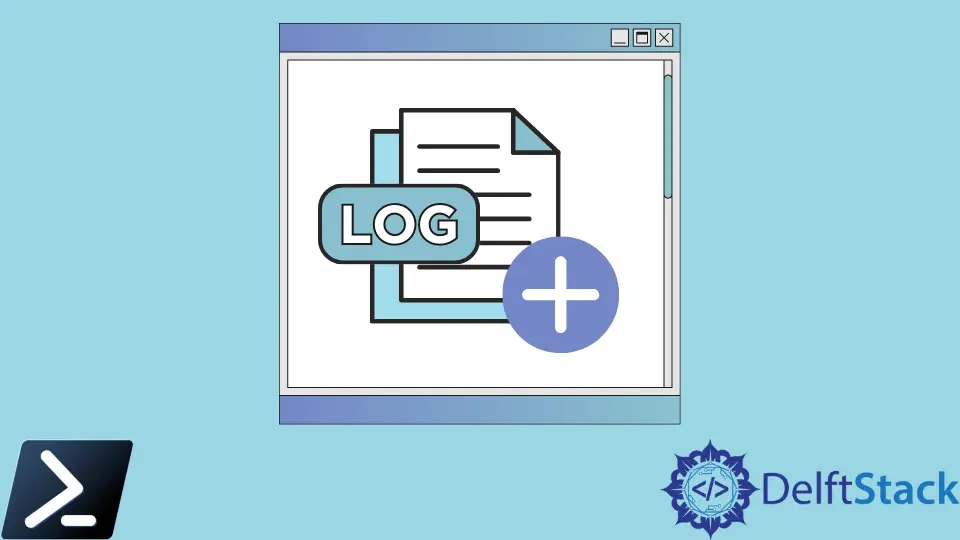
System logging is essential to track the history of actions taken on our computers.
There are multiple types of logging in some scripting languages. They have their built-in function to track logging in the development.
Unfortunately, Windows PowerShell doesn’t have logging turned on by default. This article will show us how to create and enable logging when running our scripts.
Writing Output to Log Files Using a Custom PowerShell Script
We can use simple text log files to control running and track all of the activities in our PowerShell scripts. This method is proper when debugging errors or auditing script actions.
If we want to write the Windows PowerShell command result to a text log file, we can use one of the following formats to redirect the PS result to a text file:
Example Code/s:
Write-Output "Log Files are successfully created in $env:computername" >> C:\PS\Logs\TestLog1.txt
Add-Content -Path C:\PS\Logs\TestLog2.txt -Value "Log Files are successfully created in $env:computername"
"Log Files are successfully created in $env:computername" | Out-File -FilePath C:\PS\Logs\TestLog3.txt -Append
The snippets add a new line to a text file with the specified text in all cases.
If we want to overwrite our TestLog.txt, we can use the Set-Content
cmdlet. However, this wouldn’t be good practice when we want to set historical records of our previous script runs.
The main drawback is that we cannot determine when the script wrote an entry to the log (an event occurred).
We can add the current timestamp to the log file. It will help identify when we ran the script and a specific event.
We can create a separate function in our PowerShell script that will export the data it receives to a file and add the timestamp for each event to make it more convenient.
Example Code:
$Logfile = "C:\PS\Logs\proccess_$env:computername.log"
function WriteLog {
Param ([string]$LogString)
$TimeLog = (Get-Date).toString("yyyy/MM/dd HH:mm:ss")
$LogMessage = "$TimeLog $LogString"
Add-Content $LogFile -Value $LogMessage
}
Then call the WriteLog
function if we want to log something.
Example Code:
WriteLog "The script is running."
WriteLog "Calculating…"
Start-Sleep 20
WriteLog "The script is successfully executed."
After running the cmdlet, a message appears showing the file the output of all commands is logged.
Output:
Transcript started, output file is C:\temp\PowerShell_transcript.LAPTOP01.8qaVt7PE.20220120183616.txt
If we choose to save our transcripts in a different location, we can specify the path to the text file using the snippet below:
Example Code:
Start-Transcript -Append C:\PS\Logs\PSScriptLog.txt
The -Append
option indicates that the script will log new sessions to the end of the file.
Run some Windows PowerShell cmdlets that export the results to the console.
For example, let’s export a list of running services on your current machine:
Get-Service | Where-Object { $_.status -eq 'Running' }
Stop logging for the current PowerShell session using the cmdlet below:
Stop-Transcript
The log file will show the entire history of the PowerShell commands run in the command line, including the errors, warnings, and output. It is convenient when debugging complex PowerShell scripts.
You can use Start-Transcript
and Stop-Transcript
cmdlets in your PowerShell scripts to log all actions and outputs natively.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn