How to Read if Checkbox Is Checked in PHP
-
Use the
isset()
Function on$_POST
Array to Read if Checkbox Is Checked -
Use the
in_array()
Function to Read if the Checkbox Is Checked for Checkboxes as an Array -
Use the
isset()
Function With Ternary Function to Read if the Checkbox Is Checked
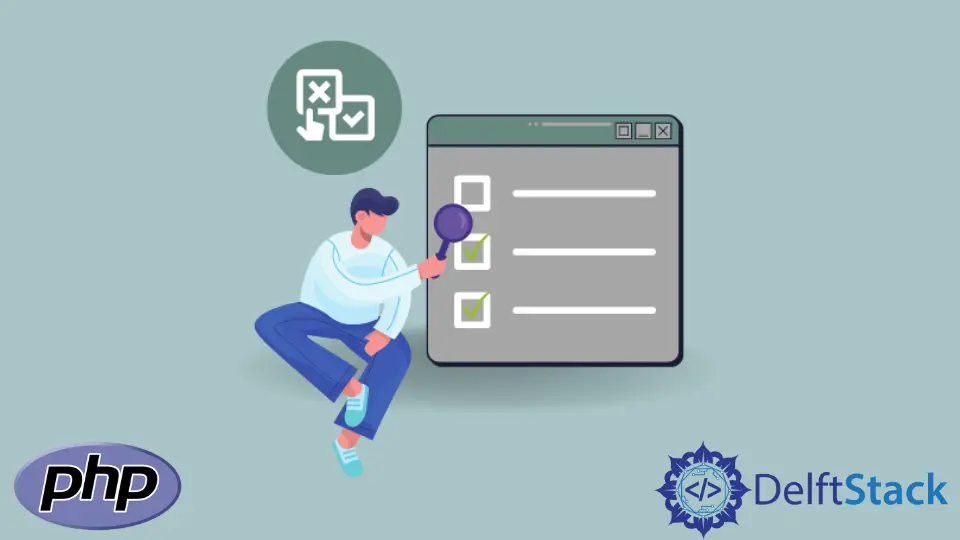
We will demonstrate how to check whether the checkbox is checked in PHP using the isset()
function on $_POST
array. We provide the value of the name
attribute of the input
tag of HTML as the array element in the $_POST
array.
We will introduce another method to read the checkbox if it is checked in PHP using the in_array()
function. We use checkboxes as an array in this method. It means that the all name
field in HTML input
tag must contain the same array.
We will introduce a short-hand method to check if the checkbox is checked using the ternary operator. The method is more straightforward and shorter and uses the isset()
function.
Use the isset()
Function on $_POST
Array to Read if Checkbox Is Checked
We can use the isset()
function to check whether the checkbox is checked in PHP. The isset()
function takes the $_POST
array as argument. The $_POST
array contains the specific value of the name
attribute present in HTML form.
For example, create a form in HTML with POST
method and specify the action to index.php
. Create two checkboxes with names test1
and test2
, respectively. Save the file with .php
extension. Create a PHP file named index.php
. Apply two if
conditions to isset()
function with $_POST
array as argument. Use test1
and test2
as the array elements in the $_POST
arrays, respectively. Print the message specifying the respective value has been checked.
The example below uses the POST
method to send the data in the form. It is secure while sending sensitive information through the form. Click here to know more about the POST
method. The user checks both the checkbox in the form. Thus, the script outputs the way it is shown below. If the user had checked only the Option 1
, the script would output as checked value1
. It goes similar to Option 2
too.
Example Code:
# html 5
<form action="index.php" method="post" >
<input type="checkbox" name="test1" value="value1"> Option 1
<input type="checkbox" name="test2" value="value2"> Option 2
<input type="submit" value="Submit">
</form>
#php 7.x
<?php
if(isset($_POST['test1'])){
echo "checked value1"."<br>";
}
if(isset($_POST['test2'])){
echo "checked value2";
}
?>
Output:
checked value1
checked value2
Use the in_array()
Function to Read if the Checkbox Is Checked for Checkboxes as an Array
We can use the in_array()
function to check whether an element lies within an array in PHP. The in_array()
function takes the value to be checked as the first argument. The second argument of the function is the array where the value is to be checked. Check the PHP manual to know more about the in_array
function. For this method to work, all the name
attribute values in HTML form must be an array.
For example, assign the value of name
attribute in HTML form with test[]
array. Note it applies to all the checkbox type
. First, in the PHP file, check whether the data has been submitted using the isset()
function as done in the first method. But, do not use the []
brackets after the test
while checking the posted data. Then, use in_array()
function to check whether the value1
is in the $_POST['test']
array. Display the message.
At first, the example below checks whether the data is submitted in the form. If the condition is true, then it checks if value1
lies in the $_POST['test']
array using the in_array()
function. The user checks the first checkbox in the form.
Example Code:
#html 5
<form action="index.php" method="post" >
<input type="checkbox" name="test[]" value="value1"> Option 1
<input type="checkbox" name="test[]" value="value2"> Option 2
<input type="submit" value="Submit">
#php 7.x
<?php
if(isset($_POST['test'])){
if(in_array('value1', $_POST['test'])){
echo "Option1 was checked!";
}
}
?>
Output:
Option1 was checked!
Use the isset()
Function With Ternary Function to Read if the Checkbox Is Checked
We can use a short-hand method to check if the checkbox has been checked in PHP. This method uses a ternary operator along with the isset()
function. Please check the MSDN Web Docs to know about the ternary operator.
For example, set a variable $check
to store the value of the ternary operation. Use the isset()
function to check whether test1
has been checked in the checkbox. Print $check
variable to show the result. In the example below, checked
is displayed if the condition is true, and the unchecked
is displayed if the condition is false. The user checks the second checkbox in the form. Therefore, the condition fails.
Example Code:
#html 5
<form action="index.php" method="post" >
<input type="checkbox" name="test1" value="value1"> Option 1
<input type="checkbox" name="test2" value="value2"> Option 2
<input type="submit" value="Submit">
</form>
#php 7.x
<?php
$check = isset($_POST['test1']) ? "checked" : "unchecked";
echo $check;
?>
Output:
unchecked
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn