How to Autoload Classes in PHP
- Understanding the Basics of Autoloading
- Implementing Autoloading with spl_autoload_register
- Organizing Classes with Namespaces
- Advanced Autoloading Techniques
- Conclusion
- FAQ
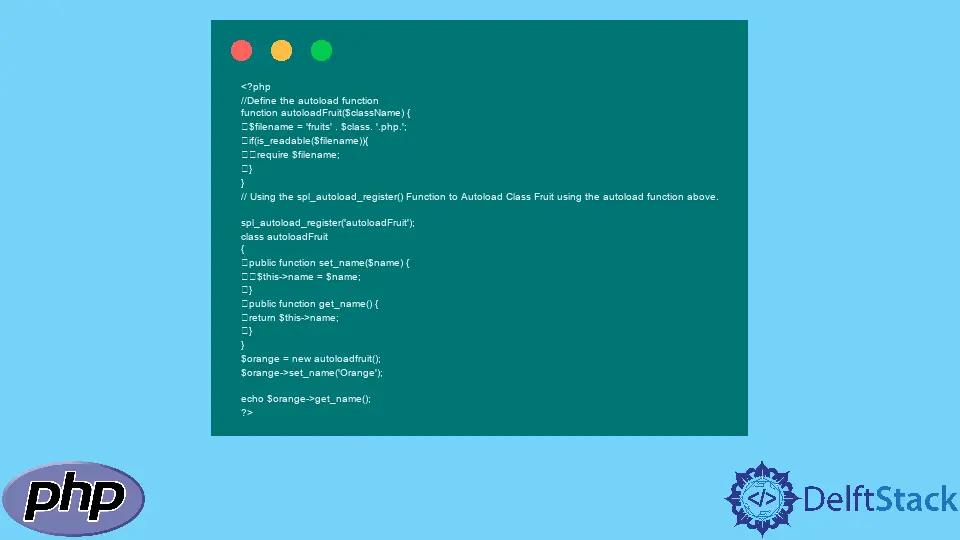
Autoloading classes in PHP is a powerful feature that streamlines the development process by automatically loading class files as needed. Instead of manually including or requiring class files, PHP offers an elegant solution through the spl_autoload_register
function. This function allows developers to define a custom autoloading mechanism, making it easier to manage large codebases and adhere to best practices like the PSR-4 standard.
In this article, we will explore how to use spl_autoload_register
effectively, providing clear examples and explanations to help you understand the process. By the end, you will be equipped with the knowledge to implement autoloading in your own PHP projects seamlessly.
Understanding the Basics of Autoloading
Before diving into the implementation, it’s essential to grasp the concept of autoloading. In PHP, when you instantiate a class that hasn’t been defined yet, PHP will throw an error. Autoloading allows you to define a function that PHP will call whenever it encounters an undefined class. This function will then include the necessary class file automatically.
Using spl_autoload_register
, you can register multiple autoload functions, which gives you flexibility in organizing your code. This method is particularly beneficial in larger applications where managing numerous class files can become cumbersome.
Implementing Autoloading with spl_autoload_register
Let’s see how to implement autoloading using spl_autoload_register
. The following example demonstrates a simple autoloading mechanism for a project that contains multiple classes.
spl_autoload_register(function ($class_name) {
include $class_name . '.php';
});
// Now you can create an instance of a class without including it manually
$myClass = new MyClass();
In this example, we register an anonymous function with spl_autoload_register
. The function takes the class name as a parameter and includes the corresponding file. If you have a class named MyClass
, PHP will look for a file named MyClass.php
.
This approach eliminates the need for multiple include
or require
statements, making your code cleaner and more maintainable. As your project grows, you can easily extend the autoload function to accommodate different directories or naming conventions.
The beauty of this method lies in its simplicity and flexibility. You can modify the autoload function to handle different file structures or naming conventions, allowing you to adapt it to your project’s needs.
Organizing Classes with Namespaces
To enhance code organization and prevent class name collisions, you can use namespaces in your classes. This is particularly useful in larger applications where multiple libraries may have classes with the same name.
Here’s how you can implement autoloading with namespaces:
spl_autoload_register(function ($class_name) {
$file = str_replace('\\', DIRECTORY_SEPARATOR, $class_name) . '.php';
include $file;
});
// Using a namespaced class
$myClass = new MyNamespace\MyClass();
In this code snippet, we replace the namespace separator (\
) with the directory separator (DIRECTORY_SEPARATOR
) to construct the file path. This way, if your class is in the MyNamespace
namespace, PHP will look for the file in the corresponding folder structure.
Using namespaces not only keeps your classes organized but also enhances the readability of your code. It allows you to create a logical structure for your project, making it easier to navigate and maintain.
Advanced Autoloading Techniques
As your application grows, you might want to implement more advanced autoloading techniques. For instance, you can create a more sophisticated autoload function that checks multiple directories for the class files.
Here’s an example of how to implement this:
spl_autoload_register(function ($class_name) {
$directories = ['src/', 'lib/', 'vendor/'];
foreach ($directories as $directory) {
$file = $directory . $class_name . '.php';
if (file_exists($file)) {
include $file;
return;
}
}
});
// You can now instantiate classes from different directories
$myClass = new MyClass();
In this example, we define an array of directories to search for class files. The autoload function iterates through each directory, checking if the file exists before including it. This allows you to keep your classes organized in separate folders without modifying the autoloading logic each time you add a new directory.
This method provides a robust solution for handling class files in various directories, making your application more modular and maintainable.
Conclusion
Autoloading classes in PHP using the spl_autoload_register
function is an efficient way to manage your codebase. By implementing autoloading, you streamline your workflow, reduce the risk of errors, and keep your code organized. Whether you are using simple class names or sophisticated namespaces, the techniques outlined in this article will help you create a more maintainable and scalable PHP application. Embrace autoloading, and watch your development process become more efficient and enjoyable.
FAQ
-
What is the purpose of spl_autoload_register in PHP?
spl_autoload_register allows you to automatically load class files without manually including them, streamlining the development process. -
Can I register multiple autoload functions?
Yes, you can register multiple autoload functions using spl_autoload_register, allowing for flexibility in how classes are loaded. -
How do namespaces affect autoloading in PHP?
Namespaces help organize classes and prevent name collisions, and they can be integrated into the autoloading mechanism to load classes from specific directories. -
Is autoloading suitable for large applications?
Yes, autoloading is particularly beneficial for large applications as it simplifies file management and enhances code organization. -
Can I use autoloading with third-party libraries?
Absolutely! Autoloading can be used with third-party libraries, especially if they follow a standard structure, making integration easier.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn