How to Return to Previous Page in PHP
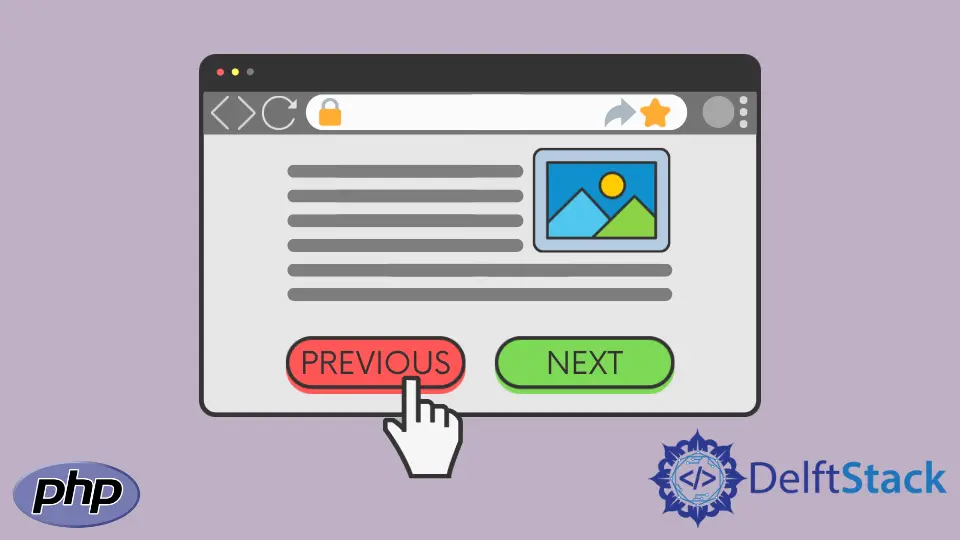
This article will introduce some methods to return to the previous page in PHP.
Use the HTTP_REFERER
Request Header to Return to the Previous Page in PHP
The HTTP_REFERER
request header returns the URL of the page from where the current page was requested in PHP. The header enables the server to acknowledge the location from where the users are visiting the current page. The header is used as the index of the $_SERVER
array. We can use the header()
function with the location
header to redirect the current page to the previous page. We should set the location
to $SERVER['HTTP_REFERER']
to return to the previous page.
Let’s see how the HTTP_REFERER
header works. For instance, create a button in HTML. Set the action
attribute to home.php
and the method
attribute to post
. Save the file as index.php
. In the home.php
file, check whether the form is submitted with the isset()
function. Then, use the echo
function to display the $_SERVER[HTTP_REFERER]
header.
Example Code:
<form action ="home.php" method = "POST">
<button type="submit" name="button"> Submit</button>
</form>
if(isset($_POST['button'])){
echo $_SERVER[HTTP_REFERER];
}
Output:
http://localhost/index.php
Here, we created the form in the index.php
file. Then, the form is submitted to the home.php
file. It means that the home.php
page was requested from the index.php
page. Therefore, the index.php
page is the referrer. The output section above shows that the HTTP_REFERER
returns the URL http://localhost/index.php
, the referrer.
Our goal is to redirect the current page home.php
to the previous page index.php
.
For example, in the home.php
file, create a variable $message
to store the message to be displayed after the redirection occurs. Use the urlencode()
to write the message in its parameter. Next, write the header()
function to set the location of the redirection. Concatenate $_SERVER[HTTP_REFERER]
and "?message=".$message
and set it the value for location
in the header()
function. Next, call the die
function. In the index.php
file, print the $_GET['message']
variable right below the form using the echo
function.
Here, we used the urlencode()
function to write the message because the message is a string that is queried in the URL. The message
index in the $_GET
array is the variable we used in the URL referring to the previous page in the header()
function.
When we click the button on the index.php
page, the form gets submitted to home.php
and will redirect back to the index.php
page, the previous page.
In this way, we can use the header()
function and the HTTP_REFERER
header to return the current page to the previous page in PHP.
Example Code:
//index.php
<form action ="home.php" method = "POST">
<button type="submit" name="button"> Submit</button>
</form>
<?php
if(isset($_GET['message'])){
echo $_GET['message'];
}
?>
//home.php
if(isset($_POST['button'])){
$message = urlencode("After clicking the button, the form will submit to home.php. When, the page home.php loads, the previous page index.php is redirected. ");
header("Location:".$_SERVER[HTTP_REFERER]."?message=".$message);
die;
}
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn