How to Find Base URL in PHP
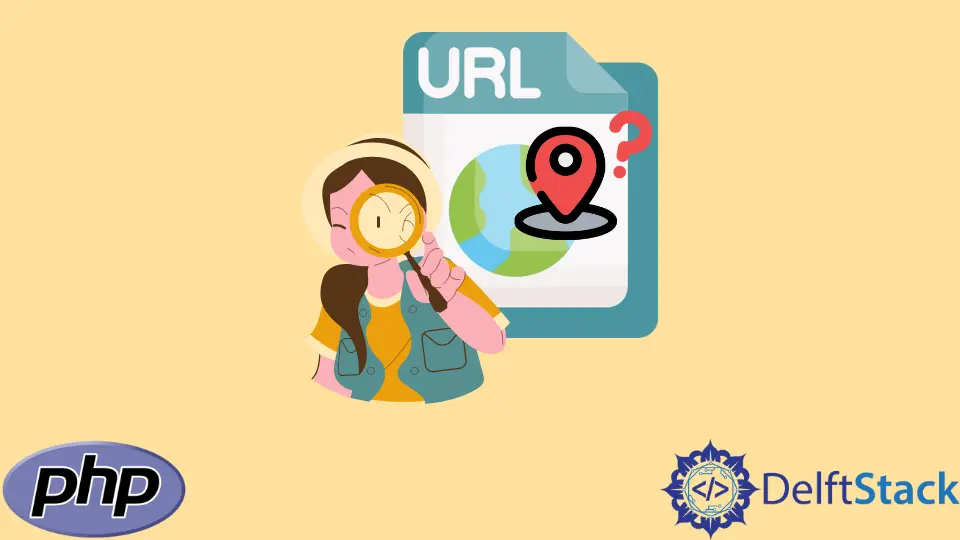
This article will introduce a few methods to find the base URL of an application in PHP.
Use the $_SERVER
Superglobal Variable to Find the Base URL in PHP
The $_SERVER
superglobal variable in PHP contains the information about the server and the execution environment.
The term superglobal variable signifies that the variable can be accessed in every scope in the program. The variable is a predefined array and contains a wide range of indices.
We can use the various indices to find paths, headers, and script locations. A few examples of the available indices used in the $_SERVER
array are SERVER_NAME
, SERVER_ADDR
, HTTP_HOST
, REMOTE_HOST
, etc.
The web servers provide these indices. So, the availability of the indices varies according to the webserver. An example of using the $_SERVER
array is shown below.
<?php
echo $_SERVER['SERVER_NAME'];
?>
Output:
localhost
In the example above, the server used is localhost. The $_SERVER['SERVER_NAME]
array returns the server hostname under which the current script is executing.
We can use the $_SERVER
array to find the base URL of the application in PHP. The SERVER_NAME
and REQUEST_URI
indices are useful for finding the base URL.
The REQUEST_URI
index returns the Unifrom Resource Identifier (URI) of the current web page.
For example, create a variable $server
and store $_SERVER['SERVER_NAME']
in it. Likewise, store $_SERVER['REQUEST_URI']
in the $uri
variable.
Next, concatenate the string http://
with the variables $server
and $uri
and print them using the print
function.
As a result, we can see the base URL of the current application. The example below shows the path of the current application as it is run in the localhost
.
$server = $_SERVER['SERVER_NAME'];
$uri = $_SERVER['REQUEST_URI'];
print "http://" .$server. $uri;
Output:
http://localhost/my_app/
We can also get the hostname using the HTTP_HOST
index in the $_SERVER
array. The index HTTP_HOST
returns the host header of the current request in the application.
The difference between it and the SERVER_NAME
index is that HTTP_HOST
retrieves the header from the client request while SERVER_NAME
retrieves the information defined at the server configuration.
The SERVER_NAME
index is more reliable than the HTTP_HOST
as the value is returned from the server and cannot be modified. The example of the HTTP_HOST
index to get the base URL of the application is shown below.
$host = $_SERVER['HTTP_HOST'];
$uri = $_SERVER['REQUEST_URI'];
print "http://" .$host. $uri;
Output:
http://localhost/my_app/
Above is how we can find the base URL of an application in PHP.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn