How to Use Default in PHP Switch Case
-
PHP
switch
Case -
Use the
default
Statement in theswitch-case
Statement in PHP -
Use the
default
Statement Without thebreak
Statement in PHPswitch-case
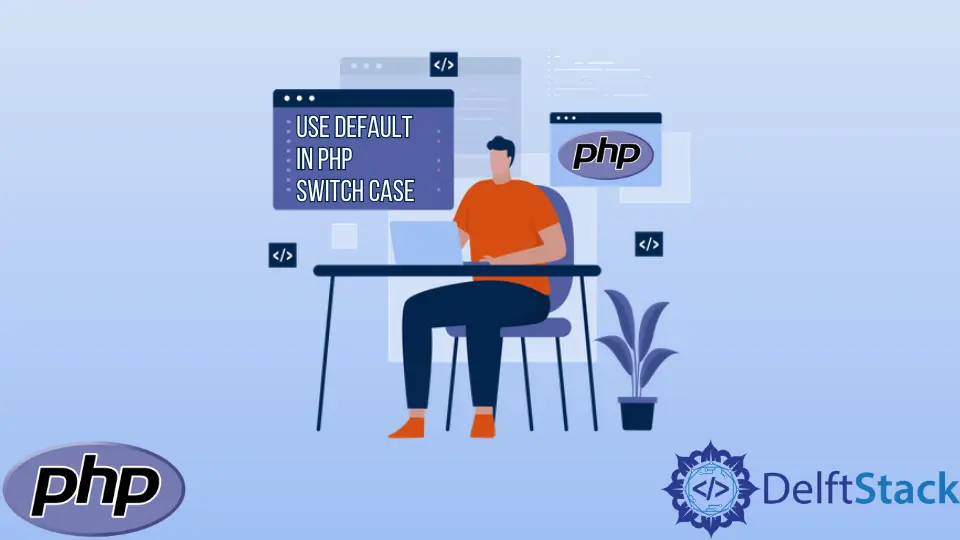
We will introduce the switch case statements in PHP. We will describe the different scenarios of switch cases and how the code handles the cases. We will then introduce the break
statement and its use in the switch case statement in PHP.
We will check if the default
case executes if there exists a matching case before it. In this method, we will remove the break
statement to check the result.
PHP switch
Case
The switch-case
statement is conditional and an alternative to the if-elseif-else
statement. The statement checks a variable for several cases until it finds the correct match and executes it according to the matched case. We can use the switch
statement to test the variable and use the case
statement to specify the case to be tested. We write the piece of code after the case statement to execute the code if the case matches.
The switch-case
statement differs from the if-elseif-else
statement in one distinctive way. The if-elseif-else
statement executes the only code after the condition is true and aborts the conditional test. But, in the switch case statement, every case is tested, and each corresponding code executes. To get rid of the problem, we use the break
statement. When the case matches and the corresponding codes run, the execution comes to the break
statement, and the conditional check aborts. Therefore, we write the break
statement at the end of each case.
For example, create a variable $favfood
and assign it with the value pizza
. Write a switch
statement taking the variable $favfood
in the parenthesis. Inside the switch statement, write the case
statement and provide the case momo
as case "momo":
. Do not miss the colon after the value. Use the echo
statement below the case and display the message Your favorite food is momo!
. Write the break
statement after displaying the message. Similarly, write the cases for spaghetti
and pizza
as you did for momo
, display the message accordingly, and write the break
statement for each of the cases.
The example below displays the message Your favorite food is pizza!
because the variable $favfood
holds the value pizza
. Firstly, the case momo
is tested. Since it does not match, the execution moves towards the case spaghetti
. This case also does not match, but the case pizza
matches. Then it displays the corresponding message and executes the break
statement. The break
statement ends the whole switch case statement preventing the further code from being executed.
Example Code:
# php 7.*
<?php
$favfood = "pizza";
switch ($favfood) {
case "momo":
echo "Your favorite food is momo!";
break;
case "spaghetti":
echo "Your favorite food is spaghetti!";
break;
case "pizza":
echo "Your favorite food is pizza!";
break;
case "burger":
echo "Your favorite food is burger!";
break;
}
?>
Output:
Your favorite color is pizza!
Use the default
Statement in the switch-case
Statement in PHP
We can use the default
statement in the switch-case
statement to denote the cases that do not meet the above-specified cases. In other words, the default
statement will execute if none of the cases matches. We write the default
statement at the end of all the cases. In the above example, there is no default case. If none of the mentioned cases match, the code outputs nothing. Therefore, the default
statement addresses the rest of the cases.
We can modify the first code example to illustrate the use of the default
statement. For instance, assign the value spaghetti
to the variable $favfood
. Remove the code blocks of the case spaghetti
and add a default statement. Inside the default
statement, display the message We could not find your favorite food
. Write the break
statement after the message.
In the example below, none of the specified cases match. So, the control reaches the default
statement. Then, it displays the corresponding message. If we had not removed the code blocks of the spaghetti
, the default statement would not get executed. It would show the message saying Your favorite food is spaghetti!
. The break
statement would abort the conditional check if the case matched.
Example Code:
#php 7.x
<?php
$favfood = "spaghetti";
switch ($favfood) {
case "momo":
echo "Your favorite food is momo!";
break;
case "pizza":
echo "Your favorite food is pizza!";
break;
case "burger":
echo "Your favorite food is burger!";
break;
default:
echo "We could not find your favorite food";
break;
}
?>
Output:
We could not find your favorite food
Use the default
Statement Without the break
Statement in PHP switch-case
We can use the default
statement in the switch case without using the break
statement to check whether the default
block will execute a matching case before it. We can slightly modify the example code above for the demonstration. For example, assign the $favfood
to the value of momo
. Write the cases and code blocks for momo
, pizza
, burger
, and default
, respectively. Do not write the break
statement in any of the code blocks.
In the example below, the execution goes through all the cases and displays all the messages. Even if there are the matching cases before, the default block will be executed along with the blocks followed by the matching case. This is because we omitted the break
statement.
Example Code:
#php 7.x
<?php
$favfood = "momo";
switch ($favfood) {
case "momo":
echo "Your favorite food is momo!"."<br>";
case "pizza":
echo "Your favorite food is pizza!"."<br>";
case "burger":
echo "Your favorite food is burger!"."<br>";
default:
echo "We could not find your favorite food"."<br>";
}
?>
Output:
Your favorite food is momo!
Your favorite food is pizza!
Your favorite food is burger!
We could not find your favorite food.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn