How to Format a Number to a Dollar Amount in PHP
-
Format a Number to a Dollar Amount in PHP Using the
number_format
Function -
Format a Number to a Dollar Amount in PHP Using the
NumberFormatter::formatCurrency
Function -
Format a Number to a Dollar Amount in PHP Using a
Regular Expression
- Format a Number to a Dollar Amount in PHP Manually
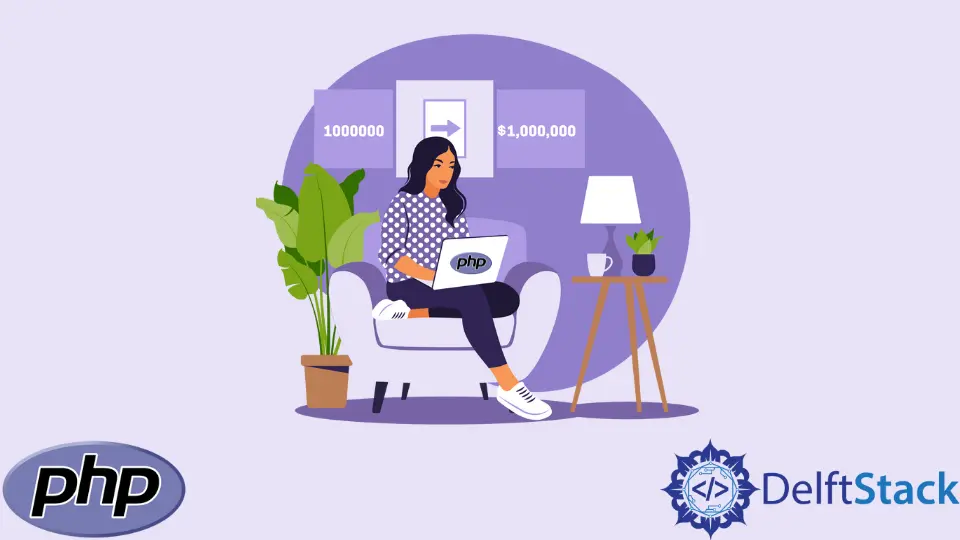
This tutorial article will cover different methods to format a number to a dollar amount in PHP with examples. These include:
number_format
NumberFormatter::formatCurrency
Regular expressions
Manual format
We will also look at why the money_format
function is no longer used.
Format a Number to a Dollar Amount in PHP Using the number_format
Function
We use the number_format
function to arrange a value with grouped thousands while adding decimal places and a currency type.
The function has four parameters:
number_format(NUMBER, DECIMAL DIGITS, THOUSANDS SEPARATOR, DECIMAL SEPARATOR)
- The number is the value to be formatted.
- Decimal digits specify how many decimal places.
- Decimal separator identifies what string to use for the decimal point.
- Thousand separator dictates the string used as a thousands separator.
It is worth noting if the thousand separator parameter is in use, the other three must accompany it for your code to work.
Example code:
<?php
// NUMBER
$amount = 123.45;
// TO USD - $123.45
$usd = "$" . number_format($amount, 2, ".");
echo $usd;
?>
Output:
$123.45
Format a Number to a Dollar Amount in PHP Using the NumberFormatter::formatCurrency
Function
This is the latest and arguably the easiest method to format numbers to strings showing different currencies.
Ensure the extension=intl
is enabled in php.ini
.
There are three parameters you should keep in mind:
- Formatter, which is the
NumberFormatter
object. - Amount, which is the numeric currency value.
- The ISO 4217 dictates the currency to use.
Example code:
<?php
// NUMBER
$amount = 123;
// TO USD - $123.00
$fmt = new NumberFormatter("en_US", NumberFormatter::CURRENCY);
$usd = $fmt->formatCurrency($amount, "USD");
echo $usd;
?>
Output:
$123.00
Example two:
<?php
// NUMBER
$amount = 123.456;
// TO USD - $123.46
$fmt = new NumberFormatter("en_US", NumberFormatter::CURRENCY);
$usd = $fmt->formatCurrency($amount, "USD");
echo $usd;
?>
Output:
$123.46
Format a Number to a Dollar Amount in PHP Using a Regular Expression
This method is a whole can of worms. Going into its details will only confuse you.
This method arranges numbers into thousands while adding the currency sign of your choice.
Let us take a look at an example:
<?php
// NUMBER
$amount = 0.13;
// REGULAR EXPRESSION
$regex = "/\B(?=(\d{3})+(?!\d))/i";
$usd = "$" . preg_replace($regex, ",", $amount);
echo $usd;
?>
Output:
$0.13
Format a Number to a Dollar Amount in PHP Manually
This method is equivalent to picking a lock with brutal force. This method gives you the advantage of using any format you want.
Let us look at an example:
<?php
// FOR A DOLLAR CURRENCY
function curformat ($amount) {
// SPLIT WHOLE & DECIMALS
$amount = explode(".", $amount);
$whole = $amount[0];
$decimal = isset($amount[1]) ? $amount[1] : "00" ;
// ADD THOUSAND SEPARATORS
if (strlen($whole) > 3) {
$temp = ""; $j = 0;
for ($i=strlen($whole)-1; $i>=0; $i--) {
$temp = $whole[$i] . $temp;
$j++;
if ($j%3==0 && $i!=0) { $temp = "," . $temp; }
}
$whole = $temp;
}
// RESULT
return "\$$whole.$decimal";
}
// UNIT TEST
echo curformat(100); // $100.00
Output:
$100.00
The above methods should format a number to a string showing dollars and cents.
There is another method called money_format
, but it does not work on windows. We highly advise you not to use this function as it is deprecated.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn