How to Pass Variable From PHP to JavaScript
- Use AJAX to Pass the PHP Variable to JavaScript
- Use JavaScript to Escape the PHP Scripts to Pass the PHP Variable to JavaScript
-
Use the Short PHP Echo Tag,
<?=?>
Inside the JavaScript to Pass the PHP Variable to JavaScript
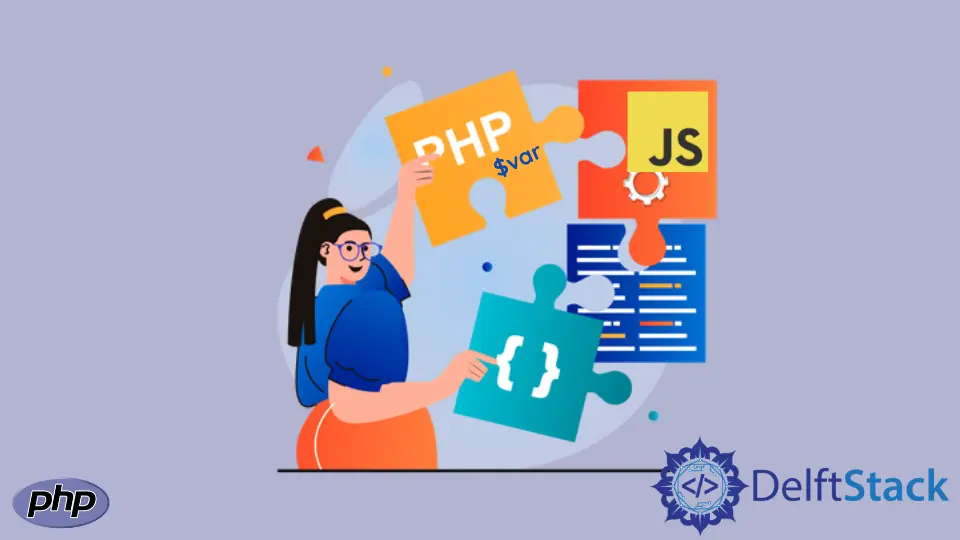
We will introduce a way to pass the PHP variable to JavaScript using AJAX. This method requests the data from the PHP server and runs on the client machine.
We will also demonstrate another method to pass the PHP variable in JavaScript by escaping the PHP to JavaScript. We write JavaScript after the PHP scripts to escape the PHP.
We will show you another example to pass the PHP variable to JavaScript using the short echo tag in the JavaScript as <?=$var?>
where $var
is the PHP variable.
Use AJAX to Pass the PHP Variable to JavaScript
We can use AJAX to get the data and variables from the PHP server to JavaScript. This method has separate server-side and client-side scripts. It makes the code cleaner and enhances the code readability. AJAX stands for Asynchronous JavaScript and XML. It uses the XMLHttpRequest
object to request the data from the server. It displays the data using JavaScript and HTML DOM. This process occurs asynchronously, which means the changes happen in the webpage without the page being reloaded. However, there is latency when AJAX is used over networks because it sends the HTTP request. In this method, we write JavaScript code in the PHP file.
For example, create a PHP file named index.php
. Write the JavaScript code inside the <script>
tag. Create a vairable req
using the var
keyword. Create a request object of the XMLHttpRequest()
function and assign it to the req
variable. Call the onload()
function with the req
object using the function expression. Inside the function, use the console.log()
function to log the this.responseText
property. Do not forget to end the function expression with a semicolon. Use the req
object to call the open()
function. Supply get
, get-data.php
and true
simultaneously as the parameters in the function. Call the send()
function with the req
object. Create another PHP file named get-data.php
. Assign a value of Mountain
to $var
variable. Echo the json_encode()
function with the $var
as the parameter.
In the example below, we created a request object with the XMLHttpRequest()
function. The onload()
function handles the operation to be performed during the response. The open()
function specifies the HTTP method, the PHP file that sends the JSON response, and a boolean value that controls the rest of the execution of the script. The json_encode()
function in the get-data.php
file sends the response in JSON format. Here, we printed the variable $var
in the console.
Example Code:
# php 7.*
<?php
?>
<script>
var req = new XMLHttpRequest();
req.onload = function() {
console.log(this.responseText);
};
req.open("get", "get-data.php", true);
req.send();
</script>
# php 7.*
<?php
$var = "Mountain";
echo json_encode($var);
?>
Output:
"Mountain"
Use JavaScript to Escape the PHP Scripts to Pass the PHP Variable to JavaScript
We can use JavaScript after the PHP tags to escape the PHP scripts. We can create a PHP variable in the PH P script. Then, we can write the JavaScript to escape the above-written PHP and pass the variable inside the JavaScript code. Inside the JavaScript, we can use the PHP tag to echo the PHP variable assigning it to a JavaScript variable.
For example, assign a value Metallica
to a variable $var
inside the PHP tags. Write a script
tag and inside it, write a PHP tag. Inside the PHP tag, echo a JavaScript variable jsvar
. Assign the PHP variable $var
to jsvar
. Do not forget a quote wrapped around the $var
and a semicolon after it. Close the PHP tag and log the value of jsvar
using the console.log()
function. Close the script
tag. In the example below, we have logged the PHP variable $var
in the console using JavaScript. We can see the output in the console of the webpage.
Example Code:
#php 7.x
<?php
$var = 'Metallica';
?>
<script>
<?php
echo "var jsvar ='$var';";
?>
console.log(jsvar);
</script>
Output:
Metallica
Use the Short PHP Echo Tag, <?=?>
Inside the JavaScript to Pass the PHP Variable to JavaScript
We can use the short PHP echo tag inside the JavaScript to pass the PHP variable to JavaScript. We can use the short PHP echo tag to get rid of the hassle of writing the PHP tag and the echo
statement. It is a short-hand method. This method is very much similar to the second method in terms of the concept and the execution.
For example, assign a value Megadeth
to a variable $var
inside the PHP tags. Open a script
tag and create a JavaScript variable jsvar
. Write the short-hand echo tag <?=$var?>
as the value of the jsvar
variable. Log the variable using the console.log()
function. We can view the output of the script in the console of the webpage.
Code Example:
#php 7.x
<?php
$var = 'Megadeth';
?>
<script>
var jsvar = '<?=$var?>';
console.log(jsvar);
</script>
?>
Output:
Megadeth
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn