How to Create a PHP Dropdown List
- Create a Dropdown List in PHP
- Create a Static Dropdown List in PHP
- Create a Dynamic Dropdown List in PHP
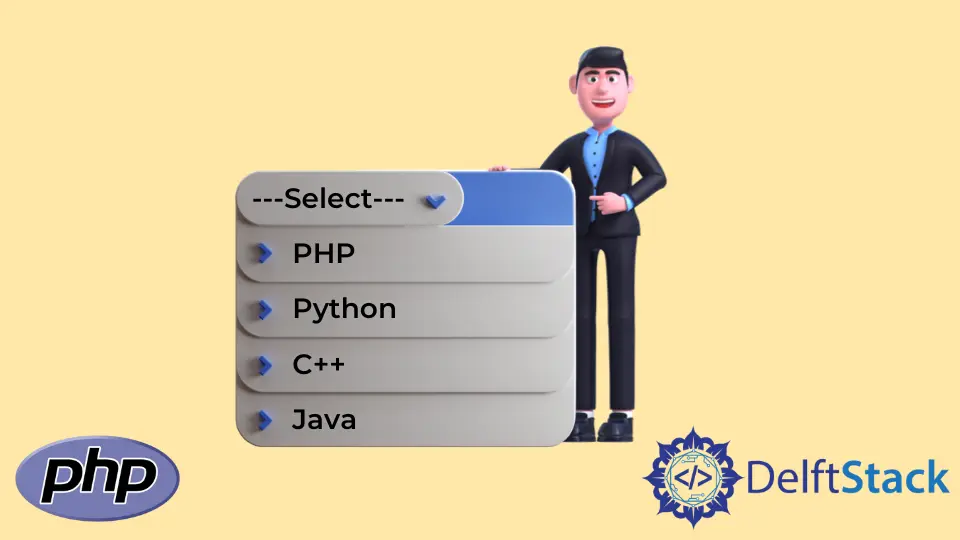
This article will introduce the dropdown list and create one with PHP.
Create a Dropdown List in PHP
A dropdown list is a group of items in a list. The contents are not visible until you click on the small arrow.
This article will look at the two types of dropdown lists.
- Static Dropdown list
- Dynamic Dropdown list
Let’s first look at the Static dropdown list.
Create a Static Dropdown List in PHP
A static dropdown list is a simple PHP dropdown box with no database connection. We will create a static dropdown box for some programming languages in the example code below.
In the list, we will have the following languages.
- PHP
- Python
- Java
- C++
We will then use PHP to echo
out the selected language.
Code:
//Create a static dropdown box
<form id="L" method="post">
<select name="Language">
<option value="PHP">PHP</option>
<option value="Python">Python</option>
<option value="Java">Java</option>
<option value="C++">C++</option>
</select>
<input type="submit" name="Submit" value="Submit">
</form>
<?php
if(isset($_POST['Language'])) {
echo "Selected Language: ".htmlspecialchars($_POST['Language']);
}
?>
The dropdown box should look like this.
We clicked the arrow to display the full list of items in the dropdown box in the image above. Let’s try and choose the language PHP
from the menu and see what happens.
That is how you create a dropdown box without a database connection. Let’s now look at the dynamic dropdown list.
Create a Dynamic Dropdown List in PHP
A dynamic dropdown list fetches contents from a database. Let’s look at an example.
We have a MySQL database called sample tutorial
. In our database, we have the table parkinglot
.
See the table below.
From the table above, we will create a dropdown box that fetches the contents of our BrandName
row.
First, we will create a database connection and use the SELECT * FROM
function to fetch the contents of the BrandName
row. Lastly, we will create a dropdown menu for the above items.
Code:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
$con = mysqli_connect("localhost", $user, $pass, $db);
$sql = "SELECT `BrandName` FROM `parkinglot1` WHERE 1;";
$car_brands = mysqli_query ($con, $sql);
?>
<html>
<head>
<title>Dynamic Drop Down Box</title>
</head>
<BODY bgcolor ="yellow">
<form id="form" name="form" method="post">
Car Brands:
<select Brand Name='NEW'>
<option value="">--- Select ---</option>
<?php
while ($cat = mysqli_fetch_array(
$car_brands,MYSQLI_ASSOC)):;
?>
<option value="<?php echo $cat['BrandName'];
?>">
<?php echo $cat['BrandName'];?>
</option>
<?php
endwhile;
?>
</select>
<input type="submit" name="Submit" value="Select" />
</form>
</body>
</html>
Output:
The code is a success. We managed to fetch the contents of our table from our database and use them in our dropdown box.
This article shows how to create the two dropdown lists types in PHP.
The code for the dynamic dropdown box does not execute when you select any of the car brands. It only shows the contents in our database.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn