How to Send POST Request in PHP
-
Use the CURL-less Method Using the
stream_context_create()
andfile_get_contents()
Functions to Send a POST Request in PHP - Use the CURL to Send the POST Request in PHP
- Create a User-Defined Function That Uses the CURL to Send the POST Request in PHP
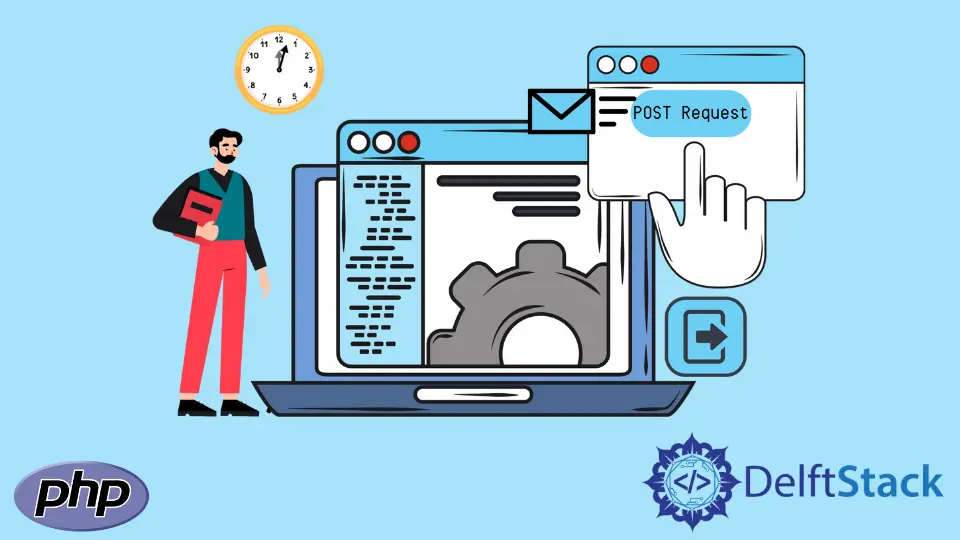
We will introduce a method to send a POST request in PHP using the CURL-less method with the use of the http_build_query()
, stream_context_create()
and file_get_contents()
functions.
We will show you a method to send the POST request in PHP using the CURL. CURL is used to send the HTTP request to the webserver. The CURL module is generally pre-installed with PHP. If it has not been installed, we should install it manually to send the server’s request using PHP.
We will show you an example to send the POST request in PHP using the CURL by creating a function. This method is very much similar to the second method. It uses a user-defined function to send the request. We can reuse the code supplying the different post data and the URL.
Use the CURL-less Method Using the stream_context_create()
and file_get_contents()
Functions to Send a POST Request in PHP
We can send the POST request in PHP by using the functions like http_build_query()
, stream_context_create()
and file_get_contents()
functions without using the CURL. We can use the http_build_query()
function to create query parameters to send in the POST request. We can create an array to specify the http headers, methods and the contents. We use the stream_context_create()
function to handle the streamed data. The file_get_contents()
function reads the content of the url into string. We use the $_POST
global variables to read the data.
For example, create the http_build_query()
function and create an array inside the function. Create the keys name
and id
and the values Robert
and 1
respectively in the array. Assign the function with a variable $postdata
. Create an array to specify the HTTP method, header, and content. Set the values for the keys method
, header
and the content
as POST
, Content-type: application/x-www-form-urlencoded
and the variable $postdata
. Wrap this array inside another array http
. Assign the array to a variable $opts
. Take the $opts
as a parameter to the stream_context_create()
function and assign it to a variable $context
. Use the file_get_contents()
function and apply a URL http://localhost/request.php
as the first parameter. Use the boolean value false
and the variable $context
as the second and the third parameters. Assign the function to a variable $result
and print it. Create a PHP file request.php
and echo the name
and id
keys using the $_POST
variable.
In the example below, name
and id
are the data to be posted using the POST method. The request.php
file reads the query parameters using the $_POST
variable.
Example Code:
# php 7.*
<?php
$postdata = http_build_query(
array(
'name' => 'Robert',
'id' => '1'
)
);
$opts = array('http' =>
array(
'method' => 'POST',
'header' => 'Content-type: application/x-www-form-urlencoded',
'content' => $postdata
)
);
$context = stream_context_create($opts);
$result = file_get_contents('http://localhost/request.php', false, $context);
echo $result;
?>
# php 7.*
<?php
echo "Name: ". $_POST['name']. "<br>";
echo "ID: ". $_POST['id'];
?>
Output:
Name: Robert
ID: 1
Use the CURL to Send the POST Request in PHP
CURL stands for Client URL. We can use the CURL to send the POST request through the PHP script. The PHP version needs the curl module to execute the different curl functions. In this method, we use the http_build_query()
function as in the method above to make the query parameters. We can use the curl_init()
function to initialize the CURL connection in the script. We use the curl_setopt()
functions with some options like CURLOPT_URL
, CURLOPT_POST
and CURLOPT_POSTFIELDS
. These options set the URL, request an HTTP POST request, and POST the data, respectively. We can use the curl_setopt()
function to return the contents of the URL and use the curl_exec()
function to execute the POST request.
For example, create the keys name
and id
and the values Wayne
and 2
in an array $fields
. Use the array as the parameter in the http_build_query()
function and assign the function with a variable $postdata
. Open a CURL connection using the curl_init()
function and assign it to a variable $ch
. Write three curl_setopt()
functions and use the $ch
variable as the first parameter in all the three functions. As the second parameter in the functions, write the options CURLOPT_URL
, CURLOPT_POST
, and CURLOPT_POSTFIELDS
in the first, the second, and the third functions, respectively. Set the third parameter as http://localhost/request.php
, true
and $postdata
respectively in the three functions. Call a function curl_setopt()
and use the $ch
variable as the first parameter, the CURLOPT_RETURNTRANSFER
option as the second parameter, and the boolean value true
as the third parameter. Call the curl_exec($ch)
function from the $result
variable and print the $result
. Use the same request.php
file of the first method.
Example Code:
#php 7.x
<?php
$fields = [
'name' => 'Wayne',
'id' => 2,
];
$postdata = http_build_query($fields);
$ch = curl_init()
curl_setopt($ch,CURLOPT_URL, 'http://localhost/request.php');
curl_setopt($ch,CURLOPT_POST, true);
curl_setopt($ch,CURLOPT_POSTFIELDS, $postdata);
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
echo $result;
?>
Output:
Name: Wayne
ID: 2
Create a User-Defined Function That Uses the CURL to Send the POST Request in PHP
We can create a method to take the URL inputs and the POST data to send the POST request. This method also uses the CURL as the second method. It uses all the curl functions as before. This method aims to reuse the code for multiple URLs and data.
For example, create a function httpPost()
and take the variables $url
and $data
as the arguments. Open the curl connection with curl_init()
function and take the variable $url
as the parameter. Use the three curl_setopt()
functions as in the second method. Use the curl_exec()
method to execute the POST data. Create an array $data
and create the keys name
and id
and the values Scott
and 3
. Call the method httpPost()
with the http://localhost/request.php
as the URL and the $data
array as data. This method also uses the request.php
file as the above two methods.
Code Example:
#php 7.x
<?php
function httpPost($url, $data){
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($data));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($curl);
echo $response;
}
$data = [
'name' => 'Scott',
'id' => 3
];
httpPost('http://localhost/request.php', $data);
?>
Output:
Name: Scott
ID: 3
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn