PHP Error Handlers
- What Is Error Handling in PHP
-
Basic Error Handling With the
die()
Function in PHP - Create a Custom Error Handler in PHP
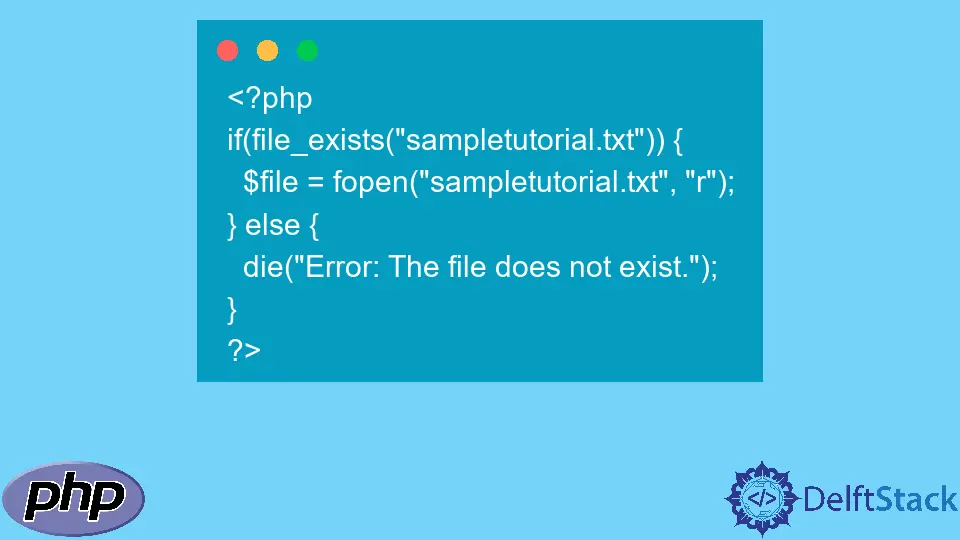
In this tutorial, we will introduce error handling in PHP. We will cover the importance of error handling on PHP and how it works.
What Is Error Handling in PHP
This is the process of identifying errors in your program and acting upon them. PHP error checking code will improve your program’s security and make it more professional.
Among the methods we will look at are:
- Basic error handling with the
die()
function. - Custom error handling.
Basic Error Handling With the die()
Function in PHP
The die()
function will send a message to the web and exit the script.
In the example code below, we will attempt to open a file that does not exist in our root folder. Let’s see what happens.
<?php
$file=fopen("sampletutorial.txt","r");
?>
Output:
Warning: fopen(sampletutorial.txt): Failed to open stream: No such file or directory in C:\xampp\htdocs\projects\New folder\PHP Error Handling\Example code 1.php on line 2
To prevent this error from showing, we can use the die()
function to print out a message and exit the script, as shown below.
<?php
if(file_exists("sampletutorial.txt")) {
$file = fopen("sampletutorial.txt", "r");
} else {
die("Error: The file does not exist.");
}
?>
Output:
Error: The file does not exist.
That’s much better. Let’s look at custom error handlers.
Create a Custom Error Handler in PHP
A custom error handler must have a minimum of two parameters.
error_level
- This is the error report level.error_message
- This is the message you want to print out.
The function accepts up to five parameters. These are:
error_file
- This parameter specifies the file with the error.error_line
- This parameter shows the coding line with an error.error_context
- Shows all variables and values with errors.
Different types of Error Report Levels:
[1] E_ERROR
[2] E_WARNING
[8] E_NOTICE
[256] E_USER_ERROR
[512] E_USER_WARNING
[1024] E_USER_NOTICE
[2048] E_STRICT
[8191] E_ALL
Let’s create a custom error handler. The example code below shows how we can create a custom function to handle errors in PHP.
<?php
function mycustomError($errno, $errstr) {
echo "<b>Error:</b> [$errno] $errstr<br>";
echo "Terminating Script";
die();
}
?>
This is how we create a custom error handler. The next thing is to set out an error handler, as shown below.
<?php
//error handler function
function mycustomError($errno, $errstr) {
echo "<b>Error:</b> [$errno] $errstr";
}
//set error handler
set_error_handler("mycustomError");
//trigger error
echo($t);
?>
Output:
Error: [2] Undefined variable $t
Since the variable $t
is not set, we get an error message, as shown on the output.
Trigger an Error:
It is important to trigger errors in scripts where users can input data. This way, users will not input illegal commands.
We use the trigger_error()
function in PHP, as shown in the example code below.
<?php
//error handler function
function mycustomError($errno, $errstr) {
echo "<b>Error:</b> [$errno] $errstr<br>";
echo "Terminating Script";
die();
}
//set error handler
set_error_handler("mycustomError",E_USER_WARNING);
//trigger error
$t = 7;
if ($t>=1) {
trigger_error("Value entered must be 1 or below",E_USER_WARNING);
}
?>
Output:
Error: [512] Value entered must be 1 or below
Terminating Script
In the example code above, we trigger an error by giving the variable $t
the value of 7. Our trigger_error()
function is set when the variable $t
is greater than 1.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn