Constructor in PHP
- Use the PHP Constructor to Initialize the Properties of an Object in a Class
-
Use the PHP Constructor to Initialize the Properties of an
Object with Parameters
in a Class -
Initiate an Object in a Child Class and Call the Parent Class Constructor When Both Classes Have
Individual
Constructors in PHP
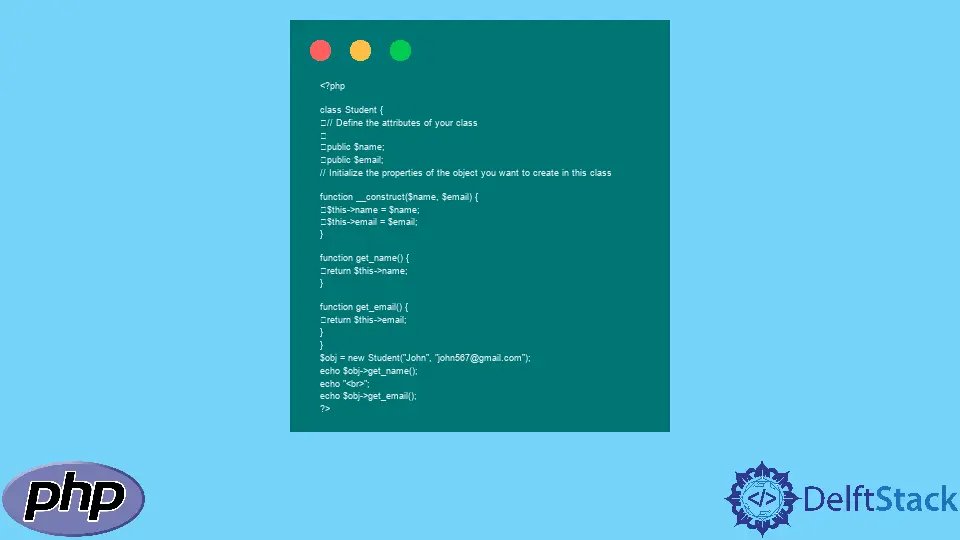
In this tutorial, we will introduce the PHP constructor. We will see how you can use the __construct()
function to initialize the properties of an instance in a class.
We will also use the function to initialize the properties of objects with given parameters in a class.
Last, we will see how you can initiate an object in a child class
and call the parent class
constructor when both classes have individual constructors.
Use the PHP Constructor to Initialize the Properties of an Object in a Class
In the example below, we will create a class Student
and use the __construct
function to assign new Student
its properties.
The __construct
function reduces the number of codes associated with using the function set_name()
.
<?php
class Student {
// Define the attributes of your class
public $name;
public $email;
// Initialize the properties of the object you want to create in this class
function __construct($name, $email) {
$this->name = $name;
$this->email = $email;
}
function get_name() {
return $this->name;
}
function get_email() {
return $this->email;
}
}
$obj = new Student("John", "john567@gmail.com");
echo $obj->get_name();
echo "<br>";
echo $obj->get_email();
?>
Output:
John
john567@gmail.com
Use the PHP Constructor to Initialize the Properties of an Object with Parameters
in a Class
In the example code below, we create the class Military
and use the __construct
function to give the properties and parameters of the objects we create.
<?php
class Military {
// Define the attributes of the class 'Military'
public $name;
public $rank;
function __construct($name, $rank){
$this->name = $name;
$this->rank = $rank;
}
function show_detail() {
echo $this->name." : ";
echo "Your Rank is ".$this->rank."\n";
}
}
$person_obj = new Military("Michael", "General");
$person_obj->show_detail();
echo "<br>";
$person2 = new Military("Fred", "Commander");
$person2->show_detail();
?>
Output:
Michael : Your Rank is General
Fred : Your Rank is Commander
Initiate an Object in a Child Class and Call the Parent Class Constructor When Both Classes Have Individual
Constructors in PHP
<?php
class Student
{
public $name;
public function __construct($name)
{
$this->name = $name;
}
}class Identity extends Student
{
public $identity_id;
public function __construct($name, $identity_id)
{
parent::__construct($name);
$this->identity_id = $identity_id;
}
function show_detail() {
echo $this->name." : ";
echo "Your Id Number is ".$this->identity_id."\n";
}
}
$obj = new Identity('Alice', '1036398');
echo $obj->show_detail();
?>
Output:
Alice : Your Id Number is 1036398
The class Identity
extends the class Student
in the code above. We use the keyword parent:
to call the constructor for the Student
class.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn