How to Convert NumPy Array to Tuple
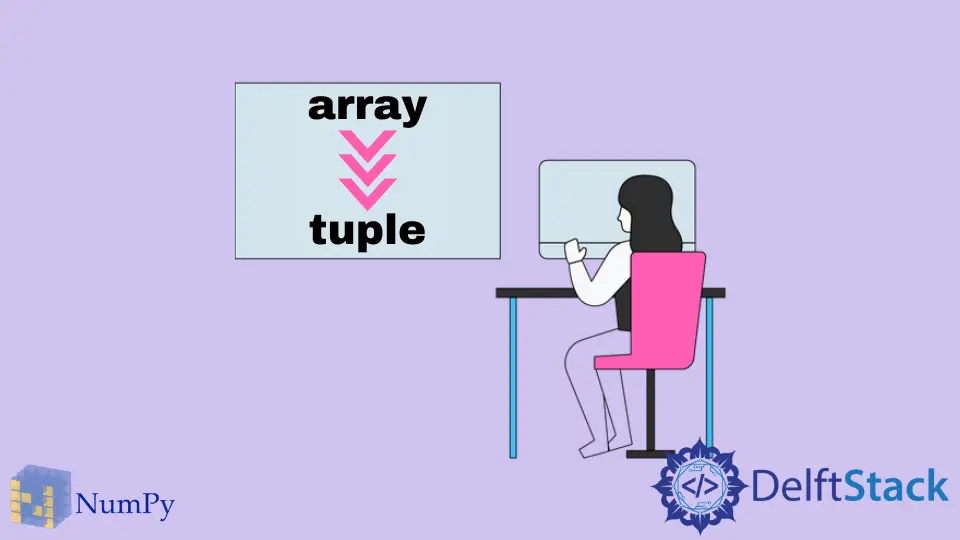
When working with data in Python, NumPy arrays are a popular choice due to their efficiency and versatility. However, there are times when you might need to convert these arrays into tuples. This can be particularly useful when you want to pass data to functions that require tuples or when you need to ensure immutability.
In this article, we will explore two primary methods for converting a NumPy array to a tuple: using the built-in tuple()
function and leveraging the map()
function. Whether you are a beginner or an experienced Python developer, this guide will provide you with clear examples and explanations to help you master this conversion.
Method 1: Using the tuple()
Function
The simplest way to convert a NumPy array to a tuple is by using the built-in tuple()
function. This function takes an iterable as an argument and returns a tuple containing the elements of that iterable. In the case of a NumPy array, you can directly pass the array to the tuple()
function.
Here’s how you can do it:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
tuple_result = tuple(array)
print(tuple_result)
Output:
(1, 2, 3, 4, 5)
Using the tuple()
function is straightforward. When you call tuple(array)
, the function iterates over the elements of the NumPy array and constructs a tuple from them. This method is ideal for simple conversions where you want to quickly transform an array into a tuple. It’s efficient and easy to understand, making it a go-to choice for many developers.
One thing to note is that the resulting tuple will contain the same elements as the original NumPy array. However, it will not maintain any array-specific properties, such as shape or dimensionality. If you need to work with multi-dimensional arrays, you might want to consider flattening the array before conversion, depending on your specific requirements.
Method 2: Using the map()
Function
Another effective way to convert a NumPy array to a tuple is by using the map()
function in combination with the tuple()
function. The map()
function applies a specified function to every item in an iterable, which can be useful for more complex transformations. In this case, we can use map()
to convert each element of the NumPy array to a desired type before creating a tuple.
Here’s how this can be accomplished:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
tuple_result = tuple(map(int, array))
print(tuple_result)
Output:
(1, 2, 3, 4, 5)
In this example, we use map(int, array)
to ensure that each element of the NumPy array is converted to an integer before being passed to the tuple()
function. While this may seem redundant since the original array contains integers, it demonstrates how you can use map()
for more complex data types or transformations.
This method is particularly useful when dealing with arrays of floats or strings, where you might want to convert them into integers or another type before creating the tuple. The flexibility of map()
allows you to customize the conversion process according to your needs.
Conclusion
Converting a NumPy array to a tuple is a straightforward task that can be accomplished using either the tuple()
function or the map()
function. Each method has its advantages, depending on the complexity of the data you are working with. Using tuple()
is quick and easy for simple arrays, while map()
provides more flexibility for data transformation. By mastering these techniques, you’ll be better equipped to handle data manipulation tasks in Python, enhancing your programming toolkit.
FAQ
-
How do I convert a multi-dimensional NumPy array to a tuple?
You can flatten the array usingarray.flatten()
orarray.ravel()
and then use thetuple()
function to convert it. -
Can I convert a NumPy array of strings to a tuple?
Yes, you can use thetuple()
function directly, as it works with any iterable, including arrays of strings. -
Is there a performance difference between using tuple() and map()?
For simple conversions,tuple()
is typically faster. Usemap()
when you need to apply transformations to the data. -
What happens to the shape of the array when converted to a tuple?
The shape of the array is not preserved in the tuple; it becomes a flat sequence of elements. -
Can I convert a NumPy array with complex numbers to a tuple?
Yes, you can convert arrays with complex numbers to tuples using thetuple()
function without any issues.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn