PHP AES Encrypt Decrypt
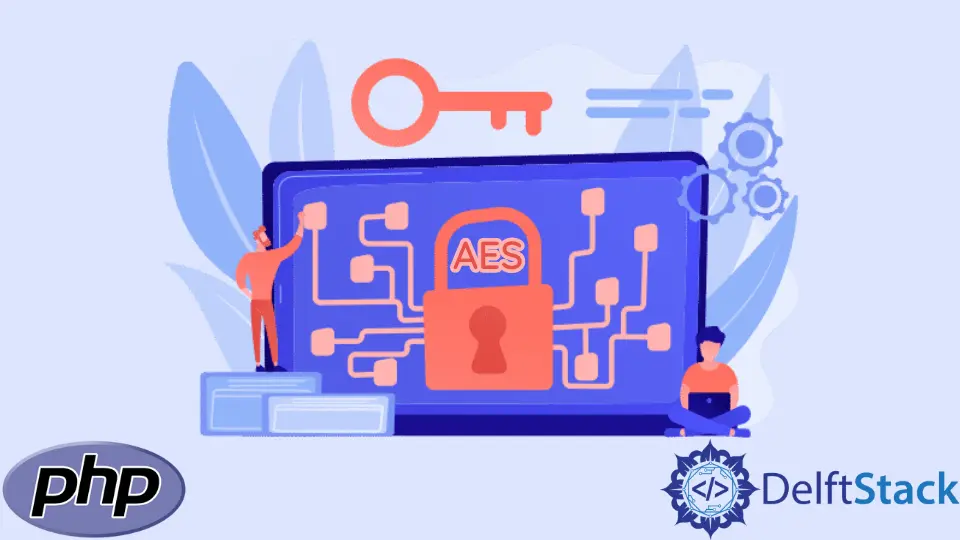
PHP has a built-in extension to encrypt and decrypt strings using PHP’s AES
method.
The function openssl_encrypt()
is used to encrypt the strings and openssl_decrypt()
is used to decrypt the strings.
Use the Open SSL Functions to Encrypt and Decrypt Strings in PHP
The openssl_encrypt()
and openssl_decrypt()
takes a set of mandatory and optional parameters, the information about the parameters is given in the table below:
Parameter | Description |
---|---|
data |
Plain text/String |
cipher_algo |
The cipher method, in our case, AES |
passphrase |
If the passphrase is shorter than the limit, it is silently padded with null characters and truncated if longer. |
options |
Bitwise disjunction of flags. OPENSSL_RAW_DATA and OPENSSL_ZERO_PADDING . |
iv |
Initialization vector, non-null |
tag |
The authentication tag, CGM , or CCM |
aad |
Additional authentication data. |
tag_length |
The length of the authentication tag is between 4 to 16 |
The openssl_encrypt()
takes all the parameters above and exclude aad
and tag_length
when using openssl_decrypt()
.
<?php
//Encryption
$original_string = "Hello! This is delftstack"; // Plain text/String
$cipher_algo = "AES-128-CTR"; //The cipher method, in our case, AES
$iv_length = openssl_cipher_iv_length($cipher_algo); //The length of the initialization vector
$option = 0; //Bitwise disjunction of flags
$encrypt_iv = '8746376827619797'; //Initialization vector, non-null
$encrypt_key = "Delftstack!"; // The encryption key
// Use openssl_encrypt() encrypt the given string
$encrypted_string = openssl_encrypt($original_string, $cipher_algo,
$encrypt_key, $option, $encrypt_iv);
//Decryption
$decrypt_iv = '8746376827619797'; //Initialization vector, non-null
$decrypt_key = "Delftstack!"; // The encryption key
// Use openssl_decrypt() to decrypt the string
$decrypted_string=openssl_decrypt ($encrypted_string, $cipher_algo,
$decrypt_key, $option, $decrypt_iv);
//Display Strings
echo "The Original String is: <br>" . $original_string. "<br><br>" ;
echo "The Encrypted String is: <br>" . $encrypted_string . "<br><br>";
echo "The Decrypted String is: <br>" . $decrypted_string;
?>
The code above first encrypts the string using the AES
method and then decrypts it.
Output:
The Original String is:
Hello! This is delftstack
The Encrypted String is:
21tZwb2Wrw2gPGid29Bfy7TacU1bEmCbaw==
The Decrypted String is:
Hello! This is delftstack
AES has different cipher_algorithams
based on method and number of bits for example aes-128-cbc
, aes-192-cfb
, or aes-256-cbc
.
See all the options for AES encryption and other methods here.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook