Encode Image to Base64 in PHP
-
Encode Image to Base64 With
file_get_contents
,pathinfo
, andbase64_encode
in PHP -
Encode Image to Base64 With
file_get_contents
andbase64_encode
in PHP -
Encode Image to Base64 With
base64_encode
andmime_content_type
in PHP - Encode Image to Base64 With a Custom Function in PHP
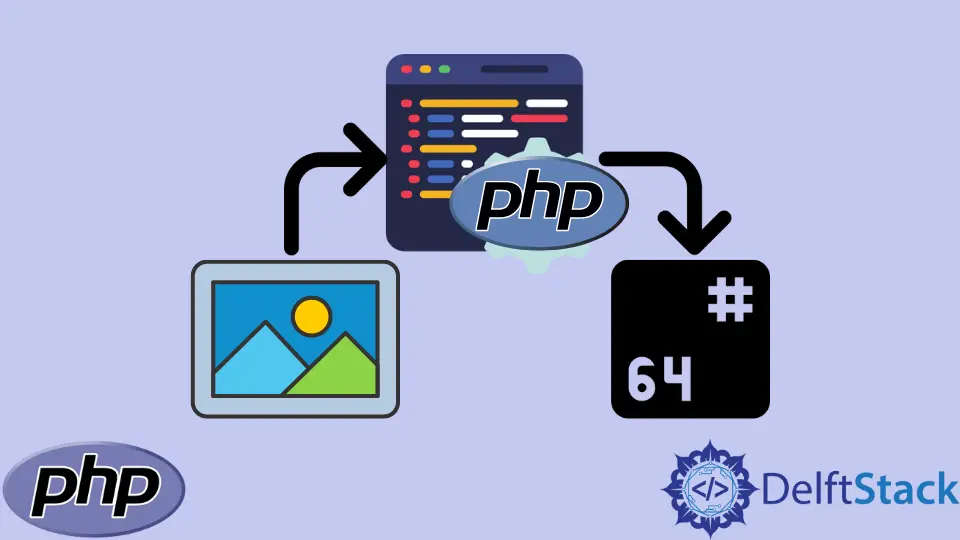
There are many ways to encode an image to base64 format with the help of multiple built-in functions in PHP.
The functions includes:
Encode Image to Base64 With file_get_contents
, pathinfo
, and base64_encode
in PHP
The image should have a defined path stored in a variable to encode an image to base64 with file_get_contents
, pathinfo
, and base64_encode
.
Use the pathinfo
function to read the information. Then pass the resulting information to the file_get_contents
function.
The job of file_get_contents
is to read the image file into a string. We can pass the string to the base64_encode
function.
To show the base64 encoded image, concatenate the string data:image/
, the information from pathinfo
, the string ;base64
, and the base64 encoded string.
<?php
// Define a path for the image
$image_path = 'xps-unsplash.jpg';
// Check the image type
$image_type = pathinfo($image_path, PATHINFO_EXTENSION);
// Read the image into a string
$data = file_get_contents($image_path);
// Encode the image
$base64_encode = 'data:image:/' . $image_type . ';base64,' . base64_encode($data);
// Return the first 50 characters of the
// base64 encoded string
echo substr($base64_encode, 0, 50);
?>
Output:
data:image:/jpg;base64,/9j/4AAQSkZJRgABAQEASABIAAD
Encode Image to Base64 With file_get_contents
and base64_encode
in PHP
To convert an image to base64, you pass the path of the image directly to the file_get_contents
function.
The file_get_contents
function will return a string. It would help if you supplied this string as an argument to the base64_encode
function.
<?php
// Define a path for the image
$data = file_get_contents('xps-unsplash.jpg');
// Encode the image
$base64_encode = base64_encode($data);
// Return the first 50 characters of the
// base64 encoded string
echo substr($base64_encode, 0, 50);
?>
Output:
/9j/4AAQSkZJRgABAQEASABIAAD/4gIcSUNDX1BST0ZJTEUAAQ
Encode Image to Base64 With base64_encode
and mime_content_type
in PHP
Use the file_get_contents
function to read the image path. Then convert the resulting string to base64 encoding.
You can format the image source before passing it to the src
tag in the HTML <img>
tag.
To do this, you need to format the image as data:{mime};base64,{data}
. You can deduce that you’ll need an image mime type from the format.
You can get the image mime type with the mime_content_type
function. In the end, you pass the format as the value of the src
attribute of the <img>
tag.
<?php
// Define a path for the image
$image_path = 'xps-unsplash.jpg';
// Read the image path and convert it to
// base64
$base64_encode = base64_encode(file_get_contents($image_path));
// Prepare the image for use in an img
// src tag
$image_source = 'data: ' . mime_content_type($image_path) . ';base64,' . $base64_encode;
// Display the image in the img src tag
echo '<img src="' . $image_source . '">';
?>
Output:
Encode Image to Base64 With a Custom Function in PHP
Within the definition of this function, you can use the likes of file_get_contents
, base64_encode
, and pathinfo
to handle the image encoding.
You can implement a cache functionality with file_put_contents
for better performance.
<?php
function encode_html_image (
$image_file,
$alternate_text = NULL,
$image_cache,
$image_extension = NULL
) {
// check if the image is not a file
if (!is_file($image_file)) {
return false;
}
// Save the image as base64 extension for
// use as a cache
$image_with_base64_extension = "{$image_file}.base64";
if ($image_cache && is_file($image_with_base64_extension)) {
// Use the cache image
$base64_encoded_image = file_get_contents($image_with_base64_extension);
} else {
// Create a new base64 encoded image
$image_binary_data = file_get_contents($image_file);
$base64_encoded_image = base64_encode($image_binary_data);
if ($image_cache) {
file_put_contents($image_with_base64_extension, $base64_encoded_image);
}
}
$image_extension = pathinfo($image_file, PATHINFO_EXTENSION);
return "<img alt='{$alternate_text}' src='data:image/{$image_extension};base64,{$base64_encoded_image}' />";
}
$sample_image = 'xps-unsplash.jpg';
$encoded_image = encode_html_image($sample_image, 'XPS by DELL', true);
echo $encoded_image;
?>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn