How to Execute PHP Function With onclick
-
Use jQuery to Execute the PHP Function With the
onclick()
Event -
Use Plain JavaScript to Execute the PHP Function With the
onclick()
Event -
Use the
GET
Method and theisset()
Function to Execute a PHP Function From a Link
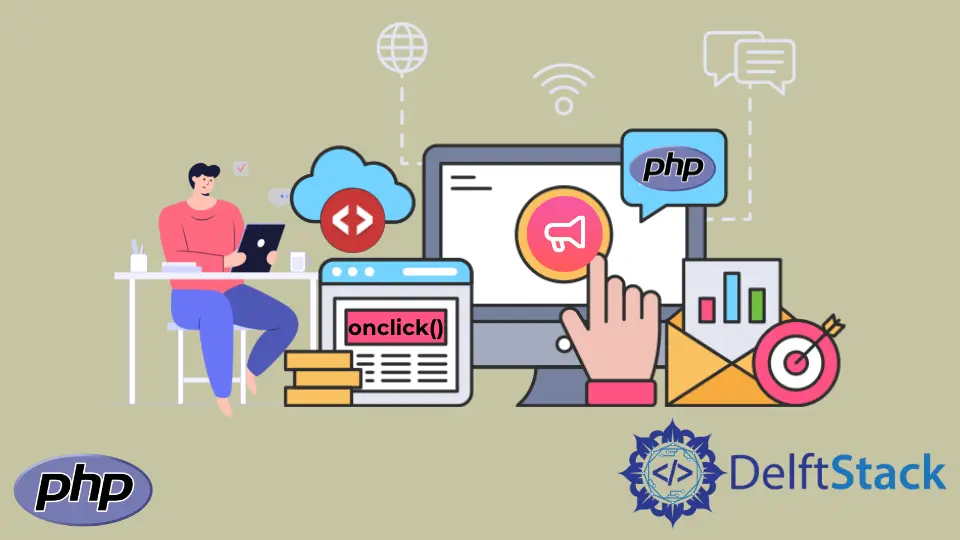
We will also introduce another method to execute a PHP function with the onclick()
event using the jQuery library. This method calls a JavaScript function which will output the contents of the PHP function in the webpage.
We will also demonstrate another method to execute a PHP function with the onclick()
event using plain JavaScript to call the PHP function.
This article will introduce a method to execute a PHP function using the GET
method to send the data in the URL and the isset()
function to check the GET
data. This method calls the PHP function if the data is set and executes the function.
Use jQuery to Execute the PHP Function With the onclick()
Event
We can use jQuery to execute the onclick()
event by writing a function that will execute the PHP function. For example, create a PHP file echo.php
and write a function php_func()
. Write a message Have a great day
inside the function and call the function. In another PHP file, write some jQuery inside the script
tag. Do not forget to link the webpage with the jQuery source. In the HTML, write a button
tag with onclick()
attribute. Write the value of the attribute as the test()
function. Write the text Click
between the button
tags. Create an empty div
tag below the button. Write the function test()
inside the script
tag. Write an AJAX method with the URL as echo.php
and write a success()
function with result
as the parameter. Then use the selector to select the div
tag and use the text()
function with result
as the parameter.
In the example below, we use the AJAX method to perform an asynchronous HTTP request. The URL species the URL to send the request to, and the success()
function runs when the request is successful. The method sends the request to the echo.php
file, which resides in the same location as the current PHP file. The request becomes successful, and the success()
function returns the result, and it gets printed.
Example Code:
#php 7.x
<?php
function php_func(){
echo " Have a great day";
}
php_func();
?>
<script>
function test(){
$.ajax({url:"echo.php", success:function(result){
$("div").text(result);}
})
}
</script>
<button onclick="test()"> Click </button>
<div> </div>
Output:
Have a great day
Use Plain JavaScript to Execute the PHP Function With the onclick()
Event
This method uses JavaScript to execute a PHP function with onclick()
event. For example, write a PHP function php_func()
that displays the message Stay Safe
. Create a button with the name Click
using the button
tag. Specify the onclick()
function as an attribute with the clickMe()
function as its value. Write the function clickMe()
inside the script
tag. Create a variable result
and call the php_func()
in it inside the PHP tags. Use the document.write()
function with the result
as the parameter to print the output.
In the example below, the JavaScript function clickMe()
executes when we click the button. Then, the PHP function php_func()
executes from the JavaScript function. The result
variable stores the result from the PHP function, and it gets printed.
Code Example:
#php 7.x
<?php
function php_func(){
echo "Stay Safe";
}
?>
<button onclick="clickMe()"> Click </button>
function clickMe() {
var result = '<?php php_func(); ?>'
document.write(result);
}
Output:
Stay Safe
Use the GET
Method and the isset()
Function to Execute a PHP Function From a Link
We can set the URL of a link with the GET
data and check whether the data has been set with the isset()
function. We can create a PHP function and call the function if the data has been set. For example, write a function myFunction()
and display a message saying Have a great day
inside the function. Create a link using the anchor tag. Set the href
attribute of the tag as index.php?name=true
. Write a text Execute PHP Function
between the anchor tags. Check if name
is set using the isset()
function with the $_GET
variable. Call the function myFunction()
inside the if
block.
In the example below, the GET
data is sent through the URL. The value of name
is set to true
. The isset()
function returns true and the function myFunction()
executes and displays the message.
Example Code:
# php 7.x
<!DOCTYPE HTML>
<html>
<?php
function myFunction() {
echo 'Have a great day'.'<br>';
}
if (isset($_GET['name'])) {
myFunction();
}
?>
<a href='index.php?name=true'>Execute PHP Function</a>
</html>
Output:
Have a great day
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn