How to Create PDF in PHP
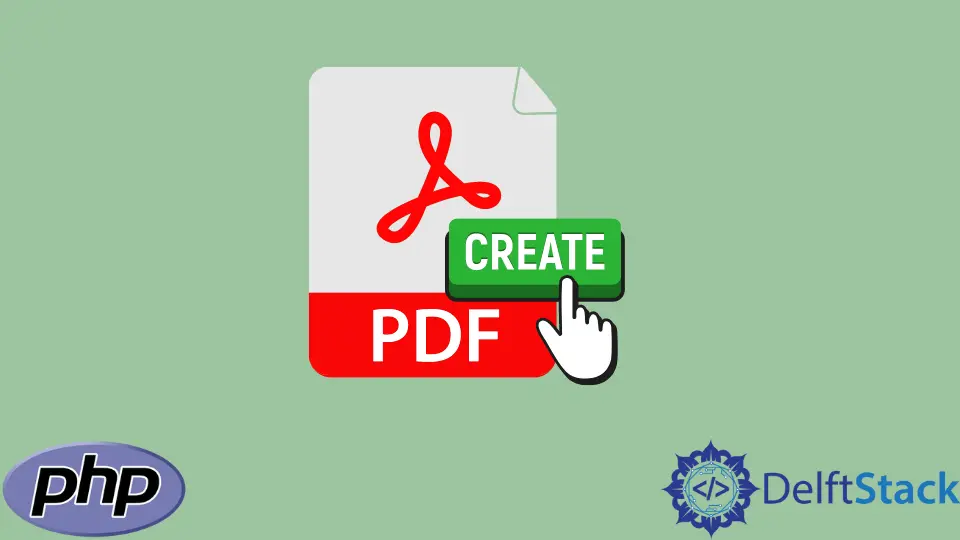
This article will introduce methods to create PDF in PHP. We will retrieve the data from the database, put it in the PDF, and download it.
Use the mpdf
Library to Create PDF in PHP
We can use the external library mpdf
to create a PDF in PHP. We can retrieve the data from the database, store them in PDF, and download the PDF. Using this library, we can create PDF from HTML documents. The HTML documents should be encoded in UTF-8. We can retrieve the data to be added to the PDF from the database in an HTML format. We can download it by the command composer require mpdf/mpd
from the project directory to use the library. The command will install the mpdf
library in the project directory. A vendor
file will be created, and we need to use the require()
function to include the file autoload.php
located inside the vendor
folder. We have to make sure that the directory where the library is installed should have write permission.
We will create an object from the Mpdf()
constructor and use methods like WriteHTML()
and output()
to create the PDF. There are different modes in which we can output the PDF. We can specify the modes in the second parameter of the output()
method. The different modes are represented by the D
, I
, F
and S
options. The option D
will forcefully download the PDF after the script runs. The option I
will show the PDF in the browser after the script runs. Meanwhile, the option F
will download the PDF saves in the folder relative to the PHP file. Lastly, the option F
will output the pdf in the browser only when the output()
method is assigned to a variable.
For example, we have a database named phprow
containing a table named Persons
. The table Persons
contains the following data.
+----------+----------+-----------+
| PersonID | Name | Address |
+----------+----------+-----------+
| 22 | Harry M | England |
| 32 | Paul P | France |
+----------+----------+-----------+
First, use the require()
function to include the vender/autoload.php
file. Then, create and establish a database connection, run the SQL query to select the data from the database, and create a table in the $html
variable. Use the .
operator to concatenate the $html
variable with the bodies of the table. Create a table with the table headers ID
, Name
, and Address
. Then populate the table by retrieving the above-shown data from the Persons
table.
Example Code:
require('vendor/autoload.php');
$con=mysqli_connect('localhost','root','','phprow');
$res=mysqli_query($con,"select * from Persons");
if(mysqli_num_rows($res)>0){
$html='<table>';
$html.='<tr><td>ID</td><td>Name</td><td>Address</td>';
while($row=mysqli_fetch_assoc($res)){
$html.='<tr><td>'.$row['PersonID'].'</td><td>'.$row['Name'].'</td><td>'.$row['Address'].'</td></tr>';
}
$html.='</table>';
}
In the example above, we have stored a table with the data from the database in the variable $html
. We have used the .
operator to concatenate all the table elements. Thus, we made an HTML document ready to write into a PDF.
Next, create a variable named $mpdf
. Assign the object of the Mpdf()
constructor to the variable using the new
keyword. Call the WriteHTML()
function with the $html
variable as the parameter with the object. Then create another variable, $file
to store the PDF. Join files/
with the time()
function and again concatenate it with .pdf
to create the file name. Store it in the $file
variable. Lastly, call the output()
function with $file
as the first parameter and the option I
as the second parameter.
Thus, we retrieved data from the database and created a PDF with those data. The example below creates a file with the name of the current time with a .pdf
extension inside the files
folder. The PDF will be shown in the browser after the script runs. We can download the PDF from the browser.
Example Code:
$mpdf=new \Mpdf\Mpdf();
$mpdf->WriteHTML($html);
$file='files/'.time().'.pdf';
$mpdf->output($file,'I');
Use the dompdf
Library to Create PDF in PHP
The dompdf
library is also an option to create and download a PDF in PHP. It lets us load the HTML to the PDF. This library is very much similar to the mpdf
library; only the methods are different. We will use the methods like loadHtml()
, render()
and stream()
. We need to download the library to our working directory using the command composer require dompdf/dompdf
. It will create the vendor
folder as in the first method along with the composer.json
and composer.lock
files.
For example, require the vendor/autoload.php
as the first line of the code in the program. Then write the use
keyword to import the Dompdf
class as use Dompdf/Dompdf
. We can use the same HTML table as in the method above to load in the PDF.
require 'vendor/autoload.php';
use Dompdf\Dompdf;
After storing the HTML in a variable $html
, create another variable $dompdf
to create an object of the class Dompdf
. Then call the loadHtml()
method with $html
as the parameter. Next, call the render()
function and then the stream()
function with the $dompdf
object.
The example below will create a PDF with the table as in the first method. The render()
method renders the HTML as a PDF file, and the stream()
method outputs the rendered HTML to the browser. Thus, we can create a PDF using the dompdf
library in PHP.
Example Code:
$dompdf = new Dompdf();
$dompdf->loadHtml($html);
$dompdf->render();
$dompdf->stream();
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn