All Replace Methods in PowerShell
-
Introduction to the
replace()
Method in PowerShell -
Use the
replace()
Method in PowerShell - Removing Characters in PowerShell
- Replacing Multiple Instances in PowerShell
- Using the Replace Operator in PowerShell
- Removing Characters in PowerShell
- Replacing Multiple Instances in PowerShell
- Replacing Strings Using Regex in PowerShell
- Escaping Regex Characters in PowerShell
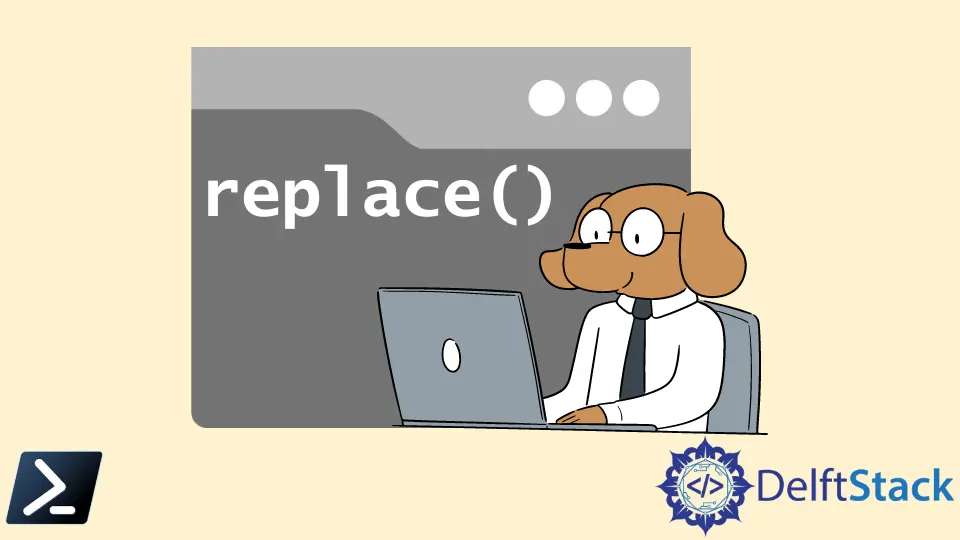
Windows PowerShell can work with texts and strings like many other languages. One of those valuable features is using Windows PowerShell to replace characters, strings, or even text inside files.
This article will discuss replacing or deleting characters, texts, and words using different PowerShell replace methods.
Introduction to the replace()
Method in PowerShell
We can use Windows PowerShell to replace characters in strings. For example, create a PowerShell variable with a data type string that we will use throughout this article.
$string = 'hello, world'
We want to replace the hello
word inside the string with the hi
word to make the $string
variable have a value of hi, world
.
To execute that, Windows PowerShell needs to figure out where to find the specific part of the text, and once it’s found, it replaces that portion of the text with a user-supplied value.
Use the replace()
Method in PowerShell
One of the most convenient ways to replace strings in Windows PowerShell is to use the replace()
method. The replace()
method accepts two arguments.
- The string to find.
- The string to replace the found text.
As we can see below, Windows PowerShell locates the word hello
and replace it with the string hi
. The method returns the final result, hi, world
.
Let’s use our previously created $string
variable as an example.
Code:
$string.replace('hello', 'hi')
Output:
hi, world
We can invoke the replace()
method on any character to replace any literal string with another. The replace()
method returns nothing if the string to be replaced isn’t found.
In addition, we don’t need to assign a string to a variable to replace text in a string. Instead, we can directly call the replace()
method on the string.
Code:
'hello world'.replace('hello', 'hi')
Removing Characters in PowerShell
Maybe we need to remove characters in a text from another string rather than replace it with something else. We can also do that by specifying an empty string.
Code:
$string.replace('hello', '')
Output:
, world
Replacing Multiple Instances in PowerShell
Since the replace()
method returns a value with a string data type, to replace another instance, we can connect and append another replace()
method call at the end. Windows PowerShell then invokes the replace()
method on the actual output.
Code:
$string.replace('hello', '').replace('world', 'earth')
Output:
, earth
We can chain together as many replace()
method calls as necessary, but we should consider using the replace operator if we have multiple strings to replace.
Using the Replace Operator in PowerShell
We can also use the replace operator of Windows PowerShell to replace a text. The replace operator is similar to the method we provide a string to find and replace.
But, it has one significant advantage: using regular expressions (regex) to find matching strings. Using the previous example, we can use the replace operator to replace hello
with hi
similarly, as shown below.
Code:
$string -replace 'hello', 'hi'
Output:
hi, world
Removing Characters in PowerShell
Like the replace()
method, we can also delete characters from a string using the replace operator. Unlike the replace()
method, we can also wholly exclude the string as an argument to replace with, and you’ll discover the same effect.
Code:
$string -replace 'hello', ''
$string -replace 'hello'
Output:
, world
, world
Replacing Multiple Instances in PowerShell
We can also chain together the usages of the replace operator.
Code:
$string -replace 'hello', 'hi' -replace 'world', 'earth'
Output:
hi, earth
Replacing Strings Using Regex in PowerShell
Replacing strings in PowerShell with the replace()
is limited. We are constrained only to use literal strings.
We cannot use wildcards or regex. If you’re performing intermediate or advanced, we should use the replace operator.
We have a script containing a string created with a variable. We want to change the string to goodbye, world
regardless of the value.
It would help transform hello, world
and hi, world
into goodbye, world
. We need to use a regular expression and match just about any specific pattern in text with regex to make this happen.
In this example, we can use the expression hello|hi
to match both required strings using the regex "or"
operator or the pipe (|
) character.
Code:
'hello, world' -replace 'hello|hi', 'goodbye'
'hi, world' -replace 'hello|hi', 'goodbye'
Output:
goodbye, world
goodbye, world
Once we learn how to wield regex to find strings, we can use PowerShell to replace wildcard strings that match any pattern.
Escaping Regex Characters in PowerShell
In the regex example above, the string to search in did not contain any special regex characters. However, the regular expression language has certain characters it uses that are not interpreted literally like most letters and numbers.
For example, perhaps we need to replace text in a string. That string contains a couple of special regex characters like a bracket and a question mark.
We then try to replace the string [hello]
with a goodbye
.
Code:
PS> '[hello], world' -replace '[hello]', 'goodbye'
Output:
[goodbyegoodbyegoodbyegoodbyegoodbye], wgoodbyergoodbyed
That’s not what we intended. This scenario happens when we use special regex characters inside the string to find ([hello]
).
To avoid this problem, we can either escape these special characters by prepending a backslash to the front of each character or using the Escape()
method. Below we can see the effect of escaping each special character with a backslash.
Code:
'[hello], world' -replace '\[hello\]', 'goodbye'
Output:
goodbye, world
Alternatively, we can use the regex type’s Escape()
method to remove all special characters automatically.
Code:
'[hello], world' -replace ([regex]::Escape('[hello]')), 'goodbye'
Output:
goodbye, world
We should use the Escape()
method because it will escape all special characters, and we don’t have to remember them.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn