Array of Arrays in NumPy
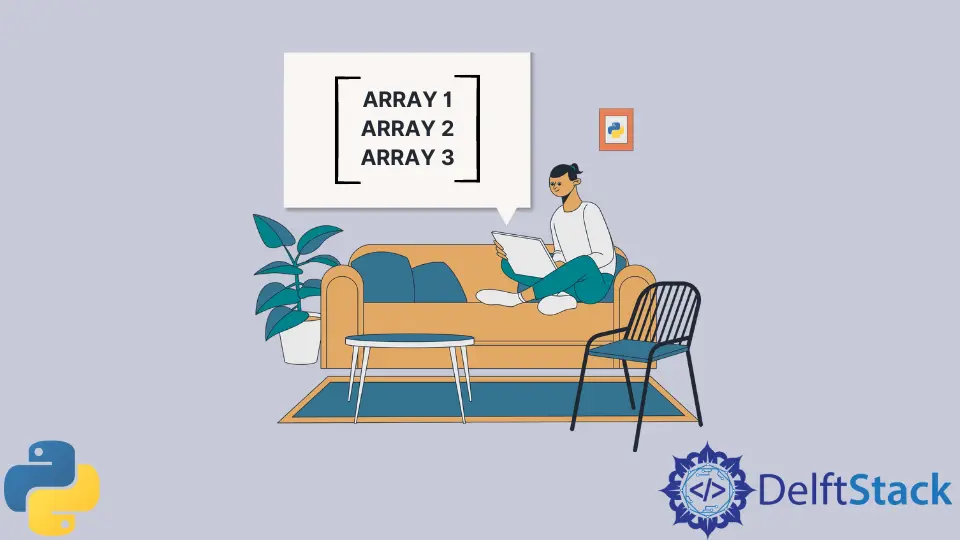
In this tutorial, we will discuss the method to create an array of arrays in Python.
Create Array of Arrays With the numpy.array()
Function in Python
By default, the Python programming language has no support for the arrays. This shortcoming can be solved with the NumPy
package for Python. The NumPy
package does not come pre-installed in Python. So, we have to install the NumPy
package for working with arrays in Python. The numpy.array()
function inside the NumPy
package is used to create an array in Python. We pass a sequence of elements enclosed in a pair of square brackets to the numpy.array()
function, and it returns an array containing the exact sequence of elements. The array of arrays, or known as the multidimensional array, can be created by passing arrays in the numpy.array()
function. The following code example shows us how to create an array of arrays or a multidimensional array with the numpy.array()
function in Python.
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
array3 = np.array([7, 8, 9])
arrayOfArrays = np.array([array1, array2, array3])
print(arrayOfArrays)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
In the above code, we created the array of arrays arrayOfArrays
that contains array1
, array2
, and array3
with the np.array()
function in Python. We first initialized three arrays array1
, array2
, and array3
with the np.array()
function. We then created another array arrayOfArrays
by passing all the previously initialized arrays to the np.array()
function. In the end, we displayed the values inside the arrayOfArrays
with the print()
function in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn