How to Get User IP Address in PHP
-
Use
$_SERVER['REMOTE_ADDR']
to Find the User’s IP Address in PHP -
Use
$_SERVER['REMOTE_ADDR']
,$_SERVER['HTTP_CLIENT_IP']
and$_SERVER['HTTP_X_FORWARDED_FOR']
to Find the User’s IP Address -
Use Ternary Operator and
isset()
Function to Find the User’s IP Address
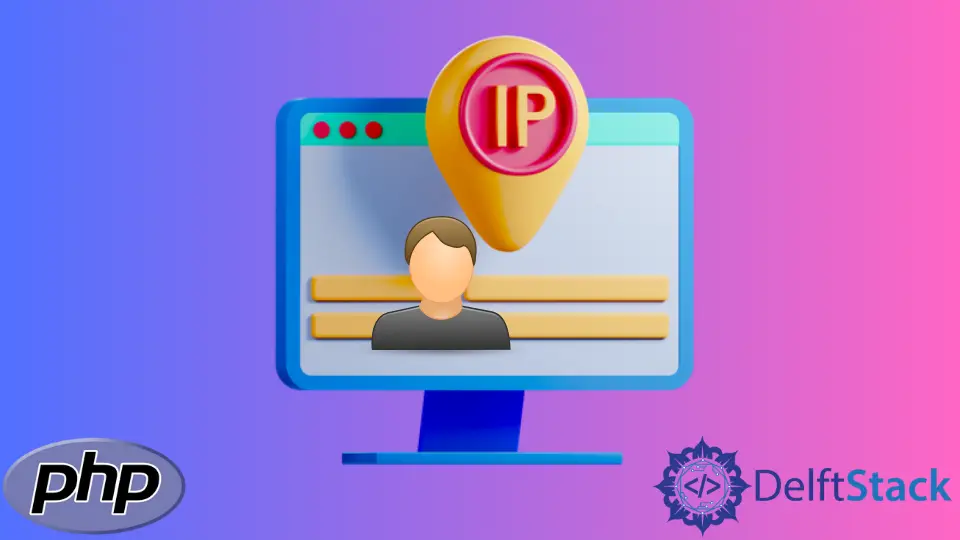
We will introduce a way to access the user’s IP address from a server in PHP using the $_SERVER
super global variable with REMOTE_ADDR
as the only array element. It is used as $_SERVER['REMOTE_ADDR']
.
We will demonstrate another way to access the user’s IP address in PHP using the conditional expression to check whether the user’s IP addresses come from shared internet, from a proxy, or is the real IP address. This method uses array elements like HTTP_CLIENT_IP
, HTTP_X_FORWARDED_FOR
and REMOTE_ADDR
.
We will show you a short-hand method to get the user’s IP address in PHP using the ternary operator and isset()
function. This method also uses the array elements like HTTP_CLIENT_IP
, HTTP_X_FORWARDED_FOR
and REMOTE_ADDR
in the $_SERVER
super global variable.
Use $_SERVER['REMOTE_ADDR']
to Find the User’s IP Address in PHP
We can use the $_SERVER['REMOTE_ADDR']
expression to find the IP address from where the user is accessing the current page. $_SERVER
is the super global variable in PHP, and it takes a variety of array elements as arguments. We use the REMOTE_ADDR
as the array element in $_SERVER
variable to access the client’s actual IP address. Note that $_SERVER['REMOTE_ADDR']
does not always give the correct IP address of the client in all cases. We will discuss the other cases below. Ensure your Server API(SAPI) is well-configured so that the above expression will return the real IP of the connection.
In the example below, the localhost
is used as the server. Thus, it returns the loopback IP address. To learn about the loopback address, please refer here. Suppose the URL of the following PHP script is http://localhost/index.php
. It outputs the IP address of the local machine as ::1
. ::1
is the IPv6 representation of the localhost
.
For example, assign the $_SERVER['REMOTE_ADDR']
to a variable ip_addr
. Then, print the variable using the echo
.
Example Code:
# php 7.x
<?php
$ip_add = $_SERVER['REMOTE_ADDR'];
echo "The user's IP address is - ".$ip_add;
?>
Output:
The user's IP address is - ::1
Use $_SERVER['REMOTE_ADDR']
,$_SERVER['HTTP_CLIENT_IP']
and $_SERVER['HTTP_X_FORWARDED_FOR']
to Find the User’s IP Address
We can use the array elements of the superglobal variable $_SERVER
like $_SERVER['REMOTE_ADDR']
,$_SERVER['HTTP_CLIENT_IP']
and $_SERVER['HTTP_X_FORWARDED_FOR']
to find the IP address of the user in PHP. Use$_SERVER['REMOTE_ADDR']
to find the real IP address of the user. Use $_SERVER['HTTP_CLIENT_IP']
to find the IP address when the user is accessing the page from shared internet. Use $_SERVER['HTTP_X_FORWARDED_FOR']
to find the IP address when the user uses a proxy to access the webpage. Note that headers like HTTP_X_FORWARDED_FOR
and HTTP_CLIENT_IP
can be spoofed as this can be set by anyone. Sometimes, they may not represent the real IP address of the client.
For Example, use the empty()
function to check whether the $_SERVER['HTTP_CLIENT_IP']
contains something. If it contains, assign the vale in the $ip
variable and print i.
Again, perform a check on $_SERVER['HTTP_X_FORWARDED_FOR']
accordingly and assign the value and print it if it exists. Perform similar operation on $_SERVER['REMOTE_ADDR']
.
The example below, $_SERVER['REMOTE_ADDR']
returns the IP address as the user is not using any proxies or shared internet connection. Check the PHP Manual to know more about the $_SERVER
array.
Example Code:
#php 7.x
<?php if (!empty($_SERVER['HTTP_CLIENT_IP'])) {
echo $ip = $_SERVER['HTTP_CLIENT_IP'];
} elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) {
echo $ip = $_SERVER['HTTP_X_FORWARDED_FOR'];
} else {
echo $ip = $_SERVER['REMOTE_ADDR'];
}
?>
Output:
::1
Use Ternary Operator and isset()
Function to Find the User’s IP Address
We can use a short-hand method to find the user’s IP address in PHP using the ternary operator. In this method, the isset()
method checks whether the array contains the specified elements or the header files.
For example, use the isset()
function to check whether the array contains HTTP_CLIENT_IP
. If the condition is true, HTTP_CLIENT_IP
is set. Then, If the condition fails, isset()
function is used to check whether the array contains HTTP_X_FORWARDED_FOR
. Similarly, if the condition is true, HTTP_X_FORWARDED_FOR
is set, and REMOTE_ADDR
is set when the condition fails.
In the example below, the client is not using a proxy or a shared internet. Therefore, the $_SERVER['REMOTE_ADDR']
gets executed. Please check the MSDN Web Docs to know about the ternary operator.
Example Code:
#php 7.x
<?php
$ip = isset($_SERVER['HTTP_CLIENT_IP']) ? $_SERVER['HTTP_CLIENT_IP'] : isset($_SERVER['HTTP_X_FORWARDED_FOR']) ? $_SERVER['HTTP_X_FORWARDED_FOR'] : $_SERVER['REMOTE_ADDR'];
echo "The user IP Address is - ". $ip;
?>
Output:
The user IP Address is - ::1
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn