How to Create a Shortcut Using PowerShell
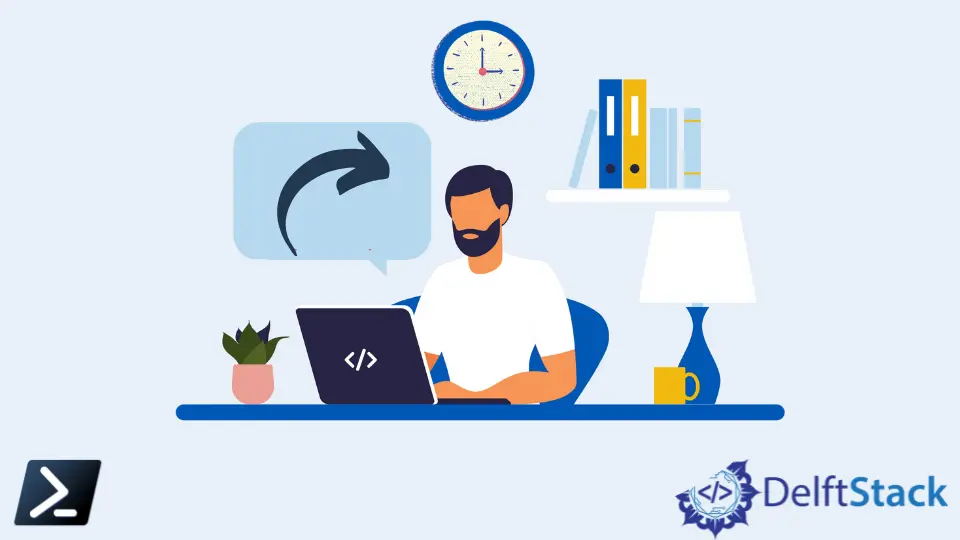
A shortcut is a link that can point to any program, file, or folder on the system. Having a shortcut on the desktop or taskbar eliminates the need for users to search through multiple folders on the computer.
In Windows, a shortcut file contains the target path of the original file and has a .lnk
extension with an arrow in the bottom left corner of the icon. When you open a shortcut file, it will open the original file.
It takes very small space in the disk. This article will teach you to create a shortcut using PowerShell.
Use ComObject
to Create a Shortcut Using PowerShell
You will need a WScript.Shell
type of COM
object to create a shortcut with PowerShell. The New-Object
cmdlet creates a new instance of the .NET
Framework or COM
object.
You can create a COM
object using the ComObject
parameter. The following command creates a COM
object that represents WScript.Shell
and assigns it to a variable $WScriptShell
.
$WScriptShell = New-Object -ComObject WScript.Shell
Now, let’s define the location of a file to create its shortcut. Here, we are creating a shortcut for the Typora
application.
$TargetFile = "C:\Program Files\Typora\Typora.exe"
Then define the name and location for your shortcut file.
$ShortcutFile = "C:\Users\rhntm\OneDrive\Desktop\Typora.lnk"
After that, use the CreateShortcut
method and assign it to a variable, as shown below.
$Shortcut = $WScriptShell.CreateShortcut($ShortcutFile)
The next step is to add the Target path to the $TargetFile
variable.
$Shortcut.TargetPath = $TargetFile
Lastly, invoke the Save()
method and save your shortcut.
$Shortcut.Save()
The shortcut is created as Typora.lnk
on the specified location.
Get-ChildItem "C:\Users\rhntm\OneDrive\Desktop"
Output:
Directory: C:\Users\rhntm\OneDrive\Desktop
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 1/30/2022 11:34 AM 970 Typora.lnk
The full script should be like this:
$WScriptShell = New-Object -ComObject WScript.Shell
$TargetFile = "C:\Program Files\Typora\Typora.exe"
$ShortcutFile = "C:\Users\rhntm\OneDrive\Desktop\Typora.lnk"
$Shortcut = $WScriptShell.CreateShortcut($ShortcutFile)
$Shortcut.TargetPath = $TargetFile
$Shortcut.Save()
You can also use this function in your PowerShell script to create a shortcut.
function createShortcut {
param ([string]$TargetFile, [string]$ShortcutFile)
$WScriptShell = New-Object -ComObject WScript.Shell
$Shortcut = $WScriptShell.CreateShortcut($ShortcutFile)
$Shortcut.TargetPath = $TargetFile
$Shortcut.Save()
}
createShortcut "C:\Program Files\Greenshot\Greenshot.exe" "C:\New\Greenshot.lnk"