One Line if Statement in PHP
-
if
Statement in PHP -
if...else
Statement in PHP -
if...elseif...else
Statement in PHP - Ternary Operator to Provide the One Line if Statement in PHP
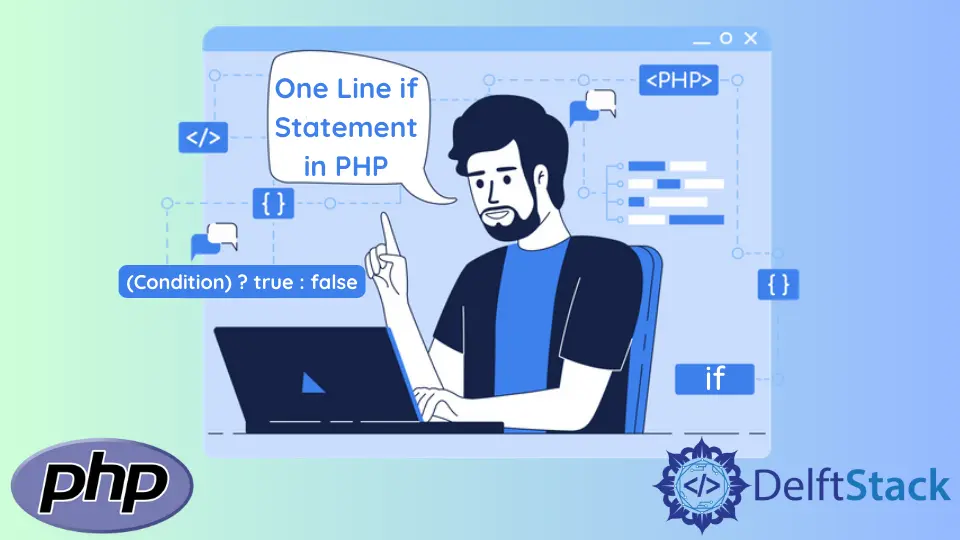
We as programmers often have to make decisions based on certain conditions and write code that is executed by the program if the conditions are met. The if
statement is a decision-making statement available in all programming languages. We will learn about one line if
statement & its alternatives in PHP.
PHP supports 4 differing types of Conditional Statements. All the conditional statements support logical operators inside the condition, such as &&
and ||
.
if
Statement in PHP
The if statement will decide the flow of execution. It executes the code of the if
block only when the condition matches. The program evaluates the code sequentially; if the first condition is true, all other conditions in the sequence will be ignored. This is true for all the conditional statements.
Syntax
if(condition) {
// Code to be executed
}
Example
<?php
$grade = "A";
if($grade = "A"){
echo "Passed with Distinction";
}
?>
Output:
Passed with Distinction
if...else
Statement in PHP
It executes the code of the if
block if the condition matches; otherwise, it executes the code of the else
block. An alternative choice of an else statement to the if
statement enhances the decision-making process.
Syntax
if(condition){
// Code to be executed if condition is matched and true
} else {
// Code to be executed if condition does not match and false
}
Example
<?php
$mark = 30;
if($mark >= 35){
echo "Passed";
} else {
echo "Failed";
}
?>
Output:
Failed
if...elseif...else
Statement in PHP
It Executes the code based on the matching condition. If no condition matches, the default code will be executed written inside the else
block. It combines many if...else
statements. The program will try to find out the first matching condition, and as soon as it finds outs matching condition, it executes the code inside it and breaks the if loop. If no else
statement is given, the program will execute no code by default, and the code following the last elseif
will be executed.
Syntax
if (test condition 1){
// Code to be executed if test condition 1 is true
} elseif (test condition 2){
// Code to be executed if the test condition 2 is true and condition1 is false
} else{
// Code to be executed if both conditions are false
}
Example
<?php
$mark = 45;
if($mark >= 75){
echo "Passed with Distinction";
} else if ($mark > 35 && $mark < 75) {
echo "Passed with first class";
} else {
echo "Failed";
}
?>
Output:
Passed with first class
Ternary Operator to Provide the One Line if Statement in PHP
It is an alternative to if...else
because it provides an abbreviated way of writing the if...else
statements. Sometimes it becomes difficult to read the code written using the ternary operator. Yet, developers use it because it provides a great way to write compact if-else statements.
Syntax
(Condition) ? trueStatement : falseStatement
Condition ?
: A condition to checktrueStatement
: A result if condition matchesfalseStatement
: A result if the condition does not match
The ternary operator selects the value on the left of the colon if the condition evaluates to be true and selects the value on the right of the colon if the condition evaluates to be false.
Let’s check the following examples to understand how this operator works:
Example:
- Using
if...else
<?php
$mark = 38;
if($mark > 35){
echo 'Passed'; // Display Passed if mark is greater than or equal to 35
} else{
echo 'Failed'; // Display Failed if mark is less than 35
}
?>
- Using ternary operator
<?php
$mark = 38;
echo ($mark > 35) ? 'Passed' : 'Failed';
?>
Output:
Passed
There is no difference between both these statements at the byte code level. It writes compact if-else statements, nothing else. Bear in mind that ternary operators are not allowed in some code standards because it decreases the readability of code.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn