How to Read Text Files in PHP
-
Use the
fgets()
Function to Read Text Files Line by Line in PHP -
Use the
file()
Function to Read Text Files Line by Line in PHP -
Use the
file_get_contents()
andexplode()
Functions to Read File Line by Line in PHP
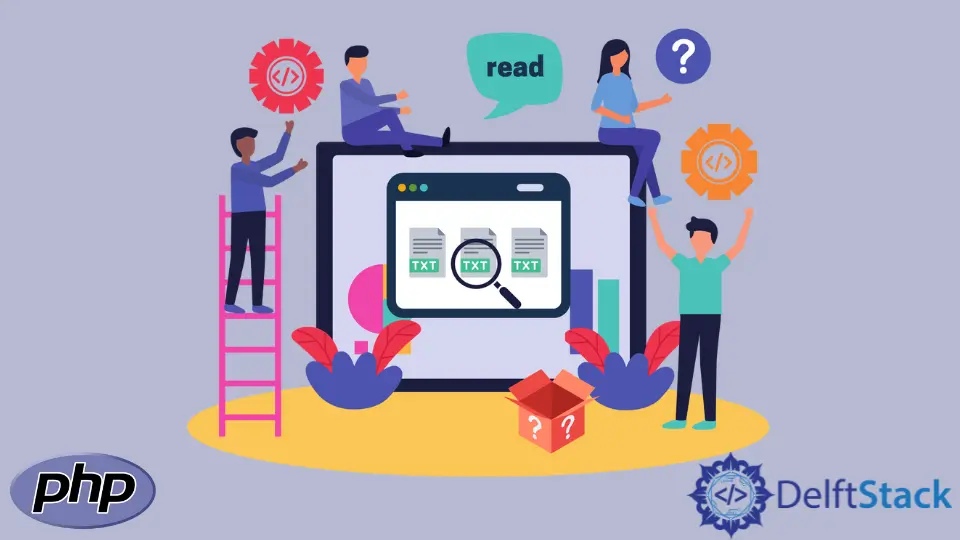
This article will introduce methods to read text files line by line in PHP.
Use the fgets()
Function to Read Text Files Line by Line in PHP
We can use the fgets()
function with a while
loop to read text files line by line in PHP. The function returns a line if there is a line, and it returns false if there are no more lines to read. It takes two parameters. The syntax is as the following.
fgets($file, $length)
Here, $file
resembles a file pointer that points to a successfully opened file. The $length
option, optional, denotes the number of bytes to be read.
We can use the open()
function to read the file and then use the while
loop to loop through each line with the fgets()
function. We have a text file abc.txt
containing the following contents.
Hi
How are you
Have a great day
For example, create a variable $txt_file
and write the fopen()
function in it. Open the file abc.txt
in r
mode. Create a line counter variable $a
and assign the value 1
to it. Then, create a while
loop. Inside the parenthesis of the loop, write the fgets()
function with $text_file
as the parameter. Assign the function to a $line
variable in the loop. Print the $line
variable, concatenating it with the $a
variable inside the loop body. Increment the $a
variable and outside the loop, use the fclose()
function to close the file stream.
Example Code:
<?php
$txt_file = fopen('abc.txt','r');
$a = 1;
while ($line = fgets($txt_file)) {
echo($a." ".$line)."<br>";
$a++;
}
fclose($txt_file);
?>
Output:
1 Hi
2 How are you
3 Have a great day
Use the file()
Function to Read Text Files Line by Line in PHP
The file()
function reads the whole file into an array. The syntax of the file()
function is as below.
file($filename, $flag, $context)
Here, $filename
is the file path to be read. The $flag
option is optional and it comprises of various constants such as FILE_USE_INCLUDE_PATH
, FILE_IGNORE_NEW_LINES
and FILE_SKIP_EMPTY_LINES
. The third one is also optional, which defines a context resource.
The file()
function returns the whole array if it exists or returns false. We can use the function to read the file context line by line with the helo of the foreach()
function. The foreach()
function will loop through the entire contents and extract each line individually.
For example, store the filepath in a variable $txt_file
. Write a variable $lines
and store the file()
function on it with $txt_file
as the parameter. Next, use the foreach()
function to loop through the contents of the file. Use $lines
as iterator and $num=>$line
as the value. Inside the loop body, print the $num
and $line
variables.
Example Code:
$txt_file = 'abc.txt';
$lines = file($txt_file);
foreach ($lines as $num=>$line)
{
echo 'Line '.$num.': '.$line.'<br/>';
}
Output:
Line 0: Hi
Line 1: How are you
Line 2: Have a great day
Use the file_get_contents()
and explode()
Functions to Read File Line by Line in PHP
The file_get_contents()
function reads the whole file into a string. It returns the whole file as a string if the contents exist or it returns false. We can specify the file path as the parameter to the function. The explode()
function splits a string into an array using a separator. The syntax of the explode()
function is as the following.
explode(separator, $string, $limit)
The separator
option is used to separate $string
with the number of $limit
elements while returning the value. We can combine the file_get_contents()
, explode()
and foreach()
functions to read the text file line by line in PHP.
For example, create a variable $contents
and write the file_get_contents()
function on it with the filepath in the parameter. Use the explode()
function with the \n
character as a separator and $contents
as the string. Assign the function to a variable $lines
. Use the foreach
loop to loop $lines
as $line
. Then, print the $line
inside the loop body.
In the example below, the $contents
variable returns string. We split it into an array with the \n
newline character and printed each line using the loop.
Example Code:
$contents=file_get_contents("abc.txt");
$lines=explode("\n",$contents);
foreach($lines as $line){
echo $line.'<br>';
}
Output:
Hi
How are you
Have a great day
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn