How to Create and Get PHP Class Constants
-
Use the
const
Keyword to Create Class Constant in PHP - Use the Access Modifiers to Create Constants in PHP
-
Use the
final
Keyword to Create Constants in PHP - Use the Static Variables to Emulate a Constant in PHP
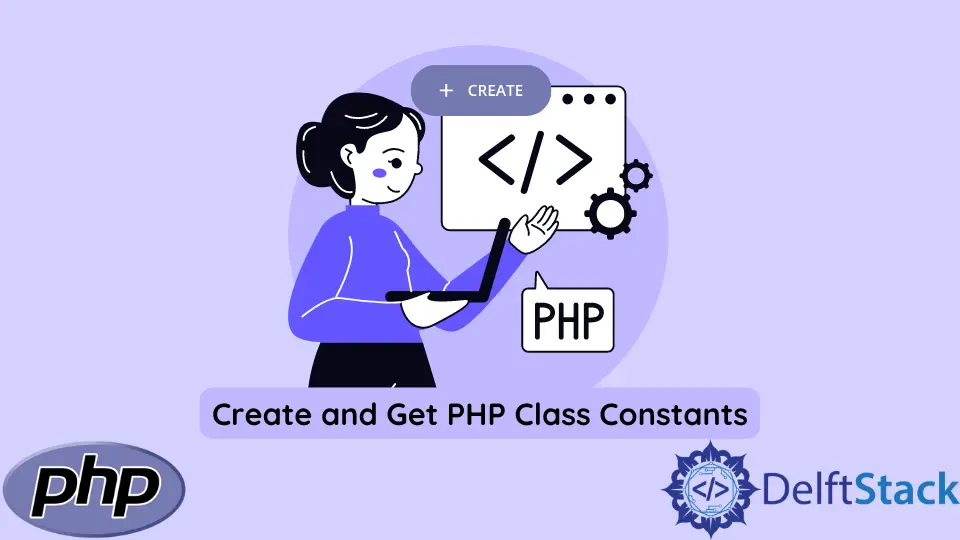
This tutorial will look at multiple code examples that will teach you how to create PHP class constants: the const
keyword, access modifiers, and the final
keyword. You’ll also learn how to emulate a constant with static variables.
Use the const
Keyword to Create Class Constant in PHP
PHP has the const
keyword. You make use of this keyword to create constants.
Once created, you can access the constant via the Scope Resolution Operator ::
.
In our next example, we define a class.
Within this class, we have a constant. Also, a function accesses this constant via the Scope Resolution Operator.
We can access it directly from the class name with the Scope Resolution Operator to show the constant.
<?php
class ClassWithConstant {
const CLASSCONSTANT = 23;
function show_constant() {
echo self::CLASSCONSTANT. "\n";
}
}
echo ClassWithConstant::CLASSCONSTANT;
?>
Output:
23
Also, you can create a new object. This object can reveal the constant in two ways.
- Call the
show_constant
function to reveal the constant. - Reveal the constant via Scope Resolution Operator
::
.
You’ll find both options in the next code block.
<?php
class ClassWithConstant {
const CLASSCONSTANT = 23;
function show_constant() {
echo self::CLASSCONSTANT. "\n";
}
}
$new_object = new ClassWithConstant();
// Call the inner function
// to reveal the constant
$new_object->show_constant();
// Or, access the constant via scope
// resolution
echo $new_object::CLASSCONSTANT;
?>
Output:
23
23
A final option is to assign the class name to a string variable then access the constant via Scope resolution.
<?php
class ClassWithConstant {
const CLASSCONSTANT = 23;
function show_constant() {
echo self::CLASSCONSTANT. "\n";
}
}
$class_name = "ClassWithConstant";
echo $class_name::CLASSCONSTANT. "\n";
Output:
23
Use the Access Modifiers to Create Constants in PHP
PHP’s access modifiers are public
, private
, and protected
. Available from PHP 7.1, you can create constants with these access modifiers.
Also, any constant declared with the private
modifier cannot be accessed outside of that class.
Our next code example defines two constants with public
and private
access modifiers. We can access the public constant via the Scope Resolution Operator.
Any attempt to access the private constant
results in a fatal error.
<?php
class ClassWithConst {
// As of PHP 7.1.0
public const PUBLICCONSTANT = 'Public Constant';
private const PRIVATECONSTANT = 'Private Constant';
}
echo ClassWithConst::PUBLICCONSTANT . "\n";
?>
Output:
Public Constant
Use the final
Keyword to Create Constants in PHP
With the final
keyword, you can emulate a public constant. Due to the final
keyword, the class scope will restrict this constant thanks.
Also, you can write the final
keyword before the class declaration. By doing this, the final
keyword will prevent inheritance.
The next code shows how to create constants with the final
keyword. First, there is a final
keyword before the first class definition.
Then, a function calls the class definition in the second class definition. Which in turn returns the constant.
<?php
final class ClassWithConstants {
public function getConstant()
{
return 'CONSTANT VALUE';
}
}
class CallClassConstant {
static public function ReturnClassConstant()
{
return new ClassWithConstants();
}
}
$constant_value = CallClassConstant::ReturnClassConstant()->getConstant();
echo $constant_value;
?>
Output:
CONSTANT VALUE
Use the Static Variables to Emulate a Constant in PHP
By their name, static variables are not meant to change. Hence, once assigned, you cannot change them.
It’s like the behavior of constants because you’ll want them to remain unchanged once you assign them their values.
In the following code example, there is a class definition. This class has a static variable set to null
.
Also, it has an access modifier that prevents outside access. Within the class, we have a function that returns the static variable once it’s assigned.
This function will ignore any further attempt to reassign the static variable. As a result, it will always return the first assigned value.
<?php
class ClassWithStaticVars {
private static $static_variable = null;
public static function returnStaticVariable($static_value = null)
{
if (
(is_null(self::$static_variable) === true)
&& (isset($static_value) === true)
) {
self::$static_variable = $static_value;
}
return self::$static_variable . "\n";
}
}
echo ClassWithStaticVars::returnStaticVariable();
echo ClassWithStaticVars::returnStaticVariable(200);
echo ClassWithStaticVars::returnStaticVariable("Hello");
echo ClassWithStaticVars::returnStaticVariable();
?>
Output:
200
200
200
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn