How to Change Font Size and Color in PHP
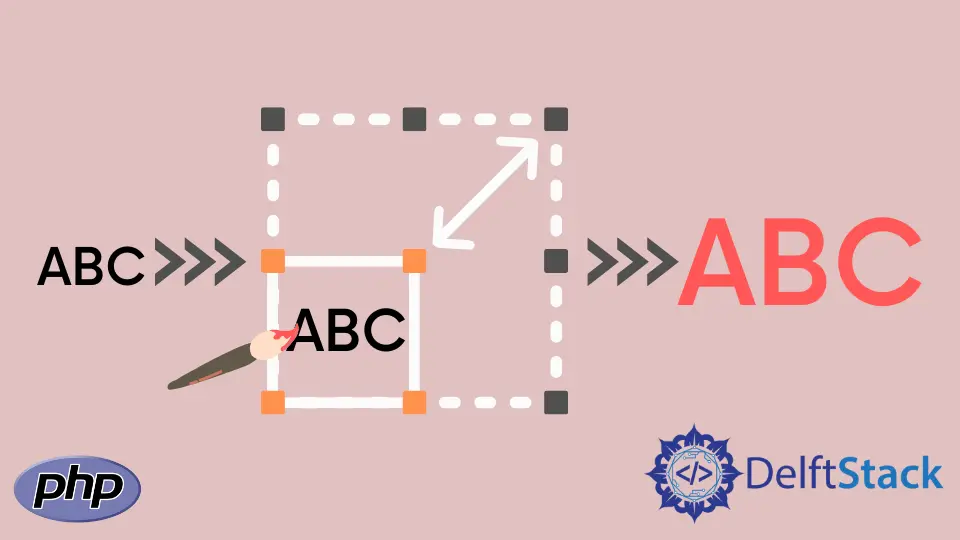
In this guide, we explore practical ways to utilize PHP for dynamic styling in web development, specifically focusing on how to change font size in PHP and color adjustments using CSS.
PHP doesn’t have built-in functionality for changing the font size or color. We can use HTML and CSS with PHP to change the font size and color.
This guide starts by introducing the use of inline CSS within HTML, showcasing how PHP can be used to dynamically apply styles based on specific conditions or data. It then progresses to a more advanced technique, which involves defining CSS in separate PHP files.
Through an examination of syntax, practical examples, and code analysis, the guide aims to offer developers comprehensive insights on how to effectively integrate PHP into their CSS styling processes.
Using Inline Style with HTML
One of the simplest ways to change the font size in a web page using PHP is by utilizing inline CSS styles within HTML elements. This method is particularly useful when you need to dynamically set the font size based on certain conditions processed in PHP.
Inline styles are CSS rules applied directly to HTML elements, providing a quick and straightforward way to style content. The PHP echo function plays a crucial role in dynamically setting the font size based on specific conditions processed in PHP.
Syntax
Inline CSS is written directly within HTML tags using the style
attribute. PHP can be embedded within this attribute to dynamically set CSS property values.
print '<tag style="property: ' . $phpVariable . ';">Content</tag>';
<tag>
: HTML tag where the style will be applied.
property
: CSS property (like font-size
or color
).
$phpVariable
: PHP variable containing the CSS property value.
Example:
<!DOCTYPE html>
<html>
<body>
<?php
$fontSize = '18px';
$fontColor = 'blue';
print '<p style="font-size: ' . htmlspecialchars($fontSize) . '; color: ' . htmlspecialchars($fontColor) . ';">This is dynamic text styled with PHP.</p>';
?>
</body>
</html>
Code Analysis:
In this PHP code snippet, we dynamically set inline CSS styles for an HTML paragraph element, showcasing how server-side scripting can influence the styling of web content.
We declare PHP variables, $fontSize
and $fontColor
, representing the desired font size and color. Using the print
function, we output an HTML paragraph with inline styles, dynamically embedding the values of these variables into the style
attribute.
The htmlspecialchars
function is employed to secure against potential XSS attacks by converting special characters to HTML entities.
Output:
Using CSS in a Separate File
In some scenarios, you might want to define your CSS styles within a PHP file, such as when you need to dynamically generate styles based on certain conditions or data. Using a PHP file like style.php
for CSS allows you to leverage PHP’s capabilities within your stylesheet.
This can be particularly useful for developers who prefer managing styles from the command line interface.
Syntax
Setting Content Type in PHP style.php
:
header("Content-type: text/css; charset: UTF-8");
This header function at the beginning of style.php
informs the browser that the file should be treated as a CSS file. The character set UTF-8
ensures proper encoding of the content.
Linking style.php
in HTML:
<link rel="stylesheet" type="text/css" href="style.php">
This HTML tag links style.php
as a stylesheet
in the document’s head section. Despite being a PHP file, it’s referenced like a regular CSS file, thanks to the content type header set in style.php
.
Example:
style.php
<?php
header("Content-type: text/css; charset: UTF-8");
$fontSize = '20px';
$fontColor = 'green';
?>
body {
font-size: <?php echo $fontSize; ?>;
color: <?php echo $fontColor; ?>;
}
index.php
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="style.php">
</head>
<body>
<?php
print 'This text is styled using PHP-generated CSS.';
?>
</body>
</html>
Code Analysis:
style.php
:
We begin by setting the content type to CSS in the file.
We then define the styles using PHP variables $fontSize
and $fontColor
.
Finally, we use PHP to dynamically assign values to the CSS rules for the body element.
index.php
:
We include style.php
as a stylesheet
, allowing us to leverage its defined rules.
And we utilize PHP’s print
function to display text, ensuring that it is automatically styled in accordance with the rules outlined in style.php
.
Output:
Using CSS classes
In web development, styling elements using CSS classes is a fundamental approach. PHP, primarily a server-side scripting language, can dynamically generate HTML content including these classes.
This section explores how to change font size and color using CSS classes in conjunction with PHP.
Syntax
Defining CSS Classes in the Head Tag:
<head>
<style>
.className {
/* CSS properties */
}
/* More classes */
</style>
</head>
CSS classes are defined inside a <style>
tag in the document’s head section. Each class can contain various CSS properties to style elements accordingly.
PHP Output with Dynamic Class Names:
print '<p class="' . $className . '">Content</p>';
PHP is used to dynamically generate HTML content. The print
function outputs a paragraph <p>
with a class attribute.
The class name is stored in the $className
variable, allowing for dynamic assignment of styles.
Example:
<!DOCTYPE html>
<html>
<head>
<style>
.large-text {
font-size: 24px;
color: blue;
}
.small-text {
font-size: 14px;
color: red;
}
</style>
</head>
<body>
<?php
$className = 'large-text';
print '<p class="' . $className . '">This is large blue text.</p>';
$className = 'small-text';
print '<p class="' . $className . '">This is small red text.</p>';
?>
</body>
</html>
Code Analysis:
In our code analysis, we first define two CSS classes within the <head>
section of the HTML document: .large-text
and .small-text
. These classes are equipped with specific font size and color properties – large-text
for larger, blue text and small-text
for smaller, red text.
We can also use specific color codes font color within these classes to ensure consistent and accurate color representation across different elements of our webpage.
Next, in the PHP script, we dynamically assign these class names to HTML paragraph elements using the print
function. By changing the value of the $className
variable, we control which CSS class is applied to each paragraph.
This approach demonstrates how we can effectively combine PHP and CSS to dynamically render styled text in a webpage, showcasing the flexibility and power of PHP in manipulating HTML content.
Output:
Conclusion
In wrapping up our exploration of PHP and CSS integration, we’ve uncovered valuable techniques for dynamically styling web content. From inline CSS applications within HTML to the utilization of PHP-driven stylesheets, the guide offers practical examples and clear code analysis.
By focusing on font size and color adjustments, we’ve addressed specific challenges faced by developers seeking dynamic and responsive web solutions. As you implement these strategies into your projects, the symbiotic relationship between PHP and CSS becomes apparent, providing a solid foundation for efficient and visually compelling web development.
To broaden your styling capabilities, we recommend exploring other CSS properties such as text align. This property allows you to control the alignment of text within HTML elements, adding a layer of customization to your web design.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook