URL Encoding in PHP
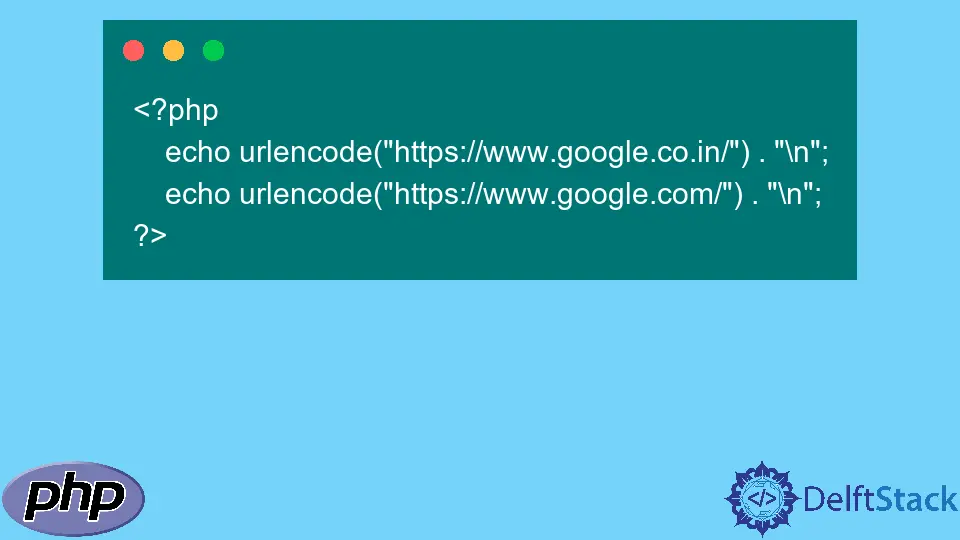
URLs may have path and query parameters like someone’s full name, another redirect URL or a password. These can contain special characters outside the ASCII set, like spaces or characters like $ & : /
.
Therefore the URL should be regenerated into a legitimate ASCII format before being transmitted over the Internet; otherwise, they can interfere with the HTTP protocol.
In today’s post, we will learn how to encode URLs in PHP.
PHP provides 2 functions to encode the URL.
Use urlencode()
to Encode the URL in PHP
It is a built-in function provided by PHP that is used to encode the URL. This function replaces unsafe ASCII characters with a %
followed by 2 hexadecimal digits. This function encodes according to application/x-www-form-urlencoded
. A URL cannot contain spaces, so that this function will replace space with a plus +
sign. Special characters are regenerated in an exceedingly specific format based on some predefined rules.
Syntax for urlencode()
urlencode(string $input);
Parameters
$input
: This is a mandatory parameter, which takes only the string input URL on which encoding is performed.
Return Value
It returns a string that contains all non-alphanumeric characters except -_.
, which are replaced by the %
sign and followed by 2 hex digits.
Example code:
<?php
echo urlencode("https://www.google.co.in/") . "\n";
echo urlencode("https://www.google.com/") . "\n";
?>
Output:
https%3A%2F%2Fwww.google.co.in%2F
https%3A%2F%2Fwww.google.com%2F
Use rawurlencode()
to Encode URL in PHP
It is a built-in function provided by PHP that can encode a given URL(Uniform Resource Locator) string according to RFC 3986. It encodes according to the plain Percent-Encoding. It’s used to prevent literal characters from being interpreted as special URL delimiters and to prevent URLs from being mangled by transmission media with character conversions (like some email systems).
The symbols or space character will be replaced with a percent (%) sign followed by 2 hex digits.
Syntax for rawurlencode()
rawurlencode(string $input);
Parameters
$input
: It is a mandatory parameter that takes only the string input URL on which encoding is performed.
Return Value
It returns an encoded string that contains all non-alphanumeric characters except -_.~
symbols.
Example code:
<?php
echo '<a href="http://testdomain.com/', rawurlencode('subscribers and admins/India'), '">';
?>
Output:
<a href="http://testdomain.com/subscribers%20and%20admins%2FIndia">
The sole distinction between urlencode()
and rawurlencode()
functions is that first encodes space as +
while later encodes as %20
. Also ~
is encoded in urlencode()
but not in rawurlencode()
. If you want to encode query component, use urlencode()
and if you want to encode path segment, use rawurlencode()
.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn