How to Get Command Line Arguments in Windows PowerShell
- Define Parameters in PowerShell Script
- Named Parameters in PowerShell Script
- Assign Default Values to Parameters in PowerShell Script
- Switch Parameters in PowerShell Script
- Mandatory Parameters in PowerShell Script
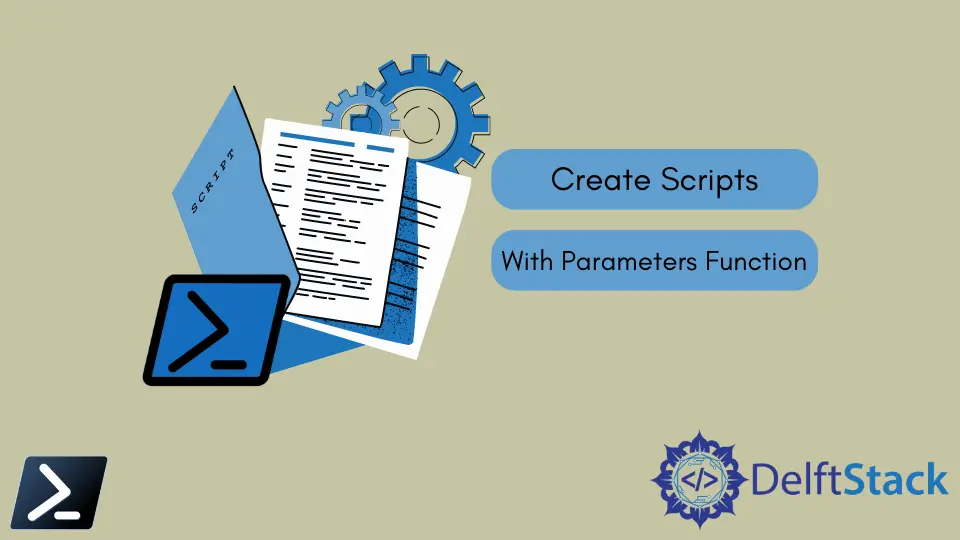
We handle arguments using the Windows PowerShell parameter function called param()
. The Windows PowerShell parameter function is a fundamental component of any script. A parameter is how developers enable script users to provide input at runtime.
In this article, you’ll learn how to create scripts with the parameter function, use them, and some best practices for building parameters.
Define Parameters in PowerShell Script
Administrators can create parameters for scripts and functions using the parameter function param()
. A function contains one or more parameters defined by variables.
Hello_World.ps1
:
param(
$message
)
However, to ensure the parameter accepts only the type of input you need, best practices dictate assigning a data type to the parameter with the use of a parameter block [Parameter()]
and enclosing the data type with square brackets []
before the variable.
Hello_World.ps1
:
param(
[Parameter()]
[String]$message
)
In the example Hello_World.ps1
above, the variable message
will only accept a passed value if the given value has a data type String
.
Named Parameters in PowerShell Script
One way to use parameter function in a script is via parameter name – this method is called named parameters. When calling a script or function via named parameters, we use the variable name as the full name of the parameter.
We created a Hello_World.ps1
and defined variables inside the parameter function in this example. Remember that we can put one or more variables inside the parameter function.
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion
)
Write-Output $message
Write-Output "I am $emotion"
We can then use the named parameters as an argument when executing a .ps1
file.
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy'
Output:
Hello World!
I am happy
Assign Default Values to Parameters in PowerShell Script
We can pre-assign a value to a parameter by giving the parameter a value inside of the script. Then, running the script without passing values from the execution line will take the default value of the variable defined inside the script.
param(
[Parameter()]
[String]$message = "Hello World",
[String]$emotion = "happy"
)
Write-Output $message
Write-Output "I am $emotion"
.\Hello_World.ps1
Output:
Hello World!
I am happy
Switch Parameters in PowerShell Script
Another parameter that we can use is the switch parameter defined by the [switch]
data type. The switch parameter is used for binary or Boolean values to indicate true
or false
.
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion,
[Switch]$display
)
if ($display) {
Write-Output $message
Write-Output "I am $emotion"
}
else {
Write-Output "User denied confirmation."
}
We can call our script with the script parameter using the syntax below.
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy' -display:$false
Output:
User denied confirmation.
Mandatory Parameters in PowerShell Script
It’s common to have one or more parameters that must be used when a script is executed. We can make a parameter mandatory by adding a Mandatory
attribute inside the parameter block [Parameter()]
.
Hello_World.ps1:
param(
[Parameter(Mandatory)]
[String]$message,
[String]$emotion,
[Switch]$display
)
if ($display) {
Write-Output $message
Write-Output "I am $emotion"
}
else {
Write-Output "User denied confirmation."
}
When executing Hello_World.ps1
, Windows PowerShell will not allow the script to run and prompt you for a value.
.\Hello_World.ps1
Output:
cmdlet hello_world.ps1 at command pipeline position 1
Supply values for the following parameters:
message:
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn