Method Overloading in PHP
- Method Overloading in PHP
-
Use the
__call()
Magic Method to Implement Method Overloading in PHP -
Use the
__callStatic()
Magic Method to Implement Method Overloading in PHP
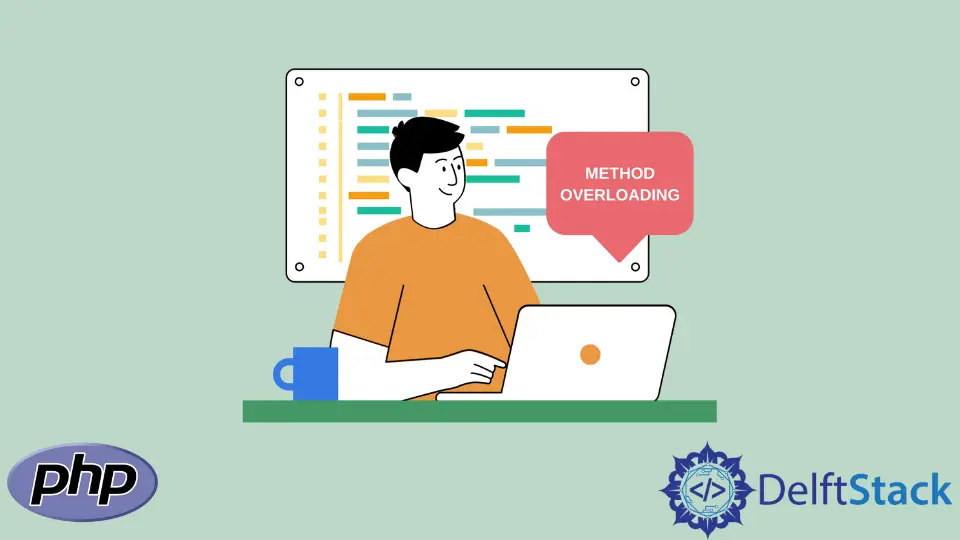
This article will introduce the concept of method overloading in PHP along with examples.
Method Overloading in PHP
Method overloading is a concept in OOP where multiple methods of the same name exist within a class that accepts a different number of parameters or data types. For example, a method add()
can be overloaded by defining it twice; the first one takes two parameters, while the second one takes three parameters.
We can even overload the function by providing integer arguments in one method while providing floating-point arguments in the other. This type of overloading is popular in the languages like Java and C++.
However, the concept of method overloading is quite different in PHP. Method overloading is the process of dynamically creating a method with the help of magic methods.
We can create methods that do not exist within the scope of a class. It works for both class methods and static methods.
The magic methods __call()
and __callStatic()
are used to overload the dynamic methods. It is clear from the name that the __call()
method is used for class methods, and the callStatic()
method is used for static methods.
The syntax of these methods is shown below.
__call($name, $arguments){
//function body
}
Here, $name
is the name of the dynamic method, and $arguments
is an array that holds the arguments of the dynamic method. Inside the method, we can access the arguments using the indices in the $arguments
array.
Use the __call()
Magic Method to Implement Method Overloading in PHP
We can use the __call()
method to create a method in PHP dynamically. The method will be executed when we invoke a method not yet created in the object context.
The process is called method overloading in PHP.
For example, create a class University
and the method __call()
inside it. Name the parameters $name
and $arguments
in this method.
Inside the method, print the $name
variable and the items of the $arguments
array. Outside the class, create an object $student
of the University
class.
Next, invoke the student_info()
method with the $student
object. Pass the arguments 001
and Biswash
in the method.
Example Code:
<?php
class University{
public function __call($name, $arguments){
echo "inside the method: ".$name."<br>";
echo "id:".$arguments[0]."<br>";
echo "name:".$arguments[1]."<br>";
}
}
$student = new University;
$student->student_info(001,'Biswash');
?>
Let’s understand the flow of the example above.
When we invoke the student_info()
method with the parameters, the program doesn’t find the method in the University
class. But, the method __call()
exists in the class, so this function is executed.
The $name
parameter in the method holds the student_info()
method, and the $arguments
parameter holds the arguments 001
and Biswash
as Array ( [0] => 1 [1] => Biswash )
. The parameters are printed inside the __call()
method.
Output:
inside the method: student_info
id:1
name:Biswash
In this way, we can use the __call()
magic method to achieve method overloading in the object context in PHP.
Use the __callStatic()
Magic Method to Implement Method Overloading in PHP
We can also use the method overloading feature in the static context in PHP. We must use the __callStatic()
magic method.
The function definition of the method is similar to that of the __call
method. However, as we use it in the static context, the method should be static.
Since it is a static context, we need not create an instance of the class. We can directly call the method to be created dynamically using the ::
operator from the class.
For example, create a method __callStatic()
inside the class University
as we did for the __call()
method. Do not forget to write the static
keyword while defining the method.
Fill the parameters and body of the method as in the method above. Outside the class, invoke a method professor_info
using the ::
operator by the class as University::professor_info()
.
Supply the arguments of your choice in the method.
Example Code:
<?php
class University{
public static function __callStatic($name, $arguments){
echo "inside the method: ".$name."<br>";
echo "id:".$arguments[0]."<br>";
echo "name:".$arguments[1]."<br>";
}
}
University::professor_info(0010,'Professor Jack ');
?>
Output:
inside the method: professor_info
id:8
name:Professor Jack
This way, we can overload a static method using PHP’s __callStatic()
magic method.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn