How to Convert PIL Image to NumPy Array
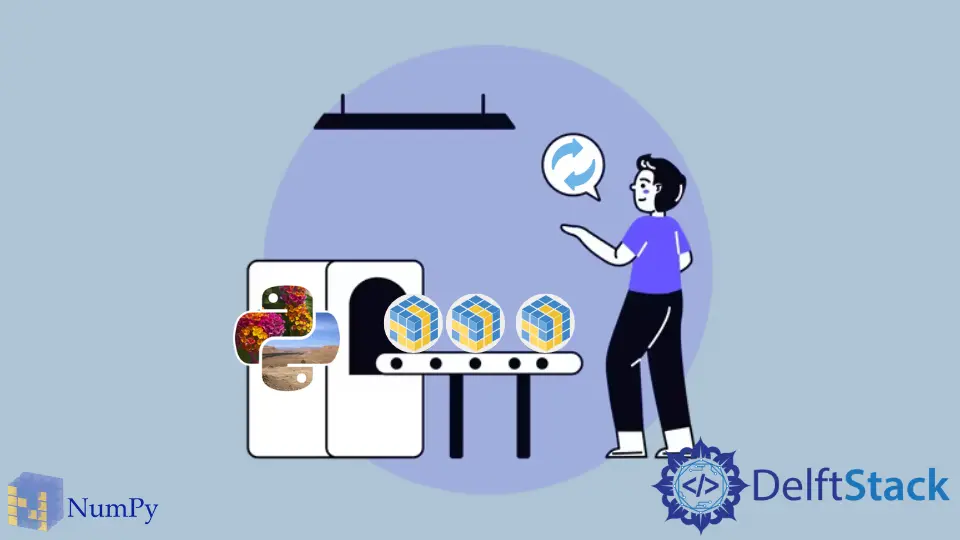
When working with images in Python, the Python Imaging Library (PIL) and NumPy are two powerful tools that often go hand in hand. Converting a PIL image to a NumPy array can be essential for various image processing tasks, machine learning applications, or simply for data manipulation. Fortunately, this conversion is straightforward and can be accomplished using two primary methods: the numpy.array()
function and the numpy.asarray()
function.
In this article, we will explore both methods in detail, providing clear code examples and explanations to help you understand how to make this conversion seamlessly. Whether you’re a beginner or an experienced developer, you’ll find the information here useful for your projects involving image processing.
Method 1: Using numpy.array()
The first method to convert a PIL image to a NumPy array is by using the numpy.array()
function. This function creates a new array from the given image, which is particularly useful when you want to ensure that the data is independent of the original image object. Here’s how you can do it:
from PIL import Image
import numpy as np
# Open an image file
image = Image.open('example_image.jpg')
# Convert the image to a NumPy array
image_array = np.array(image)
print(image_array.shape)
Output:
(height, width, channels)
In this code snippet, we first import the necessary libraries: Image
from PIL and numpy
as np
. After opening the image file using Image.open()
, we convert it to a NumPy array by passing the image object to np.array()
. The resulting image_array
is a 3-dimensional array where the first dimension corresponds to the height, the second to the width, and the third to the color channels (e.g., RGB). This method is particularly beneficial when you need a fresh copy of the image data, as it creates a new array, ensuring that any modifications you make to the NumPy array do not affect the original image.
Method 2: Using numpy.asarray()
The second method involves using the numpy.asarray()
function to convert a PIL image to a NumPy array. This function is slightly different from numpy.array()
in that it does not create a new copy of the data if the input is already an array. This can save memory and improve performance in certain situations. Let’s look at how this works:
from PIL import Image
import numpy as np
# Open an image file
image = Image.open('example_image.jpg')
# Convert the image to a NumPy array
image_array = np.asarray(image)
print(image_array.shape)
Output:
(height, width, channels)
In this example, we follow a similar process as before. After importing the necessary libraries and opening the image, we convert it to a NumPy array using np.asarray()
. The output will also display the shape of the array, which again indicates the height, width, and color channels. The key difference with asarray()
is that it will return a view of the original data if possible, making it more memory-efficient. This method is ideal when you are confident that you won’t need to modify the original image and want to work with the data as is.
Conclusion
Converting a PIL image to a NumPy array is a straightforward process that can significantly enhance your image processing capabilities in Python. Whether you choose to use numpy.array()
for a fresh copy of the data or numpy.asarray()
for a more memory-efficient approach, both methods are effective and easy to implement. Understanding these conversions can open up a world of possibilities for image analysis, machine learning, and data manipulation. So, the next time you’re working with images in Python, remember these techniques to streamline your workflow.
FAQ
-
What is the difference between numpy.array() and numpy.asarray()?
numpy.array() creates a new array, while numpy.asarray() returns a view of the original data if possible, making it more memory efficient. -
Can I convert images in formats other than JPEG?
Yes, you can convert images in various formats like PNG, BMP, and GIF using the same methods. -
Do I need to install additional libraries to use PIL and NumPy?
Yes, you need to install Pillow for PIL functionality and NumPy for numerical operations. You can install them using pip. -
How can I visualize the NumPy array after conversion?
You can use libraries like Matplotlib to visualize the NumPy array by displaying it as an image. -
Is it possible to convert a NumPy array back to a PIL image?
Yes, you can convert a NumPy array back to a PIL image using the Image.fromarray() function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn