How to Calculate Absolute Value in NumPy
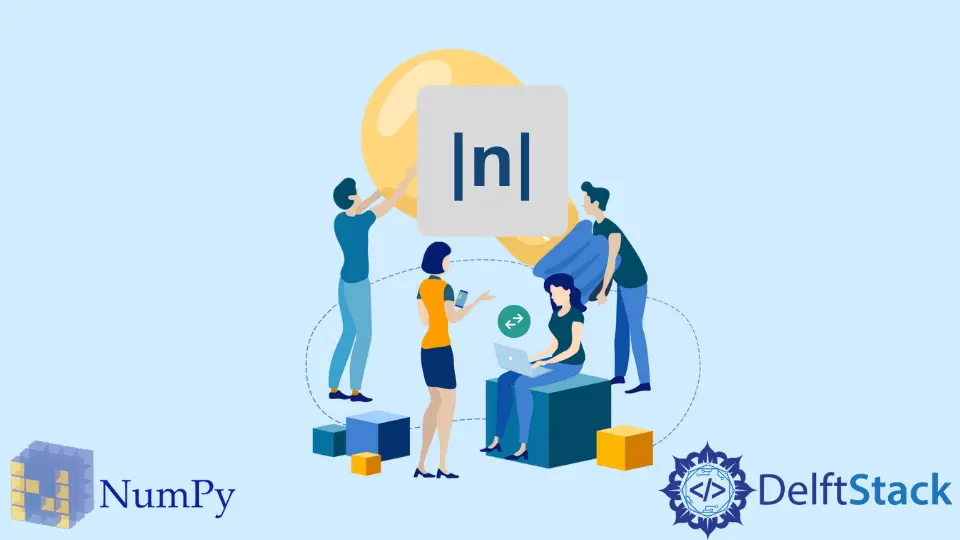
Calculating absolute values is a fundamental operation in data analysis and scientific computing. In Python, the NumPy library offers efficient ways to perform this operation on arrays and numerical data. Whether you’re dealing with a simple list of numbers or complex multi-dimensional arrays, understanding how to calculate absolute values can enhance your data manipulation skills.
In this article, we will explore three primary methods to calculate absolute values in NumPy: the abs()
function, numpy.absolute(), and numpy.abs(). Each method has its unique features and use cases, making it essential to know which to use in different scenarios. Let’s dive into each method and see how they work in practice.
Using the abs()
Function
The abs()
function is a built-in Python function that returns the absolute value of a number. While it is not specific to NumPy, it can be used effectively with NumPy arrays. When you apply the abs()
function to a NumPy array, it will return a new array containing the absolute values of the original array elements.
Here’s how you can use the abs()
function with NumPy:
import numpy as np
array = np.array([-1, -2, 3, -4])
absolute_values = abs(array)
print(absolute_values)
Output:
[1 2 3 4]
The abs()
function works seamlessly with NumPy arrays. When you pass an array to abs()
, it processes each element and returns a new array with the absolute values. This method is straightforward and effective for one-dimensional arrays. However, if you work with multi-dimensional arrays, you might want to consider using NumPy’s specific functions for better performance and clarity. The abs() function is easy to remember and use, making it a good choice for quick calculations.
Using numpy.absolute()
The numpy.absolute() function is specifically designed for NumPy arrays and is a more explicit way to calculate absolute values. It behaves similarly to the built-in abs()
function but is optimized for use with NumPy data structures. This function can handle arrays of any shape and size, making it versatile for various applications.
Here’s an example of how to use numpy.absolute():
import numpy as np
array = np.array([[-1, -2, 3], [-4, 5, -6]])
absolute_values = np.absolute(array)
print(absolute_values)
Output:
[[1 2 3]
[4 5 6]]
By using numpy.absolute(), you ensure that your code is clear about its intent, which can be helpful for readability. This function also supports complex numbers, returning the magnitude of each complex element. As a result, it’s a powerful option for scientific computations where complex numbers are common. The ability to work with multi-dimensional arrays without additional effort makes numpy.absolute() a preferred choice for many data scientists and engineers.
Using numpy.abs()
The numpy.abs() function is yet another way to calculate absolute values in NumPy, and it is essentially an alias for numpy.absolute(). This means that both functions perform the same operation and can be used interchangeably. However, using numpy.abs() can sometimes make the code more concise and easier to read, especially for those familiar with NumPy.
Here’s how to use numpy.abs():
import numpy as np
array = np.array([[1, -2], [-3, 4]])
absolute_values = np.abs(array)
print(absolute_values)
Output:
[[1 2]
[3 4]]
The numpy.abs() function is optimized for performance and is capable of handling arrays of any dimension. Just like numpy.absolute(), it can also process complex numbers. The choice between numpy.abs() and numpy.absolute() often comes down to personal preference or coding style. In practice, both functions will give you the same results, so you can use whichever you find more intuitive.
Conclusion
Calculating absolute values in NumPy is a straightforward process, thanks to the various methods available. Whether you choose the built-in abs() function, numpy.absolute(), or numpy.abs(), each method has its unique strengths and can be used effectively depending on your specific needs. Understanding these functions will not only enhance your coding skills but also improve your efficiency when working with numerical data. As you continue to explore the capabilities of NumPy, these methods will become essential tools in your data analysis toolkit.
FAQ
- What is the difference between abs() and numpy.absolute()?
abs() is a built-in Python function that can be used with NumPy arrays, while numpy.absolute() is specifically designed for NumPy arrays and provides better clarity in code.
-
Can I use numpy.abs() with complex numbers?
Yes, numpy.abs() can handle complex numbers and will return their magnitudes. -
Are there performance differences between these methods?
Generally, numpy.absolute() and numpy.abs() are optimized for performance with NumPy arrays, while abs() may not be as efficient for large datasets. -
Which method should I use for multi-dimensional arrays?
It is recommended to use numpy.absolute() or numpy.abs() for multi-dimensional arrays as they are specifically designed for that purpose. -
Is there any difference in output when using these functions?
No, all three methods will yield the same output when calculating absolute values.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn