How to Print to Console in PHP
-
Use the JavaScript
console.log()
in PHP to Write to the Console -
Use JavaScript
console.log()
and thejson_encode()
Function to Write to the Console in PHP
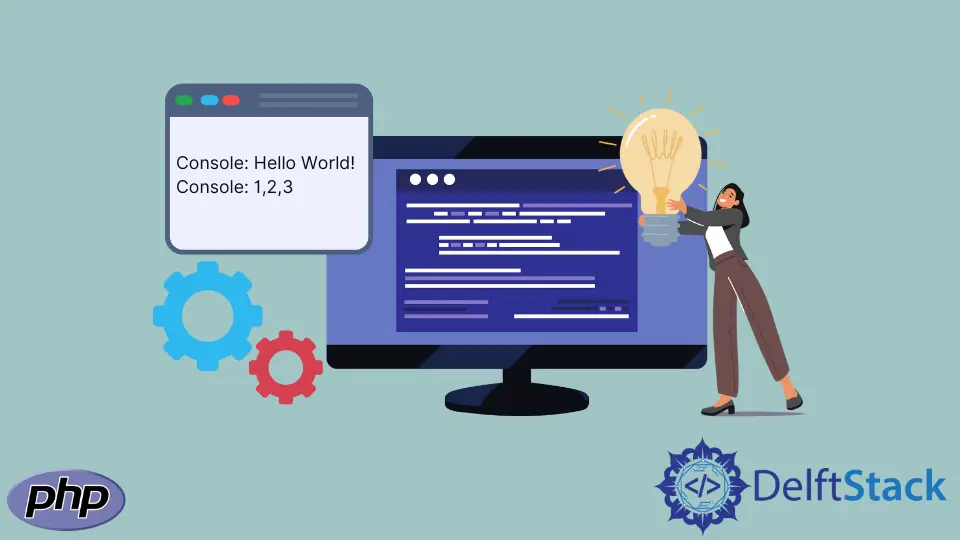
This tutorial introduces how to write to the console in PHP.
Use the JavaScript console.log()
in PHP to Write to the Console
We can use some JavaScript in PHP to write to the console. We use console.log()
in JavaScript to write anything to the console. We can use the echo
statement to print the console.log()
from JavaScript in PHP. If we use a PHP variable as the argument to console.log()
then, the content of the variable will be written to the console. We can use the is_array()
function to check if the variable to be printed is an array. If the element is an array, we can use the implode()
function with ,
as the separator to concatenate the array elements to a string.
For example, create a function write_to_console()
that takes a parameter $data
. Store the $data
variable in a new variable $console
. Check if the variable $console
is an array with the is_array()
function. If the variable is an array, then concatenate the array elements using the implode()
function using the ,
as the separator. Store the value at the $console
variable. Then, inside the script
tag, use console.log()
to log the $console
variable. Then use the echo
statement to print the JavaScript code. Outside the function, call the write_to_console()
function with two different parameters. Supply the string Hello World!
as the first parameter and an array [1,2,3]
as the second parameter.
When we go to the webpage and check the console from the Inspect Element
option, we can see the data written in the console. In this way, we can use a helper function to write to the console in PHP.
Example Code:
<?php
function write_to_console($data) {
$console = $data;
if (is_array($console))
$console = implode(',', $console);
echo "<script>console.log('Console: " . $console . "' );</script>";
}
write_to_console("Hello World!");
write_to_console([1,2,3]);
?>
Output:
Console: Hello World!
Console: 1,2,3
Use JavaScript console.log()
and the json_encode()
Function to Write to the Console in PHP
We can use the json_encode()
function along with the JavaScript console.log()
to write to the console in PHP. The json_ecode()
function converts the given associative array into a JSON object and indexed array into a JSON array. We can use the function on those data items that need to be written to the console.
For example, create a function write_to_console()
with a parameter $data
. Inside the function, apply the json_encode()
function on the $data
variable and log it with console.log
. Make this whole expression a string and save it in the $console
variable. Then, print the variable using the sprintf()
function as sprintf('<script>%s</script>', $console);
and assign the expression to $console
. Finally, print the $console
variable with the echo
statement. Outside the function, create an associative array on a variable $data
and indexed array on a variable $days
. Then, call the write_to_console()
function with these two variables as parameters.
The associative array is written as a JSON object, and the indexed array is written as a JSON array in the console. This is how we can use the json_encode()
function to write to the console in PHP.
Example Code:
<?php
function write_to_console($data) {
$console = 'console.log(' . json_encode($data) . ');';
$console = sprintf('<script>%s</script>', $console);
echo $console;
}
$data = [ 'foo' => 'bar' ];
$days = array("Sun", "Mon", "Tue");
write_to_console($data);
write_to_console($days);
?>
Output:
Object { foo: "bar" }
Array(3) [ "Sun", "Mon", "Tue" ]
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn