How to Create Countdown Timer in PHP
- Create a Static Countdown Timer in PHP
-
Use
TimeCircles.js
to Create a Dynamic Countdown Timer in PHP
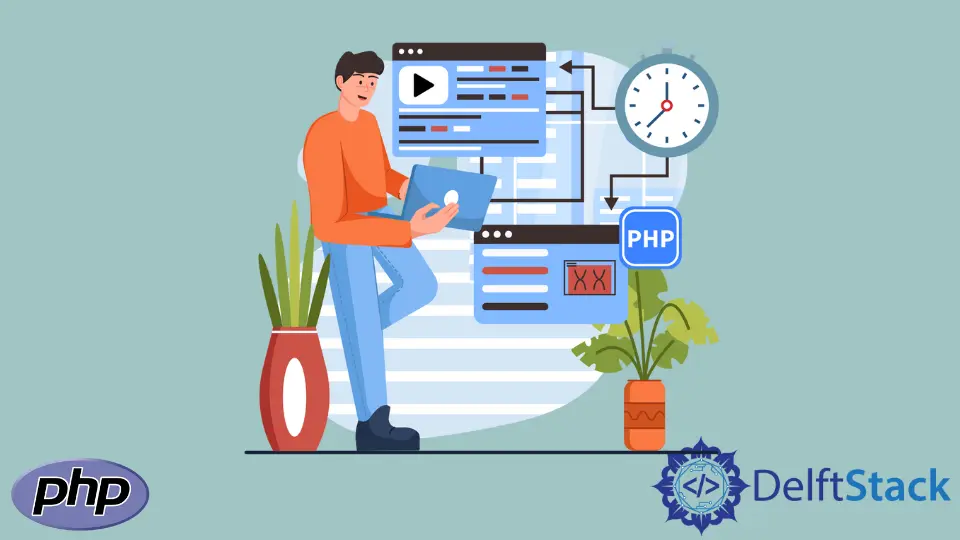
In this article, we will introduce a few methods to create a countdown timer in PHP. We can create a static countdown timer using only PHP. To make a dynamic countdown timer, we will have to use JavaScript.
Create a Static Countdown Timer in PHP
We can create a static countdown timer using PHP. The timer will only get updated when we refresh the page. Otherwise, the timer will stay the same. PHP runs on the server, and the page should be refreshed when new information is needed from the server. The countdown timer works perfectly, but we have to refresh the web page whenever we see progress.
We will use the PHP function time()
to get the current time. We can find the difference between the target date and the current time, and format them in days, hours, minutes, and seconds. We will use the floor()
function while converting the time left into the other units. The function will round the result down to the nearest integer. As a result, the newly calculated unit of time will be accurate. The time()
function gives the current time in seconds since January 1 1970 00:00:00 GMT. We can use EpochConverter to convert the current date and time to the timestamps and vice versa.
For example, pick a target date and use the EpochConverter to convert the date into Epoch timestamps. Then, create a variable $waiting_day
and assign the timestamp to it. Then find the difference between $waiting_day
and the current time and store the result in a variable $time_left
. Now, calculate the total number of days dividing $time_left
with the total number of seconds in a day, 60*60*60
. Use the floor()
function while converting. Store the result in the $days
variable. Next, update the $time_left
variable to find the remaining hours in terms of seconds. For this, find the remainder while dividing $time_left
by 60*60*60
. Use the %
operator to find the remainder. Similarly, find the remaining hours, minutes, and seconds. To find the hours, divide $time_left
by 60*60
, and to find minutes, divide by 60
. The remaining timestamps will be in seconds. Finally, print all the variables where days, hours, minutes, and seconds are stored.
Thus, we created a static countdown timer using PHP.
Example Code:
<?php
$waiting_day = 1637210196;
$time_left = $waiting_day - time();
$days = floor($time_left / (60*60*24));
$time_left %= (60 * 60 * 24);
$hours = floor($time_left / (60 * 60));
$time_left %= (60 * 60);
$min = floor($time_left / 60);
$time_left %= 60;
$sec = $time_left;
echo "Remaing time: $days days and $hours hours and $min min and $sec sec left";
?>
Output:
Remaing time: 17 days and 23 hours and 51 min and 26 sec left
Use TimeCircles.js
to Create a Dynamic Countdown Timer in PHP
We can create a dynamic countdown timer using the TimeCircles.js
library in PHP. The countdown timer will update itself without refreshing the webpage. TimeCircles is a jQuery plugin that helps to create a countdown timer with ease. We can use CDN to include the TimeCircles.js
and jQuery in our script. The script tags are as follows.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/timecircles/1.5.3/TimeCircles.min.js" integrity="sha512-FofOhk0jW4BYQ6CFM9iJutqL2qLk6hjZ9YrS2/OnkqkD5V4HFnhTNIFSAhzP3x//AD5OzVMO8dayImv06fq0jA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
For example, create a div
with a data-date
attribute. Write PHP as the value of the attribute. Use the echo
function to display the target date 2019-02-12 07:25:14
. Next, set the id
of the div
as cd_timer
. Next, open the script
tag and write some jQuery. Write a function and select the id cd_timer
and call the TimeCircles()
function.
When we run the webpage from localhost, we can see a countdown timer showing the remaining days, hours, minutes, and seconds. In this way, we can create a dynamic countdown timer using TimeCircle.js
in PHP.
Example Code:
<div data-date="<?php echo '2019-02-12 07:25:14' ; ?>" id="cd_timer"></div>
<script>
$(function () {
$("#cd_timer").TimeCircles();
});
</script>
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn