How to Validate Phone Number in PHP
- Use Regular Expressions to Validate Phone Number in PHP
-
Use the
libphonenumber-for-php
Library to Validate Phone Number in PHP - Check the Digits and Length to Validate Phone Number in PHP
-
Use the
filter_var()
Function to Validate Phone Number in PHP - Check Country Code to Validate Phone Number in PHP
- Conclusion
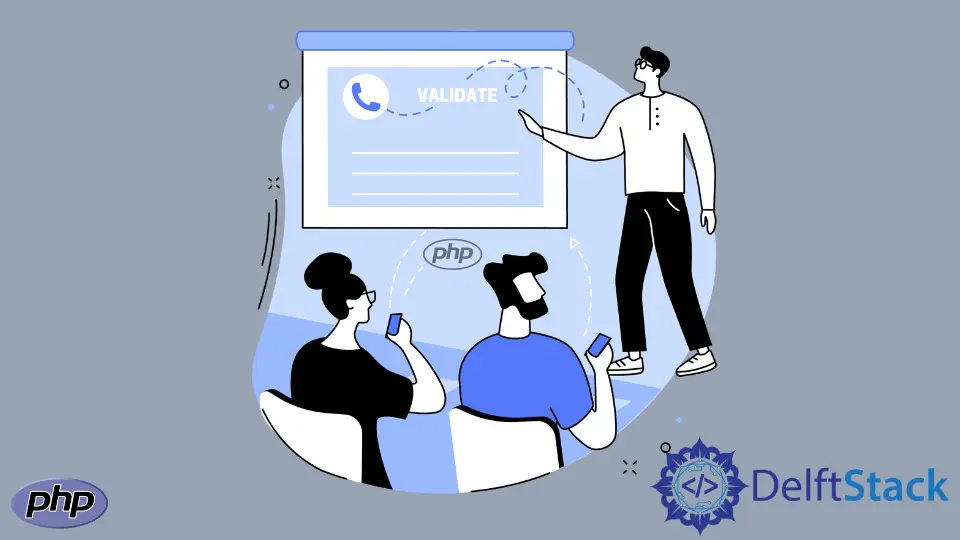
Phone number validation in PHP is a crucial task, especially when dealing with user input or integrating phone number functionality in your applications. Validating phone numbers can help ensure data integrity and improve user experience.
In this article, we will discuss various methods and techniques for validating phone numbers in PHP, along with code examples and explanations.
Use Regular Expressions to Validate Phone Number in PHP
Phone numbers come in various formats and can be challenging to validate using simple string comparisons. With regex, you can create custom patterns to match specific phone number formats, making your validation process more robust.
A regular expression is a sequence of characters that defines a search pattern.
In PHP, you can use the preg_match()
function to apply a regex pattern to a string, determining whether the string matches the pattern. Its syntax is as follows:
preg_match(pattern, subject, matches, flags, offset)
Parameters:
pattern
: This parameter specifies the regular expression pattern you want to match against thesubject
string.subject
: This is the string you want to search for a match within using the regular expression.matches
(optional): An array where the matched results will be stored. If provided,matches
will be populated with the matched substrings based on capturing groups in the regular expression.flags
(optional): This parameter allows you to specify optional flags that modify the behavior of thepreg_match()
function. Some common flags include:PREG_OFFSET_CAPTURE
: If set,matches
will contain the matched substrings along with their starting positions in thesubject
string.PREG_PATTERN_ORDER
: If set,matches
will be a two-dimensional array containing matched patterns and their corresponding matches.PREG_SET_ORDER
: If set,matches
will be a one-dimensional array containing an array for each match.
offset
(optional): The optionaloffset
parameter allows you to specify a starting point in thesubject
string from which the search should begin.
The preg_match()
function returns 1
if a match is found, 0
if no match is found, or FALSE
on error.
When validating phone numbers, it’s essential to understand the components of the regex pattern.
Let’s break down the regex pattern used to validate a 10-digit US phone number in the format 123-456-7890
:
$pattern = "/^\d{3}-\d{3}-\d{4}$/";
In this pattern:
/ |
The forward slashes delimit the regex pattern. They indicate the beginning and end of the pattern. |
^ |
The caret symbol asserts the start of the string. |
\d |
This represents a digit (0-9). It’s a shorthand character class for any numeric character. |
{n} |
The curly braces indicate repetition. In this case, \d{3} specifies that exactly three digits are expected. |
- |
Hyphens are included in the pattern to match the hyphens in the phone number. |
$ |
The dollar sign asserts the end of the string. |
Customizing the regex pattern allows you to adapt the validation to different phone number formats. For example, if you want to validate phone numbers without hyphens, you can modify the pattern as follows:
$pattern = "/^\d{10}$/";
In this pattern, we are matching exactly 10 digits without any hyphens. You can adjust the regex pattern according to the specific phone number format you want to validate.
While the previous example focused on a specific US phone number format, regex can also handle international phone numbers. To do this, you’ll need to create more complex patterns that consider various international formats and country codes.
You can use alternation (the |
operator) to account for multiple patterns.
Here’s a simplified example for validating international phone numbers with country codes:
$pattern = "/^(\+\d{1,4}-)?\d{5,15}$/";
In this pattern, (\+\d{1,4}-)?
matches an optional plus sign followed by one to four digits and a hyphen (for the country code). Then, \d{5,15}
matches five to fifteen digits for the phone number itself.
This pattern allows for international phone numbers with or without a country code.
Here’s the complete code example for validating a 10-digit US phone number using regular expressions:
<?php
$phone_number = '123-456-7890';
$pattern = "/^\d{3}-\d{3}-\d{4}$/";
if (preg_match($pattern, $phone_number)) {
echo "Valid US phone number: $phone_number";
} else {
echo "Invalid phone number";
}
?>
Output:
Valid US phone number: 123-456-7890
In this code example, we start by defining the $phone_number
variable with the value '123-456-7890'
, which represents a 10-digit US phone number in the format 123-456-7890
. We then create a regular expression pattern in the $pattern
variable, "/^\d{3}-\d{3}-\d{4}$/"
, which checks for the exact format we’re expecting.
The preg_match()
function is used to apply the regular expression pattern to the phone number string. If the pattern matches, it means the phone number is valid, and the script outputs Valid US phone number
along with the phone number itself; otherwise, it outputs an Invalid phone number
.
In this case, the output indicates that the provided phone number, 123-456-7890
, is a valid US phone number.
Use the libphonenumber-for-php
Library to Validate Phone Number in PHP
Phone number validation becomes more complex when dealing with international phone numbers, as they can have varying formats and country-specific rules. Fortunately, there are specialized PHP libraries, such as libphonenumber-for-php
, that provide comprehensive solutions for phone number validation, especially when handling international formats.
Before using the libphonenumber-for-php
library, you need to install it. You can download the library’s Phar (PHP Archive) file from the GitHub repository or use Composer to manage the package.
For this example, we’ll assume you’ve already installed the library and have the Phar file in your project directory.
Here’s how to use the libphonenumber-for-php
library to validate a phone number:
require 'libphonenumber-for-php/libphonenumber.phar';
$phone_number = '1234567890';
$phoneNumberUtil = \libphonenumber\PhoneNumberUtil::getInstance();
try {
$phoneNumber = $phoneNumberUtil->parse($phone_number, null);
if ($phoneNumberUtil->isValidNumber($phoneNumber)) {
echo "Valid phone number: $phone_number";
} else {
echo "Invalid phone number";
}
} catch (\libphonenumber\NumberFormatException $e) {
echo "Invalid phone number";
}
In this code, we begin by including the libphonenumber-for-php
library using the require
statement. The variable $phone_number
is set to the phone number that you want to validate. In this example, it’s set to 1234567890
.
We create a PhoneNumberUtil
instance using $phoneNumberUtil = \libphonenumber\PhoneNumberUtil::getInstance();
. This instance will be used to parse and validate the phone number.
Inside a try-catch
block, we attempt to parse the phone number using $phoneNumberUtil->parse($phone_number, null);
. The parse
method is responsible for interpreting the phone number.
Next, we use $phoneNumberUtil->isValidNumber($phoneNumber)
to check if the phone number is valid according to the library’s rules. If it’s a valid phone number, we display Valid phone number
, and if not, we display Invalid phone number
.
In the catch
block, we handle exceptions, particularly the libphonenumber\NumberFormatException
that may be thrown if there’s an issue with the phone number.
When you run the provided code with the phone number 1234567890
, you should see the following output:
Valid phone number: 1234567890
The library successfully validates the phone number as valid based on its internal rules and patterns. This example showcases how the libphonenumber-for-php
library can handle international phone number validation with ease, making it a valuable tool for applications dealing with diverse phone number formats from around the world.
Check the Digits and Length to Validate Phone Number in PHP
One of the most common and straightforward methods for phone number validation is by checking if the phone number consists of digits and is of the correct length.
To validate phone numbers by checking if they consist of digits and are of the correct length, first, you need to obtain the user’s input, typically through an HTML form or from other sources in your PHP application. Then, you can use a combination of functions to validate the phone number.
Here’s a code example that validates a US phone number with 10 digits:
<?php
function validatePhoneNumber($phoneNumber) {
if (ctype_digit($phoneNumber) && strlen($phoneNumber) == 10) {
return true; // Valid phone number
} else {
return false; // Invalid phone number
}
}
$phoneNumber = "1234567890"; // Replace with the user's input
if (validatePhoneNumber($phoneNumber)) {
echo "Valid phone number: $phoneNumber";
} else {
echo "Invalid phone number";
}
?>
Here, we define a function called validatePhoneNumber
that takes the user’s input, $phoneNumber
, as its parameter.
Inside the function, we use ctype_digit($phoneNumber)
to check if the phone number consists of digits only. We also use strlen($phoneNumber) == 10
to verify that the phone number has the expected length of 10 digits.
If both conditions are met, the function returns true
, indicating a valid phone number. Otherwise, it returns false
for an invalid phone number.
In the main part of the script, we obtain the user’s input (in this case, 1234567890
) and call the validatePhoneNumber
function to check its validity. Depending on the result, we display Valid phone number
or Invalid phone number
.
If you run the code with the provided phone number 1234567890
, you will see the following output:
Valid phone number: 1234567890
Validating phone numbers in PHP by checking digits and length is a straightforward and effective method to ensure that the provided phone numbers are correctly formatted. This method is suitable for scenarios where you expect a specific format and length for phone numbers, such as national phone numbers in a given country.
Keep in mind that international phone numbers may have varying formats and lengths, and you might need to employ more advanced validation techniques for such cases.
Use the filter_var()
Function to Validate Phone Number in PHP
In PHP, filter_var()
serves as another valuable tool for validating and filtering user inputs based on specific filters. It checks if a variable is of a specified filter type, which includes validation, sanitization, and data type verification.
To validate phone numbers using filter_var()
, we can apply the FILTER_VALIDATE_INT
filter along with a length check. This process can help ascertain if the provided input meets the criteria of a valid phone number, such as having a certain number of digits.
Let’s have an example:
$phone_number = '1234567890';
if (filter_var($phone_number, FILTER_VALIDATE_INT) && strlen($phone_number) == 10) {
echo "Valid US phone number: $phone_number";
} else {
echo "Invalid phone number";
}
In this code snippet, we are validating a phone number represented as a string in the variable $phone_number
.
The filter_var($phone_number, FILTER_VALIDATE_INT)
is the first check. Here, we are using the FILTER_VALIDATE_INT
filter to ensure that the input can be parsed as an integer.
This check will return true
if the string consists of digits only, with an optional negative sign at the beginning.
Then, strlen($phone_number) == 10
is the second condition. This checks whether the length of the phone number is exactly 10 characters.
In this context, we assume that a valid US phone number should have ten digits.
If both conditions are met, the script will output Valid US phone number
followed by the actual phone number. Otherwise, it will output Invalid phone number
.
The example provided in the code snippet validates the phone number 1234567890
and produces the following output:
Valid US phone number: 1234567890
This shows that the input phone number is considered valid according to the specified criteria.
It’s important to note that this approach is a simple validation method. Depending on your application’s requirements, you might need to implement more robust phone number validation, considering international phone number formats, country-specific rules, and number formatting variations.
For instance, real-world phone numbers may include dashes, spaces, parentheses, or other characters. In such cases, you may want to remove these characters before applying validation.
Additionally, consider using external libraries or services, such as the libphonenumber
library discussed above, for comprehensive phone number validation and formatting, especially when dealing with phone numbers from various countries.
Check Country Code to Validate Phone Number in PHP
The country code is an essential prefix used in international phone numbers to designate the country to which a particular number belongs. Each country has its unique country code, enabling callers to identify the location of the phone number they are dialing.
Validating phone numbers based on their country code is a crucial aspect of ensuring the accuracy and legitimacy of the provided information. By checking if a phone number begins with the expected country code, you can confirm its association with a specific geographic location.
To illustrate the process of validating a phone number based on the expected country code, here’s an expanded example focusing on different country codes:
PHP Code Example:
$phone_number_1 = '+14155552671'; // US country code: +1
$phone_number_2 = '+447700900000'; // UK country code: +44
$phone_number_3 = '+618123456789'; // Australia country code: +61
$expected_country_code_us = '+1'; // US country code
$expected_country_code_uk = '+44'; // UK country code
$expected_country_code_australia = '+61'; // Australia country code
function validatePhoneNumber($phone_number, $expected_country_code) {
if (strpos($phone_number, $expected_country_code) === 0) {
echo "Valid phone number ($expected_country_code): $phone_number\n";
} else {
echo "Invalid phone number: $phone_number\n";
}
}
validatePhoneNumber($phone_number_1, $expected_country_code_us);
validatePhoneNumber($phone_number_2, $expected_country_code_uk);
validatePhoneNumber($phone_number_3, $expected_country_code_australia);
In the code, we first define sample phone numbers and their respective expected country codes. These variables serve as the data we want to validate.
These variables represent phone numbers from the United States, the United Kingdom, and Australia, along with their respective expected country codes.
Next, we define a custom function called validatePhoneNumber
, designed to check whether a given phone number starts with the expected country code.
This function takes two parameters: $phone_number
and $expected_country_code
. $phone_number
is the phone number to be validated and $expected_country_code
is the expected country code for the validation.
Inside the function, we use the strpos()
function to check if the $expected_country_code
can be found at the very beginning of the $phone_number
.
If the strpos()
function returns 0
, it means that the expected country code is found at the start of the phone number, indicating a valid phone number. If it doesn’t return 0
, the phone number is considered invalid.
After defining the validatePhoneNumber
function, we proceed to validate each sample phone number against its corresponding expected country code.
In the last part of the code, we call the validatePhoneNumber
function for each of the sample phone numbers. It checks each phone number against its expected country code and outputs the result.
Upon running the code, you will receive the following validation results:
Valid phone number (+1): +14155552671
Valid phone number (+44): +447700900000
Valid phone number (+61): +618123456789
Validating phone numbers based on their country codes is a foundational step in ensuring data accuracy and integrity. By confirming that a phone number begins with the expected country code, businesses and systems can accurately process and categorize phone numbers according to their geographic origin, contributing to better data management and communication practices.
Conclusion
Phone number validation is essential in PHP applications to ensure that user input adheres to expected formats and rules. You can choose from various methods, such as regular expressions, phone number libraries, digit and length validation, or country code validation, depending on your specific use case.
Keep in mind that phone number validation can be complex due to international variations. Choose the method that best suits your application’s needs, and be aware of international phone number formats and local regulations when implementing phone number validation.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook