How to Check if Post Exists in PHP
-
Check if
$_POST
Exists Withisset()
-
Check if
$_POST
Exists With theEmpty()
Function -
Check if
$_POST
Exists Withisset()
Function and Empty String Check -
Check if
$_POST
Exists With Negation Operator
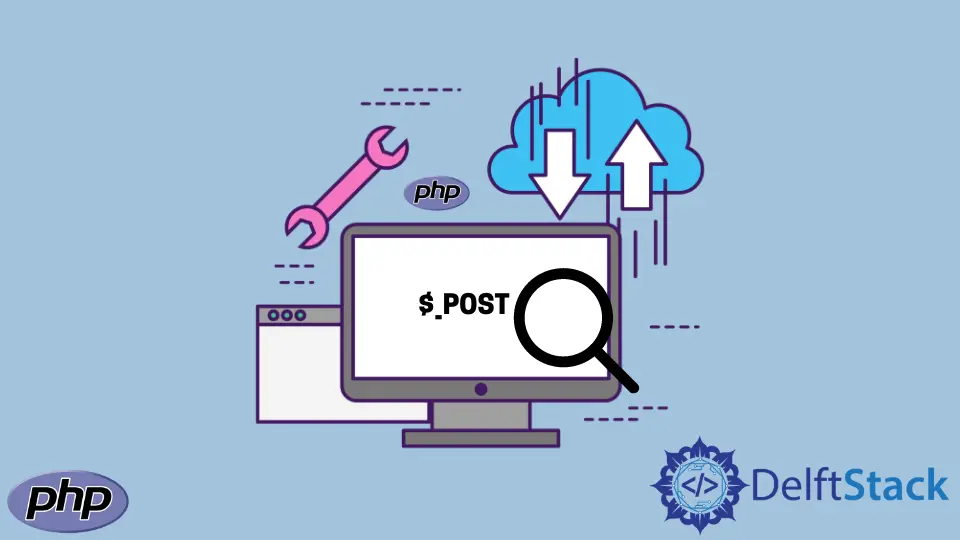
PHP $_POST
is a super-global variable that can contain key-value pair of HTML form data submitted via the post method. We will learn different methods to check if $_POST
exists and contains some data in this article. These methods will use isset()
, empty()
, and empty string check.
Check if $_POST
Exists With isset()
The isset()
function is a PHP built-in function that can check if a variable is set, and not NULL. Also, it works on arrays and array-key values. PHP $_POST
contains array-key values, so, isset()
can work on it.
To check if $_POST
exists, pass it as a value to the isset()
function. At the same time, you can check if a user submitted a particular form input. If a user submits a form input, it will be available in $_POST
, even if it’s empty.
The following HTML provides us with something to work with. It has a form field with a prepopulated name field.
<form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>">
<label>First Name</label>
<input type="text" name="first_name" value="DelftStack">
<input type="submit">
</form>
The following PHP will check if $_POST
exists when you click the submit button:
<?php
if (isset($_POST['first_name'])) {
$first_name = $_POST['first_name'];
echo $first_name;
}
?>
Output:
DelftStack
Check if $_POST
Exists With the Empty()
Function
You can check the existence of $ _POST
with the empty()
function. But, the empty()
function will return true in the following cases:
- When all
$_POST
values are empty strings - The argument is zero
The following HTML is like the previous one, this time, the name is different:
<form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>">
<label>First Name</label>
<input type="text" name="first_name" value="Mathias Jones">
<input type="submit">
</form>
The next code block will show you how to check for $_POST
with the empty()
function:
<?php
if (!empty($_POST)) {
$first_name = $_POST['first_name'];
echo $first_name;
}
?>
Output:
Mathias Jones
Check if $_POST
Exists With isset()
Function and Empty String Check
The isset()
function returns true if the value of $_POST is an empty string, but it will return false for NULL values. if you try to print the values of isset($_POST['x']) = NULL
and isset($_POST['x']) = ''
, in both cases, you’ll get an empty string.
As a result, you will need to check for empty strings. The combination of isset()
and empty string check eliminates the possibility that $_POST
contains empty strings before you process its data.
In the next code block, we have an HTML to work with:
<form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>">
<label>First Name</label>
<input type="text" name="first_name" value="Mertens Johanssen">
<input type="submit">
</form>
Check if $_POST
exists:
<?php
if (isset($_POST['first_name']) && $_POST['first_name'] !== "") {
$first_name = $_POST['first_name'];
echo $first_name;
}
?
Output:
Mertens Johanssen
Check if $_POST
Exists With Negation Operator
The negation operator (!) will turn a true statement into false and a false statement into true. Therefore, you can check if $_POST
exists with the negation operator. To check for $_POST
, prepend it with the negation operator in an if-else statement.
In the first part, if $_POST
is empty, you can stop the p processing of its data. In the second part of the conditional, you can process the data.
First, the HTML:
<form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>">
<label>First Name</label>
<input type="text" name="first_name" value="Marcus Alonso">
<input type="submit">
</form>
The next code block demonstrates checking if $_ POST
exists with the negation operator.
<?php
if (!$_POST) {
echo "Post does not exist";
} else {
$first_name = $_POST['first_name'];
echo $first_name;
}
?>
Output:
Marcus Alonso
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn