How to Use if...else Shorthand in PHP
- Introduction to the Ternary Operator in PHP
-
Use the Ternary Operator for
true/false
Declaration in PHP
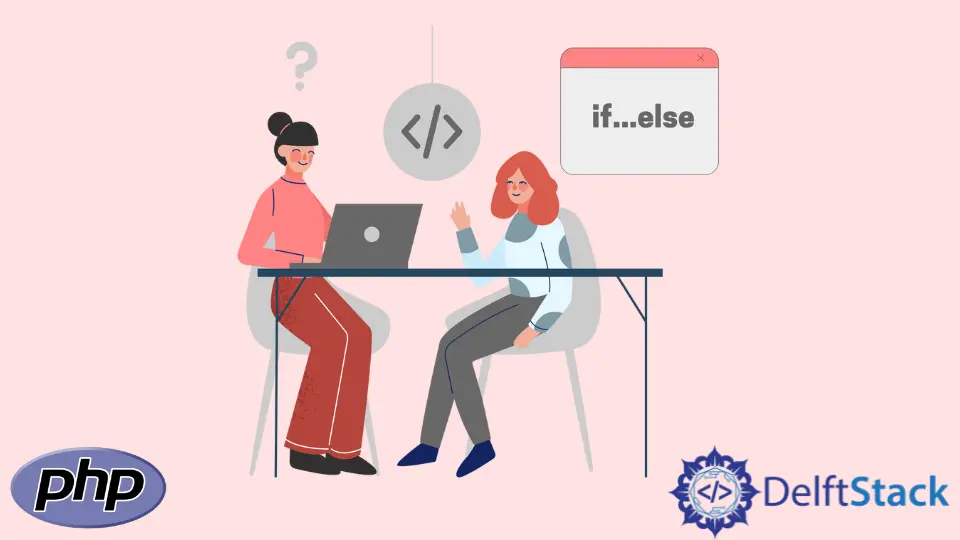
This article will introduce how we can use the short-hand method to write the if...else
condition in PHP. We will use the ternary operator and null coalescing operator with the demonstrations.
Introduction to the Ternary Operator in PHP
We can use the ternary operator as a short-hand method of if-else
condition in PHP. However, the ternary operator can be used in every other programming language. The word ternary means having three elements. So, the ternary operator has three operands. We write the condition at first, which is the first operand. Then the symbol ?
separates the condition with the expression to be evaluated if the condition is true. Lastly, the :
symbol separates the truthy and the falsy expression. The syntax is written below.
condition ? trueExpression : falseExpression
Here, condition
is an expression whose value is to be evaluated. The option trueExpression
is an expression that will be executed if condition
is true, and falseExpression
will be executed if condition
is false.
Let’s see how we can write equivalent ternary operation of the if-else
condition. Let’s have a look at the following if-else
condition.
Example Code:
$num = rand(0,10);
echo "The number is: " . "$num"."<br>";
if($num>5){
echo "heads";
}else{
echo "tails";
}
Output:
The number is: 9
heads
Here, we used an if-else
condition to display heads or tails using the rand()
function. If the number is greater than 5, we displayed heads, and we displayed tails if the number is less than or equal to 5. Here, rand(0,10)
generates a random number from 0
to 10
. In the example below, the random number is 9, and it displays heads as a result. The important thing to consider is that we cannot use the echo
statement with the conditional operators themselves. We can only use them after the condition is evaluated as a result. We can substitute the if-else
condition by using the ternary operator for the above program.
Example code:
$num = rand(0,10);
echo "The number is: " . "$num"."<br>";
echo ($num>5)? "heads":"tails";
Output:
The number is: 6
heads
In the example below, the random number is 6. It is greater than 5 so, the program displays heads. The significant difference between the if-else
operator and the ternary operator is that we can use the echo
statement and the ternary operator. In this way, we can use the ternary operator.
Use the Ternary Operator for true/false
Declaration in PHP
We can also use the ternary operator to declare the basic true
or false
values. We can use the boolean value true
and false
in trueExpression
and falseExpression
respectively. For example, create a variable $age
and assign it to the value of 14
. Next, create another variable, $can_vote
and write a ternary operation in the variable. Write the condition $age>17
and write the values true
and false
as trueExpression
and falseExpression
. Lastly, use the var_dump()
function to dump the $can_vote
variable.
The output section shows the output as false
, which is a boolean type. In this way, we can use the ternary operator to declare the true
and false
values in PHP.
Code Example:
$age= 14;
$can_vote = ($age>17 ? true : false);
var_dump($can_vote);
Output:
bool(false)
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn