How to Call to Undefined Function in PHP
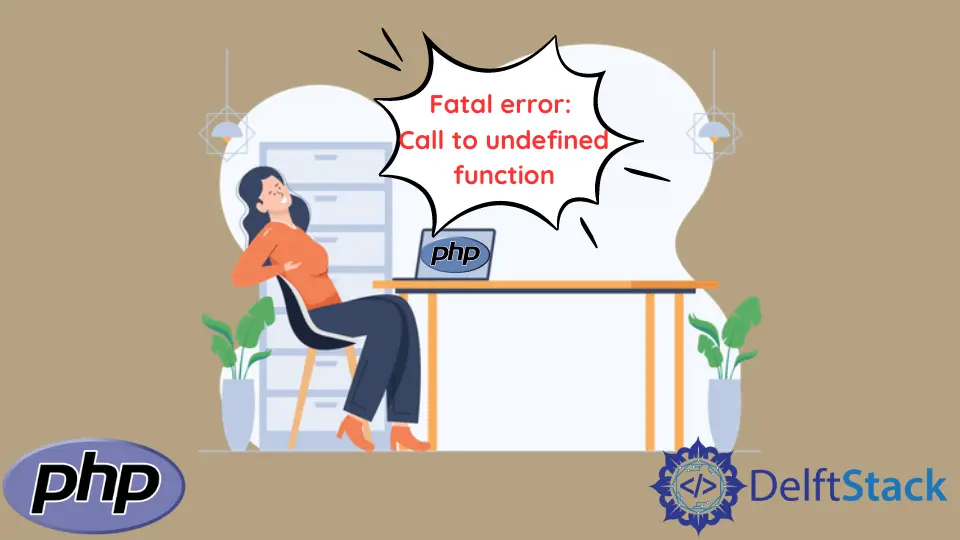
Many of you have encountered this error several times Fatal error: Call to undefined function function_name()
. In today’s post, we are finding out how to unravel this error. But before we solve this problem, let’s understand how PHP evaluates the functions.
There are several ways to define functions and call them. Let’s say you write it in the function.php file and call it in the main.php file.
// function.php
<?php
namespace fooNamespace {
function foo() {
return "Calling foo"
}
}
?>
// main.php
include function.php
<?php
echo fooNamespace\foo();
?>
Namespaces are qualifiers that enable better management by grouping classes that work together to perform a task. It allows you to use the same name for multiple classes. It is important to know how PHP knows which element of the namespace is required by the code. PHP namespaces work quite a sort of a filesystem. There are 3 ways to access a file in a file system:
- Relative file name such as
fooBar.txt
. It will resolve tofooDirectory/fooBar.txt
where fooDirectory is the directory currently busy directory. - Relative path name such as
subdirectory/fooBar.txt
. It will resolve tofooDirectory/subdirectory/fooBar.txt
. - Absolute path name such as
/main/fooBar.txt
. It will resolve to/main/fooBar.txt
.
Namespaced elements in PHP follow an equivalent principle. For example, a class name can be specified in three ways:
- Unqualified name/Unprefixed class name:
Or,
$a = new foo();
If the current namespace isfoo::staticmethod();
foonamespace
, it will always resolve tofoonamespace\foo
. If the code is a global, non-namespaced code, this resolves tofoo
. - Qualified name/Prefixed class name:
Or,
$a = new fooSubnamespace\foo();
If the present namespace isfooSubnamespace\foo::staticmethod();
foonamespace
, it will always resolve tofoonamespace\fooSubnamespace\foo
. If the code is global, non-namespaced code, this resolves tofooSubnamespace\foo
. - Fully qualified name/Prefixed name with global prefix operator:
Or,
$a = new \foonamespace\foo();
This always resolves to the literal name laid out in the code,\foonamespace\foo::staticmethod();
foonamespace\foo
.
Now suppose you define a class & call the method of a class within the same namespace.
<?php
class foo {
function barFn() {
echo "Hello foo!"
}
function bar() {
barFn();
// interpreter is confused which instance's function is called
$this->barFn();
}
}
$a = new foo();
$a->bar();
?>
$this
pseudo-variable has the methods and properties of the current object. Such a thing is beneficial because it allows you to access all the member variables and methods of the class. Inside the class, it is called $this->functionName()
. Outside of the class, it is called $theclass->functionName()
.
$this
is a reference to a PHP object
the interpreter created for you, which contains an array of variables. If you call $this
inside a normal method in a normal class, $this
returns the object to which this method belongs.
Steps to Resolve the Error of Calling to Undefined Function in PHP
-
Verify that the file exists. Find the PHP file in which the function definition is written.
-
Verify that the file has been included using the
require
(orinclude
) statement for the above file on the page. Check that the path in therequire
/include
is correct. -
Verify that the file name is spelled correctly in the
require
statement. -
Print/echo a word in the included file to ascertain if it has been properly included.
-
Defined a separate function at the end of the file and call it.
-
Check functions are closed properly. (Trace the braces)
-
If you are calling methods of a class, make sure
$this->
is written.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn