How to Send Attachments in Mail With PHP
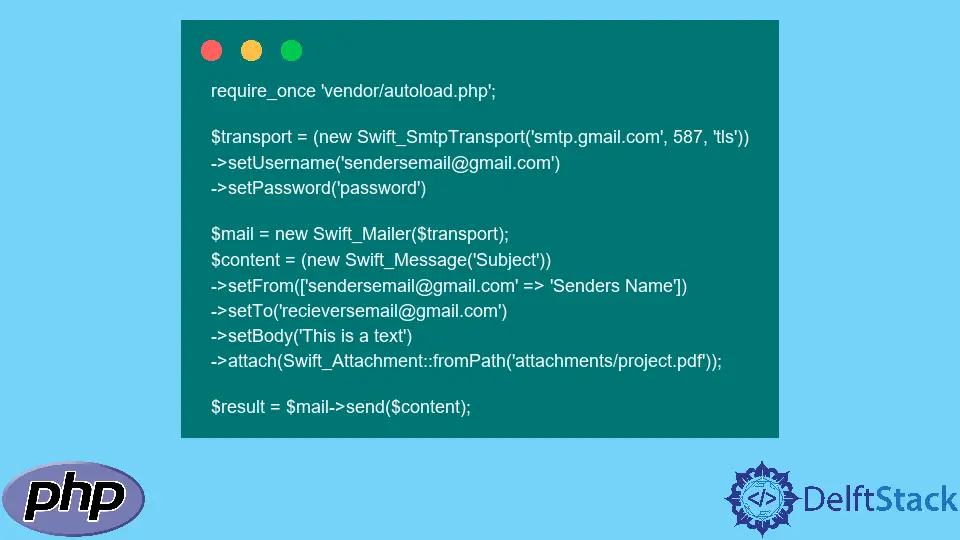
We will introduce different methods to send attachments in email in PHP.
Send Attachments in an Email With PHPMailer
We can use the PHPMailer
class to send an email, allowing us to send attachments. We can create a PHPMailer
class object and use its methods and properties to send emails to desired recipients. We will use Gmail to send an email. So, we will use the SMTP
protocol. The library has the addAttachment()
method that lets us add attachments. Firstly, we need to download the library from GitHub.
For example, create a folder src
and copy the three files PHPMailer.php
, SMTP.php
and Exception.php
to it. Then create a file index.php
and use the require
statement to include these three files. Then use the respective classes of these files. Next, create an object $mail
of the PHPMailer()
class. Set the sender’s email and password with the Username
and Password
properties. Use the Subject
and Body
properties to set the subject and body of the email. Add the attachment using the addAttachment()
function. Give the relative path of the attachment as the method’s parameter. Write the receiver’s email in the AddAddress()
method. Finally, call the Send()
method to send the email. Next, call the smtpClose()
to close the SMTP
connection.
We need to change the sender’s email to send email using Gmail from PHPMailer
. We should turn on the Less Secure Apps access options in Gmail to use the PHPMailer
. Then, running the following script will send an email and attachment to the recipient.
Example Code:
<?php
require 'src/PHPMailer.php';
require 'src/SMTP.php';
require 'src/Exception.php';
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\SMTP;
use PHPMailer\PHPMailer\Exception;
$mail = new PHPMailer();
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = "true";
$mail->SMTPSecure ="tls";
$mail->Port = "587";
$mail->Username = "sendersemail@gmail.com";
$mail->Password = "password";
$mail->SetFrom('sendersemail@gmail.com');
$mail->Subject = 'Message Subject';
$mail->Body = "This is a body text";
$mail->addAttachment('attachments/project.pdf');
$mail->AddAddress( 'receiversmail@gmail.com' );
$mail->Send();
$mail->smtpClose();
?>
Send Attachments in an Email With SwiftMailer
We can send an email with attachments using the third-party library SwiftMailer
as well. The library offers a attach()
method to add attachments while sending an email. We can install the library using the following command.
composer require "swiftmailer/swiftmailer:^6.0"
We need to include the autoloader.php
file in our script to work with SwiftMailer
. The file is located inside the vendor
folder of the downloaded file. We will be sending the email with Gmail. To use Gmail, we need to use the SMTP
protocol. So, we need to create a transport with the Swift_SmtpTransport
class to set the host, port number, and protocol. We can set the sender’s email and password using the transport. The Swift_Mailer
class allows us to set the transport, and the Swift_Mailer
class allows us to set messages, recipients, and attachments.
For example, require the autoload.php
file in the working file as vendor/autoload.php
. Create an object $transport
of the Swift_SmtpTransport
class and set the host as smtp.gmail.com
, port number as 587
, and security protocol as tls
. Then use the setUsername
and setPassword
methods to set the sender’s email and password. Next, create an object $mail
of the Swift_Mailer
class and set the $transport
object to it. Then, create another object $content
of the Swift_Message
class and write the subject as the parameter. Use the setFrom()
and setTo()
methods to specify the sender’s email and receiver’s email. Write the email body in the setBody()
method. Then, use the attach()
method to specify the attachment path using the fromPath()
method of the Swift_Attachment
class. Finally, use the $mail
object that we created to send the email with the send()
method. Supply the $content
object as the parameter of the send()
method.
This is how we can send an email with attachments using the SwiftMailer
library in PHP.
Example Code:
require_once 'vendor/autoload.php';
$transport = (new Swift_SmtpTransport('smtp.gmail.com', 587, 'tls'))
->setUsername('sendersemail@gmail.com')
->setPassword('password')
$mail = new Swift_Mailer($transport);
$content = (new Swift_Message('Subject'))
->setFrom(['sendersemail@gmail.com' => 'Senders Name'])
->setTo('recieversemail@gmail.com')
->setBody('This is a text')
->attach(Swift_Attachment::fromPath('attachments/project.pdf'));
$result = $mail->send($content);
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn