How to Divide Integer in PHP
-
Use Division Operator
a/b
to Divide Integers in PHP -
Use the
intdiv()
Method to Divide Integers in PHP
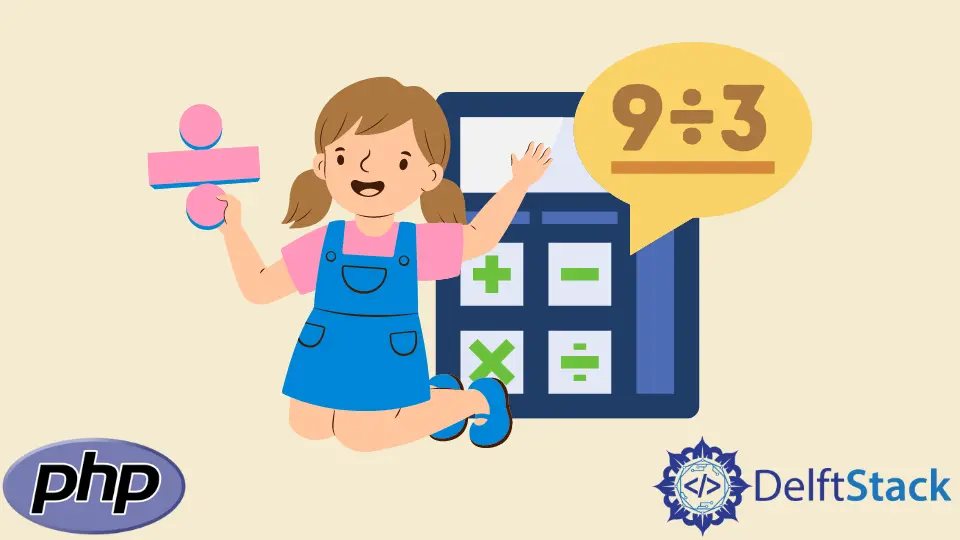
This tutorial demonstrates how to divide integers in PHP.
The are two methods to divide integers in PHP. One is by the division /
operator, and the other is by the intdiv()
method.
This tutorial demonstrates both methods for integer division in PHP.
Use Division Operator a/b
to Divide Integers in PHP
The /
is used as a division operator in PHP and can divide two numeric values. This method will return the exact answer for the division, which can be a non-integer value; let’s see an example.
<?php
$dividend_number = 8;
$divisor_number = 3;
$result = $dividend_number / $divisor_number;
echo "The result for the given integer division is: ".$result;
?>
The result for division is:
The result for the given integer division is: 2.6666666666667
As we can see, the result is not in an integer type; to convert this result to an integer, we need to use the round()
or floor
method. See the example.
<?php
$dividend_number = 8;
$divisor_number = 3;
$result = $dividend_number / $divisor_number;
echo "The result for the given integer division is: ".$result."<br>";
echo "The rounded result for the given integer division is: ". round($result)."<br>";
echo "The floored result for the given integer division is: ".floor($result)."<br>";
?>
The round
method will round the non-integer number to the upper integer, and the floor
method will round it to the lower integer. See output:
The result for the given integer division is: 2.6666666666667
The rounded result for the given integer division is: 3
The floored result for the given integer division is: 2
Use the intdiv()
Method to Divide Integers in PHP
The built-in method intdiv()
is used for the integer division, which also returns an integer no matter if the answer is in decimal value. The method intdiv()
actually removes the remainder from the dividend so it can be divided without any decimals.
The syntax for this method is:
int intdiv($dividend_number, $divisor_number)
The first parameter is the dividend number, and the second number is the divisor number. The method will return the quotient calculated.
The function will return an error or exception in the following conditions.
- If the divisor passed is 0, then the method will throw a
DivisionByZeroError
exception. - If the dividend is
PHP_INT_MIN
and the divisor is-1
, an arithmetic error exception will be thrown.
Let’s try an example.
<?php
$dividend_number = 8;
$divisor_number = 3;
$result = intdiv( $dividend_number , $divisor_number);
echo "The result for the given integer division is: ".$result."<br>";
$dividend_number = -8;
$divisor_number = 3;
$result = intdiv( $dividend_number , $divisor_number);
echo "The result for the given integer division is: ".$result."<br>";
?>
The code above first tries to divide two positive numbers and then one negative and one positive number using the intdiv()
method. See the output:
The result for the given integer division is: 2
The result for the given integer division is: -2
As we see, the method intdiv
will divide the two integers, and if the result is decimal, the method will convert it to the lower integer.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook