PHP Warning Invalid Argument Supplied for foreach()
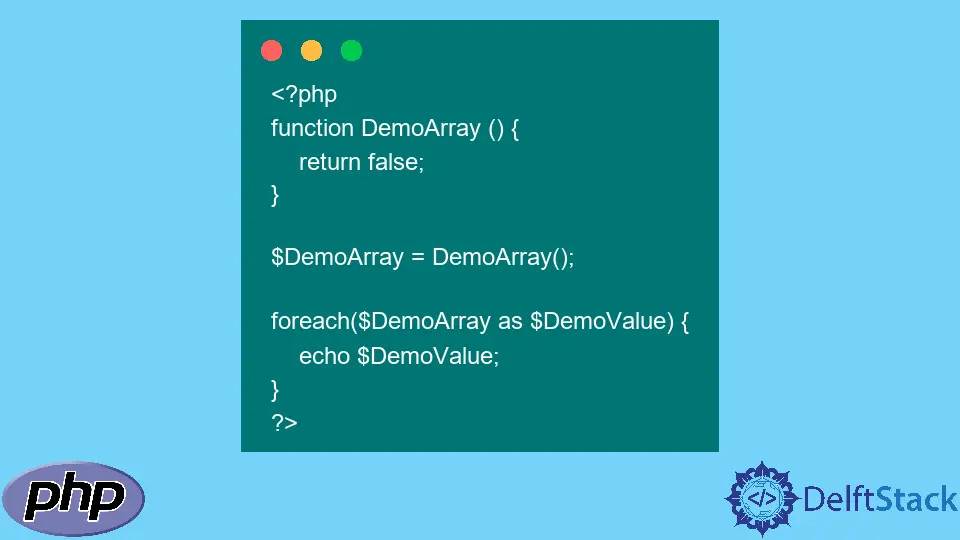
This tutorial demonstrates how to fix the PHP Warning: Invalid argument supplied for foreach()
.
PHP Warning: Invalid Argument Supplied for Foreach()
When using the foreach
loop in PHP, sometimes the warning Warning: Invalid argument supplied for foreach()
may occur. This warning occurs when we provide an invalid argument for the foreach
loop.
The common reason for this error is when we try to pass a string as a value to the foreach
loop. The foreach
loop is used to iterate over an array, but when we provide a value that is not an array, it will throw the Warning: Invalid argument supplied for foreach()
.
Let’s try a simple example that throws this error:
<?php
$DemoString = "Hello! This is delftstack.com";
foreach($DemoString as $DemoValue){
echo $DemoValue;
}
?>
The above code will throw the Invalid argument supplied for foreach()
error because the foreach()
cannot iterate over a string.
See the output:
Warning: Invalid argument supplied for foreach() in C:\Apache24\htdocs\demo.php on line 3
We must provide an array to the foreach
loop to fix this issue. Let’s try to fix this example:
<?php
$DemoString = array("Hello!", "This", "is", "delftstack.com");
foreach($DemoString as $DemoValue){
echo $DemoValue."<br>";
}
?>
Now, as we changed the string to an array, this warning should be fixed. See the output:
Hello!
This
is
delftstack.com
The Invalid argument supplied for foreach()
doesn’t only occur with string, but any value which is not iterable like the array will throw the same warning.
Here is another example where we try to iterate over the return value of a function:
<?php
function DemoArray () {
return false;
}
$DemoArray = DemoArray();
foreach($DemoArray as $DemoValue) {
echo $DemoValue;
}
?>
As we can see, the DemoArray()
function is returning false, and we try to assign it to a variable and iterate over it using the foreach()
loop. It should throw the Invalid argument supplied for foreach()
warning.
See the output:
Warning: Invalid argument supplied for foreach() in C:\Apache24\htdocs\demo.php on line 8
Ideally, to fix this issue, the method DemoArray()
should return an array, but sometimes these situations can occur. It is better to handle these situations with a default value rather than fix the issue repeatedly.
Let’s try to handle this situation with a default value:
<?php
function DemoArray() {
return false;
}
$DemoArray = DemoArray();
if (is_array($DemoArray) || is_object($DemoArray)) {
foreach($DemoArray as $DemoValue){
echo $DemoValue;
}
}
// In case the above method is not working, we set a default value in the else, or we can perform any other operations.
else {
$DemoArray = array("Hello!", "This", "is", "delftstack.com");
foreach($DemoArray as $DemoValue){
echo $DemoValue."<br>";
}
}
?>
Now the above example is handled properly. So whenever the situation occurs for the foreach
loop, we should at least have a default value of the error statement.
See the output:
Hello!
This
is
delftstack.com
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook