How to Get Last Array Element in PHP
-
Extract Last Array Element Using
array_values()
in PHP -
Extract Last Array Element Using
end()
in PHP -
Extract Last Array Element Using
count()
in PHP -
Extract Last Array Element Using
array_key_last()
in PHP -
Extract Last Array Element Using
array_keys()
in PHP
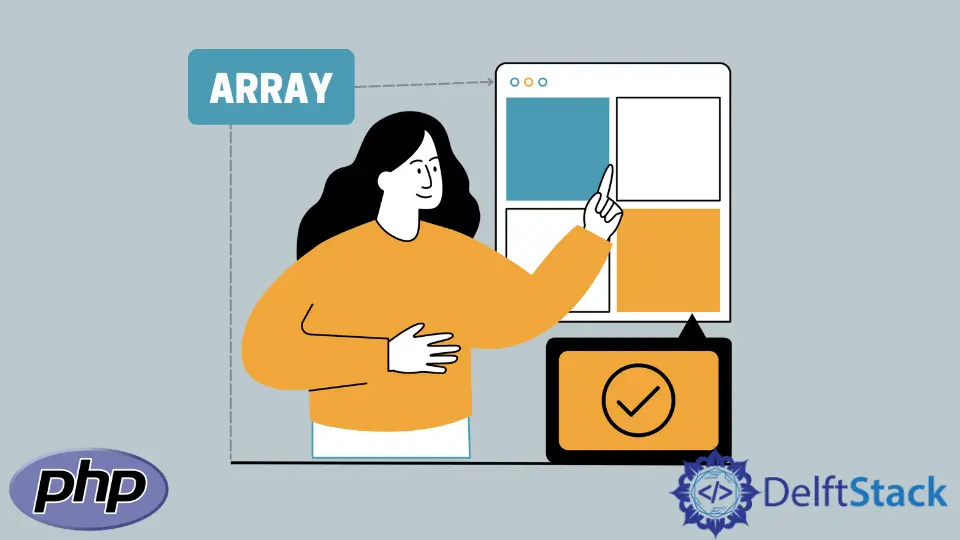
Arrays are an essential part of any programming language because they hold many elements accessed via an index. In today’s article, we will learn how to extract the last element of an array.
Extract Last Array Element Using array_values()
in PHP
It is a built-in PHP function that takes an associative array as input and returns values of the array with key replaced by numeric key.
Syntax of array_values()
array_values(array $array);
Parameters
$array
: It is a mandatory parameter. It refers to the original array from which values will be extracted.
Return Value
Return an array with the fetched values in the associative array form with a numeric key.
Example code:
<?php
$array = array("first" => 1, "second" => 2);
$lastElement = end(array_values($array));
echo $lastElement ;
?>
Output:
2
Extract Last Array Element Using end()
in PHP
PHP provides the end()
function to move and set the internal pointer of an array to its last element and prints the value.
Syntax of end()
end(array $array);
Parameters
$array
: It is a mandatory parameter. It refers to the original array on which the pointer will be set.
Return Value
Depending on the input array, it will return the value of the last element in the array. If an empty array is passed, it will return false
. If a multidimensional array is passed, it will return the last array.
Example code:
<?php
$array = array("first" => 1, "second" => 2);
$lastElement = end(array_values($array));
echo $lastElement . "<br>";
$lastArrayElement = end($array);
echo $lastArrayElement . "<br>";
$multidimensionalArray = array(array("a", "b", "c"), array("d", "e", "f"), array("g", "h", "i"));
$mdLastElement = end(end($multidimensionalArray));
echo $mdLastElement . "<br>";
?>
Output:
2
2
i
Extract Last Array Element Using count()
in PHP
PHP provides the count()
function to count the number of elements inside an array. It can count all the elements inside a multidimensional array if specified.
Syntax of count()
count(array $array, boolean $mode);
Parameters
$array
: It is a mandatory parameter. It refers to the original array on which count action will be performed.
$mode
: It is an optional parameter, and it takes 2 values as input. 0
refers to a default value, which tells PHP not to count all the elements inside a multidimensional array. 1
refers to count, which tells PHP to count all the elements inside a multidimensional array.
Return Value
It returns an integer value as the total count of elements.
Example code:
<?php
$array = array("Mac", "Windows", "Ubuntu", "Linux");
$lastElement = $array[count($array)-1];
echo $lastElement. "<br>";
$multidimensionalArray = array(array("a", "b", "c"), array("d", "e", "f"), array("g", "h", "i"));
// First extract last array from multidimensional array
$lastArray = end($multidimensionalArray);
// Extract last element of last array in multidimensional array
$lastArrayElement = $lastArray[count($lastArray)-1];
echo $lastArrayElement;
?>
Output:
Linux
i
Extract Last Array Element Using array_key_last()
in PHP
It is a built-in PHP function that gives the last key of an array. You can use this function to get the last key and fetch its value.
Syntax of array_key_last()
array_key_last(array $array);
Parameters
array
: It is a mandatory parameter that specifies an original array from which the last key will be extracted.
Return Value
If the input array is not empty, it will return the last key of an array. On empty array input, it will return NULL
.
Example code:
<?php
$array = array("Mac", "Windows", "Ubuntu", "Linux");
$lastElement = $array[array_key_last($array)];
echo $lastElement;
?>
Output:
Linux
Extract Last Array Element Using array_keys()
in PHP
It is a built-in PHP function that returns extracted keys of an original array in a new associative array, where the key will be the numeric index and values will be extracted keys.
Syntax of array_keys()
array_keys(array $array, int|string $value, boolean $strict);
Parameters
$array
: It is a mandatory parameter. It specifies an original array from which keys will be extracted.
$value
: It is an optional parameter. It specifies a value, and only the keys whose values are set to this input value match will return the keys.
$strict
: It is an optional parameter. Users can pass this parameter if they want to control the value and the data type strictly.
Possible values:
-
true
- Returns the keys whose value strictly matches with specified value along with data type check. For example, number 1 is not the same as the string “1”. -
false
- Returns the keys whose value strictly matches with specified value but does not perform a data type check. For example, number 1 is the same as the string “1”.
Return Value
It returns an associative array with the numeric key and the original array’s key as value.
Example code:
<?php
$array = array("Mac", "Windows", "Ubuntu", "Linux");
$keys = array_keys($array);
$lastElement = $array[$keys[count($keys)-1]];
echo $lastElement;
?>
Output:
Linux
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn